手动配置数据源
① 不使用数据源
@Test
public void test0() throws Exception {
// 无非四个参数———驱动、数据库url、用户名、用户密码
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/loliDB", "root", "123456");
connection.close();
}
② 手动导入c3p0数据源
@Test
public void test1() throws Exception {
// 创建数据源
ComboPooledDataSource dataSource = new ComboPooledDataSource();
// 配置数据源
dataSource.setDriverClass("com.mysql.cj.jdbc.Driver");
dataSource.setJdbcUrl("jdbc:mysql://localhost:3306/loliDB");
dataSource.setUser("root");
dataSource.setPassword("123456");
// 获取连接
Connection connection = dataSource.getConnection();
// 归还连接
connection.close();
}
③ 手动导入c3p0数据源(加载properties配置文件)
(resource下的jdbc.properties资源文件)
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/loliDB
jdbc.username=root
jdbc.password=123456
@Test
public void test2() throws Exception {
// 读取配置文件
ResourceBundle rb = ResourceBundle.getBundle("jdbc"); // 传入"基名"
String driver = rb.getString("jdbc.driver");
String url = rb.getString("jdbc.url");
String username = rb.getString("jdbc.username");
String password = rb.getString("jdbc.password");
// 创建数据源
ComboPooledDataSource dataSource = new ComboPooledDataSource();
// 配置数据源
dataSource.setDriverClass(driver);
dataSource.setJdbcUrl(url);
dataSource.setUser(username);
dataSource.setPassword(password);
// 获取连接
Connection connection = dataSource.getConnection();
// 归还连接
connection.close();
}
Spring配置数据源
将参数直接注入
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.cj.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/loliDB"></property>
<property name="user" value="root"></property>
<property name="password" value="20006212000d"></property>
</bean>
测试一下吧
@Test
// Spring配置数据源
public void test3() throws Exception {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
ComboPooledDataSource dataSource = (ComboPooledDataSource) app.getBean("dataSource");
Connection connection = dataSource.getConnection();
connection.close();
}
实际的开发场景中,我们还需要使用properties资源文件进行进一步解耦——即在applicationContext.xml配置文件中引入jdbc.properties资源文件。
实现步骤为
- 在applicationContext中引入context命名空间
xmlns:context="http://www.springframework.org/schema/context"
- 在applicationContext中引入context约束路径
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
- 加载资源文件
<context:property-placeholder location="classpath:xxx.properties" />
- 使用SPEL语法进行注入
"${xxx}"
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.cj.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/loliDB"></property>
<property name="user" value="root"></property>
<property name="password" value="123456"></property>
</bean>
</beans>
修改为:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 加载外部的properties资源文件 -->
<context:property-placeholder location="classpath:jdbc.properties" />
<!-- 写死的地方修改为SPEL语法 -->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driver}"></property>
<property name="jdbcUrl" value="${jdbc.url}"></property>
<property name="user" value="${jdbc.username}"></property>
<property name="password" value="${jdbc.password}"></property>
</bean>
</beans>
补充
不难发现,在经典的手动数据源配置时,使用了许多setXxx
格式的API —— 这不正是给spring的set依赖注入所准备的吗?
扫描二维码关注公众号,回复:
12934466 查看本文章
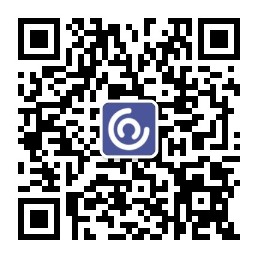
另外的一个细节是,c3p0连接池(ComboPooledDataSource
)和Druid连接池(DruidDataSource
)的set方法,或者说是数据源配置参数的名称,是有些许区别的:setDriverClass/setDriverClassName
,setJdbcUrl/setUrl
,setUser/setUsername
,setPassword/setPassword
。