一、概述
从具有亚像素精度的图像中裁剪矩形。
函数 getRectSubPix 从 src 中提取像素:
其中非整数坐标处的像素值是使用双线性插值检索的。 多通道图像的每个通道都是独立处理的。 图像也应该是单通道或三通道图像。 虽然矩形的中心必须在图像内部,但矩形的某些部分可能在外部。
二、getRectSubPix函数
1、函数原型
cv::getRectSubPix (InputArray image, Size patchSize, Point2f center, OutputArray patch, int patchType=-1)
2、参数详解
image | 源图像。 |
patchSize | 提取的图像块的大小。 |
center | 源图像中提取的矩形中心的浮点坐标。 中心必须在图像内部。 |
patch | 具有大小 patchSize 和与 src 相同数量的通道的提取出来的图像块。 |
patchType | 提取像素的深度。 默认情况下,它们的深度与 src 相同。 |
三、OpenCV源码
1、源码路径
opencv\modules\imgproc\src\samplers.cpp
2、源码代码
void cv::getRectSubPix( InputArray _image, Size patchSize, Point2f center,
OutputArray _patch, int patchType )
{
CV_INSTRUMENT_REGION();
Mat image = _image.getMat();
int depth = image.depth(), cn = image.channels();
int ddepth = patchType < 0 ? depth : CV_MAT_DEPTH(patchType);
CV_Assert( cn == 1 || cn == 3 );
_patch.create(patchSize, CV_MAKETYPE(ddepth, cn));
Mat patch = _patch.getMat();
#if defined (HAVE_IPP) && (IPP_VERSION_X100 >= 700)
CV_IPP_CHECK()
{
typedef IppStatus (CV_STDCALL *ippiGetRectSubPixFunc)( const void* src, int src_step,
IppiSize src_size, void* dst,
int dst_step, IppiSize win_size,
IppiPoint_32f center,
IppiPoint* minpt, IppiPoint* maxpt );
IppiPoint minpt={0,0}, maxpt={0,0};
IppiPoint_32f icenter = {center.x, center.y};
IppiSize src_size={image.cols, image.rows}, win_size={patch.cols, patch.rows};
int srctype = image.type();
ippiGetRectSubPixFunc ippiCopySubpixIntersect =
srctype == CV_8UC1 && ddepth == CV_8U ? (ippiGetRectSubPixFunc)ippiCopySubpixIntersect_8u_C1R :
srctype == CV_8UC1 && ddepth == CV_32F ? (ippiGetRectSubPixFunc)ippiCopySubpixIntersect_8u32f_C1R :
