一、所有类型的指针长度都是4个字节
*有两个含义:
1.定义时表示后面的变量是一个指针
2.使用时表示取值
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main()
{
#if 0
int *p; //定义一个指针
printf("%p\n", p);
*p = 1; //不合法 不能直接使用
int a = 1;
int *q;
q = &a; //给指针赋值
int *pa;
pa = q; //给pa赋值q
#endif
char *fp;
fp = (char *)malloc(sizeof(char) * 20); //向操作系统申请空间 连续的空间 堆空间
if (NULL == fp)
{
printf("malloc falure!\n");
}
strcpy(fp, "helloworld");
printf("%s\n", fp);
free(fp); //释放空间
return 0;
}
二、指针变量的使用步骤
1、定义一个指针
2、给指针赋值
3、正常使用指针进行运算
三、字符串逆序
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void string_reverse(const char *ptr, char *str)
{
int len = strlen(ptr), i;
ptr += (len - 1);
for (i = 0; i < len; i++)
{
*str = *ptr;
str++;
ptr--;
}
}
int main()
{
char *ptr = "helloworld!"; //ptr指向helloworld字符串 helloworld是常量,不能修改
char *str = (char *)malloc(sizeof(char) * 64);
string_reverse(ptr, str);
printf("%s\n", str); //打印字符串格式 %s, 参数是字符串的首地址
return 0;
}
四、字符串找子串
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int len_str, len_ptr, i;
char *str = (char *)malloc(sizeof(char) * 32);
char *ptr = (char *)malloc(sizeof(char) * 32);
printf("Please input :\n");
scanf("%s%s", str, ptr);
len_str = strlen(str);
len_ptr = strlen(ptr);
if (len_str < len_ptr)
{
printf("input error!\n");
return -1;
}
for (i = 0; i < len_str - len_ptr + 1; i++)
{
if (strncmp(str + i, ptr, len_ptr) == 0)
{
printf("%s 是 %s 的子串\n", ptr, str);
break;
}
if (i == len_str - len_ptr)
{
printf("%s 不是 %s 的子串\n", ptr, str);
}
}
return 0;
}
扫描二维码关注公众号,回复:
2437007 查看本文章
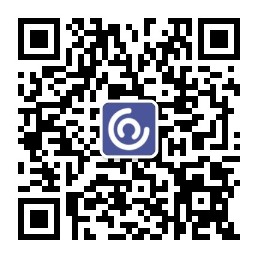