Canvas百战成神-圆
初始化容器
<canvas id="canvas"></canvas>
canvas{
border: 1px solid black;
}
让页面占满屏幕
*{
margin: 0;
padding: 0;
}
html,body{
width: 100%;
height: 100%;
overflow: hidden;
}
::-webkit-scrollbar{
display: none;
}
初始化画笔
let canvas=document.querySelector('canvas')
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
let c=canvas.getContext('2d');
以(x,y)为圆心,r为半径画一个圆
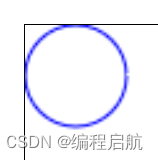
var x=20;
var y=20;
var r=20;
c.beginPath()
c.arc(x,y,r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
创建一个动画帧
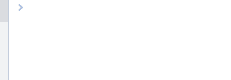
function animate(){
requestAnimationFrame(animate);
console.log(1)
}
animate()
让圆以速度vx直线运动
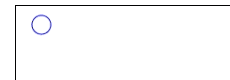
var x=20;
var y=20;
var r=10;
var vx=1;
var width=canvas.width
var height=canvas.height
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
c.beginPath()
c.arc(x,y,r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
x+=vx
}
animate()
当圆碰到边界时反弹
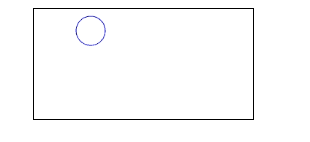
var x=30;
var y=30;
var r=20;
var vx=4;
var width=canvas.width
var height=canvas.height
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
c.beginPath()
c.arc(x,y,r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
if(x-r<0 || x+r>width){
vx=-1*vx
}
x+=vx
}
animate()
给圆加上一个y轴的速度
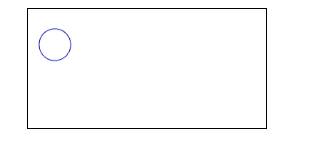
var x=30;
var y=30;
var r=20;
var vx=4;
var vy=3;
var width=canvas.width
var height=canvas.height
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
c.beginPath()
c.arc(x,y,r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
if(x-r<0 || x+r>width){
vx=-1*vx
}
if(y-r<0 || y+r>height){
vy=-1*vy
}
x+=vx
y+=vy
}
animate()
令圆的大小,生成点,速度随机
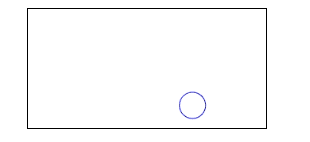
var r=(Math.random()+0.5)*10+10;
var width=canvas.width
var height=canvas.height
var x=Math.random()*(width-2*r)+r;
var y=Math.random()*(height-2*r)+r
var vx=(Math.random()-0.5)*8;
var vy=(Math.random()-0.5)*8;
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
c.beginPath()
c.arc(x,y,r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
if(x-r<0 || x+r>width){
vx=-1*vx
}
if(y-r<0 || y+r>height){
vy=-1*vy
}
x+=vx
y+=vy
}
animate()
函数化,批量化生成圆
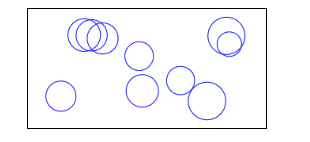
function Circle(x,y,vx,vy,r){
this.x=x;
this.y=y;
this.vx=vx;
this.vy=vy;
this.r=r;
this.draw=function(){
c.beginPath()
c.arc(this.x,this.y,this.r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
}
this.update=function(){
if(this.x-this.r<0 || this.x+this.r>width){
this.vx=-1*this.vx
}
if(this.y-this.r<0 || this.y+this.r>height){
this.vy=-1*this.vy
}
this.x+=this.vx
this.y+=this.vy
this.draw()
}
}
var width=canvas.width
var height=canvas.height
var circleArray=[]
for (var i=0;i<10;i++){
var r=(Math.random()+0.5)*10+10;
var x=Math.random()*(width-2*r)+r;
var y=Math.random()*(height-2*r)+r
var vx=(Math.random()-0.5)*8;
var vy=(Math.random()-0.5)*8;
circleArray.push(new Circle(x,y,vx,vy,r))
}
var circle = new Circle(20,50,5,5,30)
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
for (var i=0;i<circleArray.length;i++){
circleArray[i].update()
}
}
animate()
实心圆
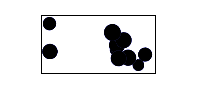
this.draw=function(){
c.beginPath()
c.arc(this.x,this.y,this.r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
