Leetcode 104题 Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.
The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Note: A leaf is a node with no children.
Example:
Given binary tree [3,9,20,null,null,15,7]
,
3
/ \
9 20
/ \
15 7
return its depth = 3.
题目大意: 求一个树的最大深度。 最大深度 = 根节点至最深的距离。
两个解法: 两个思路 DFS和BFS。
DFS很简单,直接上代码。
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def maxDepth(self, root: TreeNode) -> int:
if not root:
return 0
return 1 + max(self.maxDepth(root.left),self.maxDepth(root.right))
#DFS
self.maxDepth自己返回左右最深的数值。 取最大值再加一即可。
BFS情况
使用队列。
扫描二维码关注公众号,回复:
10326614 查看本文章
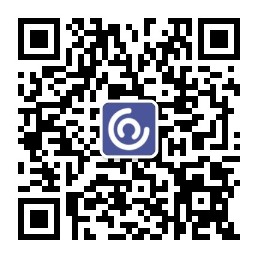
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def maxDepth(self, root: TreeNode) -> int:
if not root:
return 0
depth = 0
que = [root] #定义队列que是root的集合。
while que: #当que存在时。
depth += 1
for i in range(0, len(que)):
if que[0].left: #队列0节点的左子节点存在时:
que.append(que[0].left) #队列加上左子节点
if que[0].right: #同理
que.append(que[0].right) #同理
del que[0] #删除que[0]循环往复。que[0]永远在更新。
return depth
#BFS
最后这个del que[0] 使用的不是很熟练。学会了。
2020/03/10
英国,晚上,雨。
加油!