srctype == CV_32FC1 && ddepth == CV_32F ? (ippiGetRectSubPixFunc)ippiCopySubpixIntersect_32f_C1R : 0;
if( ippiCopySubpixIntersect)
{
if (CV_INSTRUMENT_FUN_IPP(ippiCopySubpixIntersect, image.ptr(), (int)image.step, src_size, patch.ptr(),
(int)patch.step, win_size, icenter, &minpt, &maxpt) >= 0)
{
CV_IMPL_ADD(CV_IMPL_IPP);
return;
}
setIppErrorStatus();
}
}
#endif
if( depth == CV_8U && ddepth == CV_8U )
getRectSubPix_Cn_<uchar, uchar, int, scale_fixpt, cast_8u>
(image.ptr(), image.step, image.size(), patch.ptr(), patch.step, patch.size(), center, cn);
else if( depth == CV_8U && ddepth == CV_32F )
getRectSubPix_8u32f
(image.ptr(), image.step, image.size(), patch.ptr<float>(), patch.step, patch.size(), center, cn);
else if( depth == CV_32F && ddepth == CV_32F )
getRectSubPix_Cn_<float, float, float, nop<float>, nop<float> >
(image.ptr<float>(), image.step, image.size(), patch.ptr<float>(), patch.step, patch.size(), center, cn);
else
CV_Error( CV_StsUnsupportedFormat, "Unsupported combination of input and output formats");
}
其中一个调用函数的源码
void getRectSubPix_Cn_(const _Tp* src, size_t src_step, Size src_size,
_DTp* dst, size_t dst_step, Size win_size, Point2f center, int cn )
{
ScaleOp scale_op;
CastOp cast_op;
Point ip;
_WTp a11, a12, a21, a22, b1, b2;
float a, b;
int i, j, c;
center.x -= (win_size.width-1)*0.5f;
center.y -= (win_size.height-1)*0.5f;
ip.x = cvFloor( center.x );
ip.y = cvFloor( center.y );
a = center.x - ip.x;
b = center.y - ip.y;
a11 = scale_op((1.f-a)*(1.f-b));
a12 = scale_op(a*(1.f-b));
a21 = scale_op((1.f-a)*b);
a22 = scale_op(a*b);
b1 = scale_op(1.f - b);