c.fillStyle=colorArray[Math.floor(Math.random()*colorArray.length)]
c.fill()
}
靠近鼠标的圆变大,远离再变小
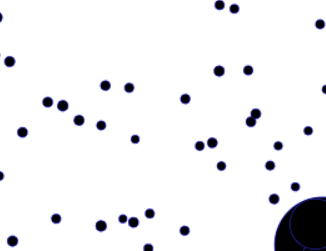
var maxRadius=40
var minRadius=4
var mouse={
x:undefined,
y:undefined
}
window.addEventListener("mousemove", function(event){
mouse.x = event.x
mouse.y = event.y
})
if(mouse.x-this.x<50 && mouse.x-this.x>-50 && mouse.y-this.y<50 && mouse.y-this.y>-50){
if(this.r<maxRadius){
this.r+=1
}
}else if(this.r>minRadius){
this.r-=1
}
给圆加上随机颜色
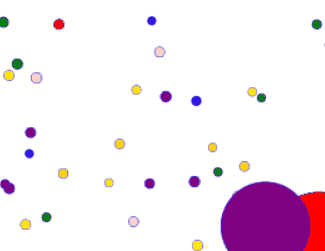
var colorArray=[
'red',
'blue',
'pink',
'orange',
'purple',
'green',
'yellow',
]
c.fillStyle=colorArray[Math.floor(Math.random()*colorArray.length)]
将随机的颜色变为圆固定的属性
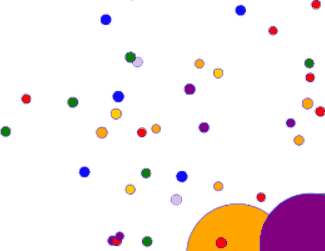
this.color=colorArray[Math.floor(Math.random()*colorArray.length)]
c.fillStyle=this.color
为每个圆设置最大最小半径
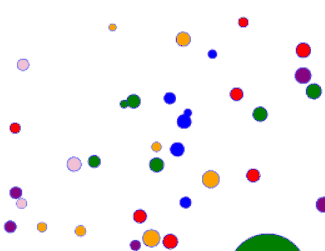
var canvas = document.getElementById('canvas');
let c=canvas.getContext('2d');
var width=canvas.width = window.innerWidth;
var height=canvas.height = window.innerHeight;
var mouse={
x:undefined,
y:undefined
}
var colorArray=[
'red',
'blue',
'pink',
'orange',
'purple',
'green',
'yellow',
]
var circleArray=[]
window.addEventListener("mousemove", function(event){
mouse.x = event.x
mouse.y = event.y
})
function Circle(x,y,vx,vy,r,maxRadius,minRadius){
this.x=x;
this.y=y;
this.vx=vx;
this.vy=vy;
this.r=r;
this.maxRadius=maxRadius
this.minRadius=minRadius
this.color=colorArray[Math.floor(Math.random()*colorArray.length)]
this.draw=function(){
c.beginPath()
c.arc(this.x,this.y,this.r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
c.fillStyle=this.color
c.fill()
}
this.update=function(){
if(this.x-this.r<0 || this.x+this.r>width){
this.vx=-1*this.vx
}
if(this.y-this.r<0 || this.y+this.r>height){
this.vy=-1*this.vy
}
this.x+=this.vx
this.y+=this.vy
if(mouse.x-this.x<50 && mouse.x-this.x>-50 && mouse.y-this.y<50 && mouse.y-this.y>-50){
if(this.r<this.maxRadius){
this.r+=1
}
}else if(this.r>this.minRadius){
this.r-=1
}
this.draw()
}
}
for (var i=0;i<200;i++){
var r=(Math.random()+0.5)*10+30;
var maxRadius=(Math.random()+0.5)*10+20;
var minRadius=(Math.random()+0.5)*4+1;
var x=Math.random()*(width-2*r)+r;
var y=Math.random()*(height-2*r)+r
var vx=(Math.random()-0.5)*2;
var vy=(Math.random()-0.5)*2;
circleArray.push(new Circle(x,y,vx,vy,r,maxRadius,minRadius))
}
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
for (var i=0;i<circleArray.length;i++){
circleArray[i].update()
}
}
animate()
增多数量
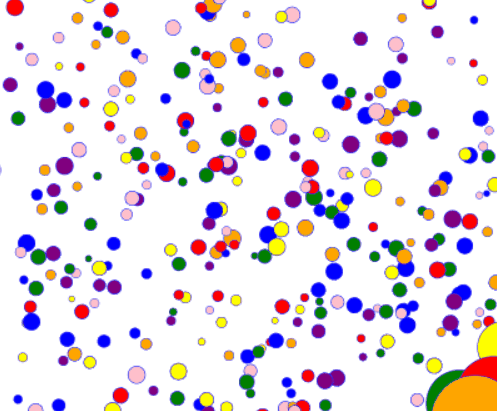
for (var i=0;i<800;i++){
}
窗口大小改变时刷新
var canvas = document.getElementById('canvas');
let c=canvas.getContext('2d');
var width=canvas.width = window.innerWidth;
var height=canvas.height = window.innerHeight;
var mouse={
x:undefined,
y:undefined
}
var colorArray=[
'red',
'blue',
'pink',
'orange',
'purple',
'green',
'yellow',
]
var circleArray=[]
window.addEventListener("mousemove", function(event){
mouse.x = event.x
mouse.y = event.y
})
window.addEventListener("resize", function(event){
width=canvas.width = window.innerWidth;
height=canvas.height = window.innerHeight;
init();
})
function Circle(x,y,vx,vy,r,maxRadius,minRadius){
this.x=x;
this.y=y;
this.vx=vx;
this.vy=vy;
this.r=r;
