文章目录
简介
uplink是访问storj网络的工具(客户端),主要有三类:1、libuplink 各类语言的类库 2、uplink cli命令行工具 3、gateway 。uplink cli可以直接和satellite交互也可以和gateway交互,由于gateway兼容s3,因此可以使用aws s3 cli与 gateway交互,甚至可以使用s3类库与gateway通信,如果你原来使用的是S3对象存储,可以直接用storj替换。gateway可以看成是一种特殊的uplink
uplink lib
- go: https://github.com/storj/uplink
- c: https://github.com/storj/uplink-c
- java: https://github.com/storj/uplink-java
- android: https://github.com/storj/uplink-android
- chrome(webassembly): https://github.com/storj/uplink-chrome
uplink go
需要的参数:
- APIKey = “change-me-to-the-api-key-created-in-satellite-gui”
- satellite = “us-central-1.tardigrade.io:7777”
// Copyright (C) 2019 Storj Labs, Inc.
// See LICENSE for copying information.
package main
import (
"bytes"
"context"
"fmt"
"io"
"io/ioutil"
"log"
"storj.io/uplink"
)
const (
myAPIKey = "change-me-to-the-api-key-created-in-satellite-gui"
satellite = "us-central-1.tardigrade.io:7777"
myBucket = "my-first-bucket"
myUploadKey = "foo/bar/baz"
myData = "one fish two fish red fish blue fish"
myPassphrase = "you'll never guess this"
)
// UploadAndDownloadData uploads the specified data to the specified key in the
// specified bucket, using the specified Satellite, API key, and passphrase.
func UploadAndDownloadData(ctx context.Context,
satelliteAddress, apiKey, passphrase, bucketName, uploadKey string,
dataToUpload []byte) error {
// Request access grant to the satellite with the API key and passphrase.
access, err := uplink.RequestAccessWithPassphrase(ctx, satelliteAddress, apiKey, passphrase)
if err != nil {
return fmt.Errorf("could not request access grant: %v", err)
}
// Open up the Project we will be working with.
project, err := uplink.OpenProject(ctx, access)
if err != nil {
return fmt.Errorf("could not open project: %v", err)
}
defer project.Close()
// Ensure the desired Bucket within the Project is created.
_, err = project.EnsureBucket(ctx, bucketName)
if err != nil {
return fmt.Errorf("could not ensure bucket: %v", err)
}
// Intitiate the upload of our Object to the specified bucket and key.
upload, err := project.UploadObject(ctx, bucketName, uploadKey, nil)
if err != nil {
return fmt.Errorf("could not initiate upload: %v", err)
}
// Copy the data to the upload.
buf := bytes.NewBuffer(dataToUpload)
_, err = io.Copy(upload, buf)
if err != nil {
_ = upload.Abort()
return fmt.Errorf("could not upload data: %v", err)
}
// Commit the uploaded object.
err = upload.Commit()
if err != nil {
return fmt.Errorf("could not commit uploaded object: %v", err)
}
// Initiate a download of the same object again
download, err := project.DownloadObject(ctx, bucketName, uploadKey, nil)
if err != nil {
return fmt.Errorf("could not open object: %v", err)
}
defer download.Close()
// Read everything from the download stream
receivedContents, err := ioutil.ReadAll(download)
if err != nil {
return fmt.Errorf("could not read data: %v", err)
}
// Check that the downloaded data is the same as the uploaded data.
if !bytes.Equal(receivedContents, dataToUpload) {
return fmt.Errorf("got different object back: %q != %q", dataToUpload, receivedContents)
}
return nil
}
func main() {
err := UploadAndDownloadData(context.Background(),
satellite, myAPIKey, myPassphrase, myBucket, myUploadKey, []byte(myData))
if err != nil {
log.Fatalln("error:", err)
}
fmt.Println("success!")