b2 = scale_op(b);
src_step /= sizeof(src[0]);
dst_step /= sizeof(dst[0]);
if( 0 <= ip.x && ip.x < src_size.width - win_size.width &&
0 <= ip.y && ip.y < src_size.height - win_size.height)
{
// extracted rectangle is totally inside the image
src += ip.y * src_step + ip.x*cn;
win_size.width *= cn;
for( i = 0; i < win_size.height; i++, src += src_step, dst += dst_step )
{
for( j = 0; j <= win_size.width - 2; j += 2 )
{
_WTp s0 = src[j]*a11 + src[j+cn]*a12 + src[j+src_step]*a21 + src[j+src_step+cn]*a22;
_WTp s1 = src[j+1]*a11 + src[j+cn+1]*a12 + src[j+src_step+1]*a21 + src[j+src_step+cn+1]*a22;
dst[j] = cast_op(s0);
dst[j+1] = cast_op(s1);
}
for( ; j < win_size.width; j++ )
{
_WTp s0 = src[j]*a11 + src[j+cn]*a12 + src[j+src_step]*a21 + src[j+src_step+cn]*a22;
dst[j] = cast_op(s0);
}
}
}
else
{
Rect r;
src = (const _Tp*)adjustRect( (const uchar*)src, src_step*sizeof(*src),
sizeof(*src)*cn, src_size, win_size, ip, &r);
for( i = 0; i < win_size.height; i++, dst += dst_step )
{
const _Tp *src2 = src + src_step;
_WTp s0;
if( i < r.y || i >= r.height )
src2 -= src_step;
for( c = 0; c < cn; c++ )
{
s0 = src[r.x*cn + c]*b1 + src2[r.x*cn + c]*b2;
for( j = 0; j < r.x; j++ )
dst[j*cn + c] = cast_op(s0);
s0 = src[r.width*cn + c]*b1 + src2[r.width*cn + c]*b2;
for( j = r.width; j < win_size.width; j++ )
dst[j*cn + c] = cast_op(s0);
}
for( j = r.x*cn; j < r.width*cn; j++ )
{
s0 = src[j]*a11 + src[j+cn]*a12 + src2[j]*a21 + src2[j+cn]*a22;
dst[j] = cast_op(s0);
}
if( i < r.height )
src = src2;
}
}
}
四、效果图像示例
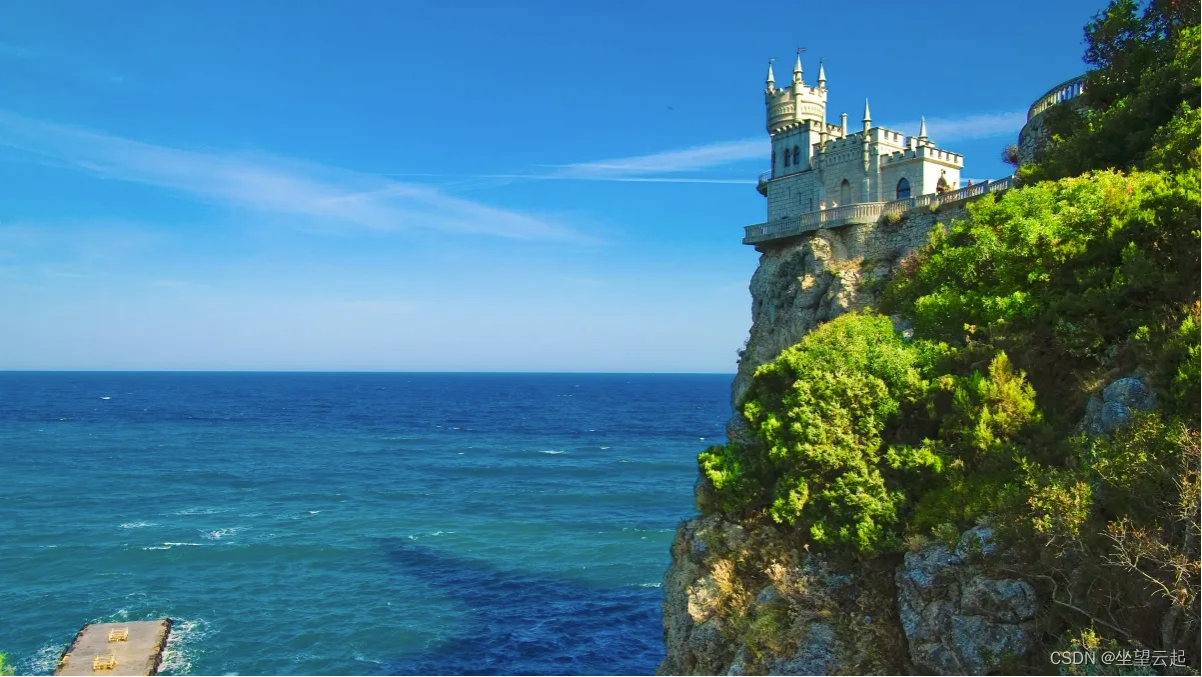
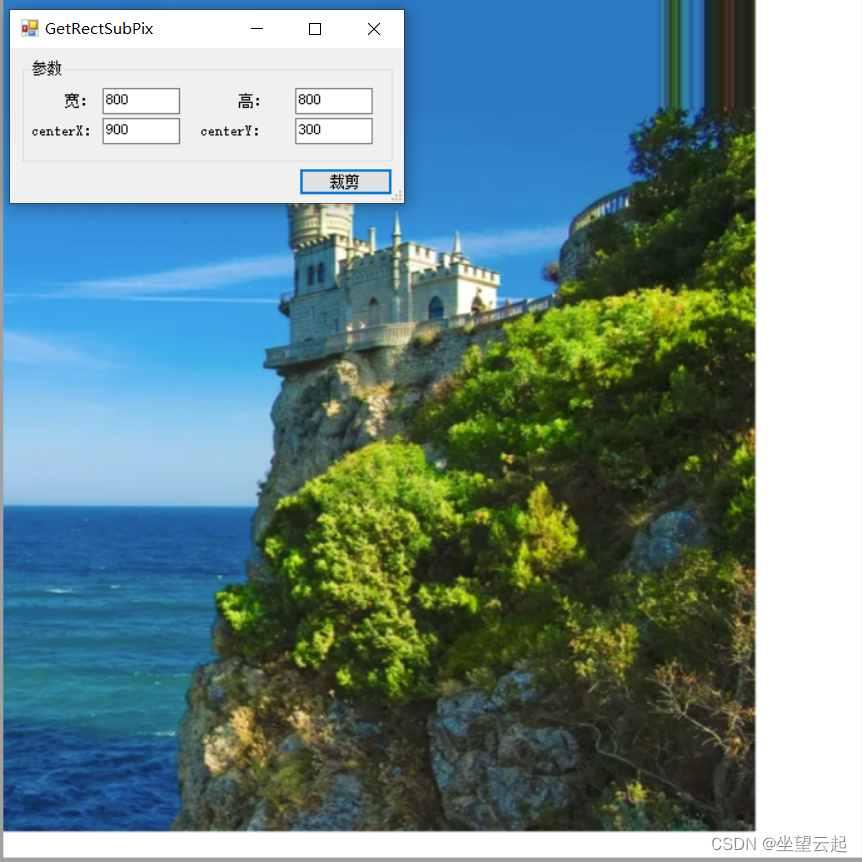