this.maxRadius=maxRadius
this.minRadius=minRadius
this.color=colorArray[Math.floor(Math.random()*colorArray.length)]
this.draw=function(){
c.beginPath()
c.arc(this.x,this.y,this.r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
c.fillStyle=this.color
c.fill()
}
this.update=function(){
if(this.x-this.r<0 || this.x+this.r>width){
this.vx=-1*this.vx
}
if(this.y-this.r<0 || this.y+this.r>height){
this.vy=-1*this.vy
}
this.x+=this.vx
this.y+=this.vy
if(mouse.x-this.x<50 && mouse.x-this.x>-50 && mouse.y-this.y<50 && mouse.y-this.y>-50){
if(this.r<this.maxRadius){
this.r+=1
}
}else if(this.r>this.minRadius){
this.r-=1
}
this.draw()
}
}
function init(){
circleArray=[]
for (var i=0;i<800;i++){
var r=(Math.random()+0.5)*10+30;
var maxRadius=(Math.random()+0.5)*10+20;
var minRadius=(Math.random()+0.5)*4+1;
var x=Math.random()*(width-2*r)+r;
var y=Math.random()*(height-2*r)+r
var vx=(Math.random()-0.5)*2;
var vy=(Math.random()-0.5)*2;
circleArray.push(new Circle(x,y,vx,vy,r,maxRadius,minRadius))
}
}
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
for (var i=0;i<circleArray.length;i++){
circleArray[i].update()
}
}
init()
animate()
完整代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{
margin: 0;
padding: 0;
}
html,body{
width: 100%;
height: 100%;
overflow: hidden;
}
::-webkit-scrollbar{
display: none;
}
#canvas{
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
</body>
<script>
var canvas = document.getElementById('canvas');
let c=canvas.getContext('2d');
var width=canvas.width = window.innerWidth;
var height=canvas.height = window.innerHeight;
var mouse={
x:undefined,
y:undefined
}
var colorArray=[
'red',
'blue',
'pink',
'orange',
'purple',
'green',
'yellow',
]
var circleArray=[]
window.addEventListener("mousemove", function(event){
mouse.x = event.x
mouse.y = event.y
})
window.addEventListener("resize", function(event){
width=canvas.width = window.innerWidth;
height=canvas.height = window.innerHeight;
init();
})
function Circle(x,y,dx,dy,r,maxRadius,minRadius){
this.x=x;
this.y=y;
this.dx=dx;
this.dy=dy;
this.r=r;
this.maxRadius=maxRadius
this.minRadius=minRadius
this.color=colorArray[Math.floor(Math.random()*colorArray.length)]
this.draw=function(){
c.beginPath()
c.arc(this.x,this.y,this.r,Math.PI*2,false);
c.strokeStyle='blue';
c.stroke();
c.fillStyle=this.color
c.fill()
}
this.update=function(){
if(this.x-this.r<0 || this.x+this.r>width){
this.dx=-1*this.dx
}
if(this.y-this.r<0 || this.y+this.r>height){
this.dy=-1*this.dy
}
this.x+=this.dx
this.y+=this.dy
if(mouse.x-this.x<50 && mouse.x-this.x>-50 && mouse.y-this.y<50 && mouse.y-this.y>-50){
if(this.r<this.maxRadius){
this.r+=1
}
}else if(this.r>this.minRadius){
this.r-=1
}
this.draw()
}
}
function init(){
for (var i=0;i<800;i++){
var r=(Math.random()+0.5)*10+30;
var maxRadius=(Math.random()+0.5)*10+20;
var minRadius=(Math.random()+0.5)*4+1;
var x=Math.random()*(width-2*r)+r;
var y=Math.random()*(height-2*r)+r
var dx=(Math.random()-0.5)*2;
var dy=(Math.random()-0.5)*2;
circleArray.push(new Circle(x,y,dx,dy,r,maxRadius,minRadius))
}
}
function animate(){
requestAnimationFrame(animate);
c.clearRect(0,0,width,height);
for (var i=0;i<circleArray.length;i++){
circleArray[i].update()
}
}
init()
animate()
</script>
</html>