文章目录
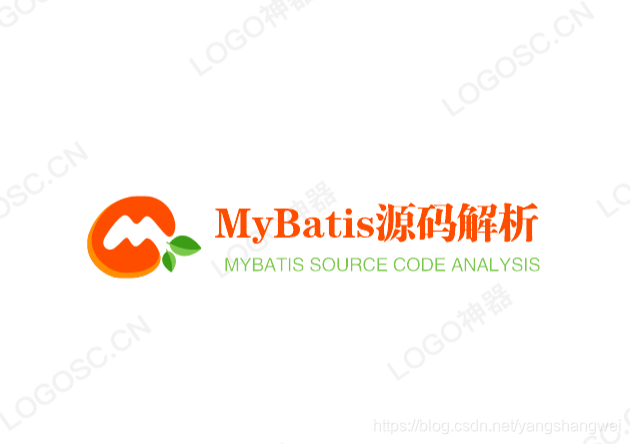
Pre
如果MyBatis的基础用法还不熟悉,31篇入门博客拿走不谢
戳戳戳 —> https://blog.csdn.net/yangshangwei/category_7205317.html
工程概览
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.artisan</groupId>
<artifactId>mybatisSource</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.17</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.1</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
<resource>
<directory>src/test/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
</build>
</project>
mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="app.properties">
</properties>
<settings>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
<typeAliases>
</typeAliases>
<environments default="dev">
<environment id="dev">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${jdbc.driver}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<package name="com.artisan"/>
</mappers>
</configuration>
UserMapper
package com.artisan;
import com.artisan.bean.User;
import org.apache.ibatis.annotations.Select;
public interface UserMapper {
@Select({"select * from users where id = #{id}"})
User selectByid(Integer id);
}
测试类
基类
import org.apache.ibatis.session.Configuration;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Before;
/**
* 基类
*/
public class BaseTest {
public SqlSessionFactory factory;
public Configuration configuration;
@Before
public void inin(){
// 获取构建器
SqlSessionFactoryBuilder factoryBuilder = new SqlSessionFactoryBuilder();
// 解析xml ,构建会话工厂
factory = factoryBuilder.build(SqlSessionTest.class.getResourceAsStream("/mybatis-config.xml"));
// 获取 configuration 这个大管家
configuration = factory.getConfiguration();
}
}
SqlSessionTest 测试类
package com.artisan;
import com.artisan.bean.User;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.session.ExecutorType;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
import java.util.List;
public class SqlSessionTest extends BaseTest{
private MappedStatement ms;
@Test
public void sqlSessionTest(){
// 获取statement SQL映射
ms = configuration.getMappedStatement("com.artisan.UserMapper.selectByid");
// 开启会话 第一个参数 执行器类型 第二个参数 是否自动提交
SqlSession sqlSession = factory.openSession(ExecutorType.SIMPLE, true);
// 门面模式 屏蔽了底层调用的复杂用 统一对接sqlSession
List<User> users = sqlSession.selectList(ms.getId(), 1);
System.out.println(users.get(0));
}
}
测试结果:
selectList 源码解析
附
SQL
/*
Navicat MySQL Data Transfer
Source Server : localhost_root
Source Server Version : 80011
Source Host : 127.0.0.1:3306
Source Database : artisan
Target Server Type : MYSQL
Target Server Version : 80011
File Encoding : 65001
Date: 2020-06-14 00:46:05
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `users`
-- ----------------------------
DROP TABLE IF EXISTS `users`;
CREATE TABLE `users` (
`id` int(11) NOT NULL,
`name` varchar(255) DEFAULT NULL,
`age` varchar(255) DEFAULT NULL,
`sex` varchar(255) DEFAULT NULL,
`email` varchar(255) DEFAULT NULL,
`phoneNumber` varchar(255) DEFAULT NULL,
`createTime` date DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of users
-- ----------------------------
INSERT INTO `users` VALUES ('1', 'artisan', '11', 'male', '[email protected]', '12345', '2020-06-04');
log4j.properties
### set log levels ###
log4j.rootLogger = DEBUG ,stdout
###
log4j.appender.stdout = org.apache.log4j.ConsoleAppender
log4j.appender.stdout.Target = System.out
log4j.appender.stdout.layout = org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern = %d{ABSOLUTE} %5p %c:%L - %m%n
app.properties
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://127.0.0.1:3306/artisan?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT
jdbc.username=root
jdbc.password=root
default.environment=dev
User
package com.artisan.bean;
import java.util.Date;
public class User implements java.io.Serializable {
private Integer id;
private String name;
private String age;
private String sex;
private String email;
private String phoneNumber;
private Date createTime;
public User() {
}
public User(Integer id, String name) {
this.id = id;
this.name = name;
}
....省略 set/get
....
}