}
uplink cli
代码:https://github.com/storj/storj/tree/main/cmd/uplink
使用说明:https://github.com/storj/storj/wiki/Uplink-CLI
命令:https://documentation.tardigrade.io/api-reference/uplink-cli
uplink配置及使用(和satellite通信)
如果是tardigrade提供的网络则选择 1、2、3,如果是私有测试网络,则需要取satellite 控制台创建grant access(类型选continue in cli),记录下Satellite Address和Token,我们在执行uplink setup时需要填写.
uplink setup
Select your satellite:
[1] us-central-1.tardigrade.io
[2] europe-west-1.tardigrade.io
[3] asia-east-1.tardigrade.io
Enter number or satellite address as "<nodeid>@<address>:<port>" [1]: [email protected]:10000
Choose an access name (use lowercase letters) ["default"]:
Enter your API key: 1FkaZdRiVFZwRPYkoecWGAqNZdPFymWxr7n9AYpDRirehEhEQ23B7zqxuS2j7xixk7UVhjuU6h1bDagg7iVrrsCWe9Ptp6H9PfyAk4iyBJmvmepJno2PBZP7yt5826g3m6TpGdLLYb1nU5GJBnxT6
Data is encrypted on the network, with an encryption passphrase
stored on your local machine. Enter a passphrase you'd like to use.
## 输入两次加密密码
Enter your encryption passphrase:
Enter your encryption passphrase again:
With your permission, Tardigrade can automatically collect analytics information from your uplink CLI and send it to Storj Labs (makers of Tardigrade) to help improve the quality and performance of our products. This information is sent only with your consent and is submitted anonymously to Storj Labs: (y/n)
y
Your Uplink CLI is configured and ready to use!
* See https://documentation.tardigrade.io/api-reference/uplink-cli for some example commands
你的api key加密后a写入到~/.local/share/storj/uplink/config.yaml,如accesses.XXX,XXX是我们执行uplink setup配置的access name,没填 默认就是default.可以使用uplink setup配置多个卫星,如果需要连接到其他卫星则需要修改config.yaml的access指向
cat /root/.local/share/storj/uplink/config.yaml
# the serialized access, or name of the access to use
access: default
accesses.default: 1AYTtHBwWpNaCRpWrz2aAdEpDhNNM8sjwR8ePqajkBUstgviLyBrHqXfW9oQGdoAmiyBB6U3nYrgCJnjh6icoHXQtyJrtu2qUPNFq7C2bY1pWCbmuup7Ba7M8o7ZURB7NsYSULnuyUTFcB9iLk26cZnUhNacrEsyarxpjE84mVNCp3VbiSGTUwjQi11QzbRsCxoziZiF5oSKFBDgKYQVXXPahHHPoLbKytRVjuka86Fe4ui3R8RvHvJDTmXkKKCDWw53tC1FozP9eo5q9F3mYhvYZHRfHdadgJaC1oJSK
可以通过命令查看有哪些access,并查看access详细信息
$ uplink access list
=========== ACCESSES LIST: name / satellite ================================
default / [email protected]:10000
s2 / [email protected]:10010
$ uplink access inspect default
{
"satellite_addr": "[email protected]:10000",
"encryption_access": {
"default_key": "d1HKtRzdk3m6Er3dZMufQupjIh3zdM0m/UKMaUTuCTM=",
"default_path_cipher": "ENC_AESGCM"
},
"api_key": "1FkaZdRiVFZwRPYkoecWGAqNZdPFymWxr7n9AYpDRirehEhEQ23B7zqxuS2j7xixk7UVhjuU6h1bDagg7iVrrsCWe9Ptp6H9PfyAk4iyBJmvmepJno2PBZP7yt5826g3m6TpGdLLYb1nU5GJBnxT6",
"macaroon": {
"head": "mtBldtl7l391b-JYDwuOlL4jJAaFyO8ocI8_f73ksrk=",
"caveats": [
{
"not_after": "2200-01-01T00:00:00Z",
"not_before": "2021-03-31T00:56:55.462Z",
"nonce": "7fRCAQ=="
}
],
"tail": "MKvEppE6u4SznF9us_FW6QWxj1yl6HBeQ0Vypj_UnEo="
}
}
mb
$uplink mb sj://test6
Bucket test6 created
put
