141. Linked List Cycle*
https://leetcode.com/problems/linked-list-cycle/
题目描述
Given a linked list, determine if it has a cycle in it.
To represent a cycle in the given linked list, we use an integer pos
which represents the position (0-indexed) in the linked list where tail connects to. If pos
is -1
, then there is no cycle in the linked list.
Example 1:
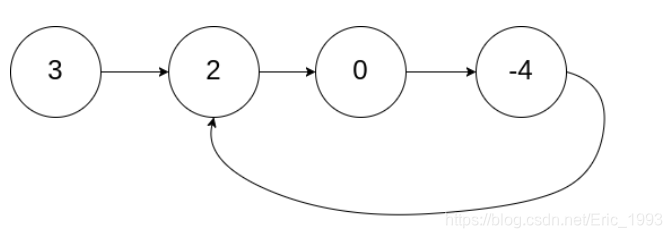
Input: head = [3,2,0,-4], pos = 1
Output: true
Explanation: There is a cycle in the linked list, where tail connects to the second node.
Example 2:
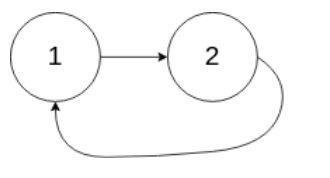
Input: head = [1,2], pos = 0
Output: true
Explanation: There is a cycle in the linked list, where tail connects to the first node.
Example 3:
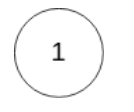
Input: head = [1], pos = -1
Output: false
Explanation: There is no cycle in the linked list.
Follow up:
Can you solve it using (i.e. constant) memory?
C++ 实现 1
使用快慢指针来判断是否有环. 第一种写法是初始化时, 快慢指针不指向同一个节点.
另外, 142. Linked List Cycle II** 这题要求找到环的起始节点.
class Solution {
public:
bool hasCycle(ListNode *head) {
if (!head || !head->next) return false;
ListNode *slow = head, *fast = head->next;
while (fast && fast->next && slow != fast) {
slow = slow->next;
fast = fast->next->next;
}
return slow == fast;
}
};
C++ 实现 2
这种写法是快慢指针初始时指向相同的节点.
class Solution {
public:
bool hasCycle(ListNode *head) {
if (!head || !head->next)
return false;
ListNode *slow = head, *fast = head;
while (fast && fast->next) {
slow = slow->next;
fast = fast->next->next;
if (fast == slow)
return true;
}
return false;
}
};