$echo "Very secret ingredient list" |uplink put sj://test6/secret.txt
Created sj://test6/secret.txt
ls
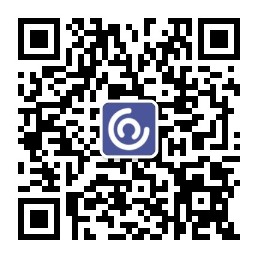
$uplink ls
BKT 2021-03-31 15:30:20 test5
BKT 2021-03-31 15:57:14 test6
$uplink ls sj:/test6
OBJ 2021-03-31 15:58:05 28 secret.txt
cat
$uplink cat sj://test6/secret.txt
Very secret ingredient list
uplink配置及使用(和gateway通信)
直接通过–config-dir指定网关配置目录即可
命令测试 /mb/put/cat/ls
创建一个桶
$ uplink --config-dir /data/storj-test/config/gateway/0/ mb sj://one
往桶里put一个key
$ echo "Very secret ingredient list" |uplink --config-dir /data/storj-test/config/gateway/0/ put sj://one/secret.txt
查看内容
$ uplink --config-dir /data/storj-test/config/gateway/0/ cat sj://one/secret.txt
Very secret ingredient list
rest.log 为当前目录的文件,将它复制到storj,key值默认为文件名
$uplink --config-dir /data/storj-test/config/gateway/0/ cp rest.log sj://one
查看桶内容
uplink --config-dir /data/storj-test/config/gateway/0/ ls sj://one/
OBJ 2021-03-30 17:36:35 28 secret.txt
OBJ 2021-03-30 17:46:27 1226171 rest.log
共享文件
uplink --config-dir /data/storj-test/config/gateway/0/ share --url sj://one/secret.txt
Sharing access to satellite [email protected]:10000
=========== ACCESS RESTRICTIONS ==========================================================
Download : Allowed
Upload : Disallowed
Lists : Allowed
Deletes : Disallowed
NotBefore : No restriction
NotAfter : No restriction
Paths : sj://one/secret.txt
=========== SERIALIZED ACCESS WITH THE ABOVE RESTRICTIONS TO SHARE WITH OTHERS ===========
Access : 1AncRH2S9S7CwCkAygf1QpPDz4ns6NmcBh8e5fF5z8jAvVeurYCD1BSVLSRzWpTAcYwjF3LMQC5uoAfZsuVCntF1QN9xtx9JLNMqpUT85X1yc5T4QQ8snmQQE2eQXzhFPkDxrfjvaQVfboi2CR4qFKy8KiHXWMuoFJDjFr4Urg25iz5CppxGmZzhSFTxHQu3vvKGnTCpYnCLMR7iKXSsbBdrH1t6SV62QwJV45bsejr4JW8xSo3iGQTcRFUzvPMe7Mmb6CLrvqCkfguR5X7PnW5bAq3gHbXM1T34soJssKx9gCShR47vBRkesSuGW9err7uaSQvJTydiS7GD23m26VkyGzSwVRfeoZgFtWmah6yi5tP57zQ4LRUTnzQgDQJgECDr34S6hU2twNX6o7b4GKMsf2D7vLNJznaB17SM
gateway
和satellite交互的中介,客户可以通过gateway直接存取数据,最重要的是它提供了一个web console 可以通过ui存取数据,它还兼容s3,可以使用aws s3相关的生态工具。
gateway
基于MinIO,Storj V3网络的S3兼容网关
地址:https://github.com/storj/gateway
gateway-mt
基于MinIO,Storj V3网络的S3兼容网关(beta版本,新增多租户)
地址:https://github.com/storj/gateway-mt
tardigrade提供的us2使用了最新的gateway-mt,现在可以注册使用https://us2.tardigrade.io/login,目前测试阶段可以免费使用
aws s3 cli测试
aws s3 可以指定endpoint为storj gateway
下载并安装aws cli
curl "https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip" -o "awscliv2.zip"
unzip awscliv2.zip
sudo ./aws/install
配置 Access Key 和Secret Access ,可以在satellite 控制台创建grant access(类型选continue in browser),再最后一步展开 Gateway Credentials,即可生成。
$ aws configure
---
AWS Access Key ID: [Enter your Gateway's Access Key]
AWS Secret Access Key: [Enter your Gateway's Secret Key]
Default region name: [null]
Default output format: [null]
创建桶
endpoint 是gateway地址
aws s3 --endpoint=http://localhost:11000/ mb s3://two
上传
aws s3 --endpoint=http://localhost:11000/ cp awscliv2.zip s3://two
列出桶下的文件
aws s3 --endpoint=http://localhost:11000/ ls s3://two