初学javaweb,现在导师要求做一个管理系统,想好思路,设计了数据库之后,我首先来实现登录功能。由于要使用到数据库,需要下载数据库连接的jar包。
数据库:
public class DBConn { public static Connection getConnection() { Connection conn = null; try { // 注册驱动 com.mysql.jdbc.Driver Class.forName("com.mysql.jdbc.Driver"); System.out.println("驱动加载成功!"); // 获取连接 conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/workproject", "root", "root"); System.out.println("获取连接成功!"); } catch (Exception e) { // TODO Auto-generated catch block System.out.println("失败!"); e.printStackTrace(); } return conn; } public static void CloseConn(Connection conn) { try { conn.close(); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
登录页面:
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <title>login</title> </head> <body> <form action="WorkServlet?action=login" method="post"> 用户名:<input type="text" name="user"><br/><br/> 密 码:<input type="password" name="pwd"><br/><br/> <input type="submit" value="登录"> <input type="button" src="main.html" value="退出"> </form> </body>
由于是javaweb项目,所以我把表单数据提交到了servlet,并且通过传入一个参数来选择执行什么功能。
String action = request.getParameter("action"); if (action.equals("login")) { try { login(request, response); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); }
写一个登录方法:
private void login(HttpServletRequest request, HttpServletResponse response) throws SQLException { System.out.println("============="); // 接受参数 String user = request.getParameter("user"); user = (user == null) ? "" : user; String pwd = request.getParameter("pwd"); pwd = (pwd == null) ? "" : pwd; System.out.println("用户名:" + user + "密码:" + pwd); WorkDaoimpl wd2 = new WorkDaoimpl(); int result = wd2.loginfind(user, pwd); if (result == 0) { try { // request.getRequestDispatcher("index.jsp").forward(request, response); PrintWriter out = response.getWriter(); out.print("<script language='javascript'>alert('登录成功!');window.location.href='index.jsp';</script>"); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } else { try { // request.getRequestDispatcher("login.html").forward(request, response); PrintWriter out = response.getWriter(); out.print( "<script language='javascript'>alert('账号或密码错误,请重新登录!');window.location.href='login.html';</script>"); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
dao层的实现类代码:
public int loginfind(String user1, String pwd1) { int b = 0; // 获取连接 Connection conn = DBConn.getConnection(); // 创建sql语句 String sql = "select * from managers where user=? and pwd=?"; // 创建Preparedstatement对象 PreparedStatement pstmt; try { pstmt = conn.prepareStatement(sql); pstmt.setObject(1, user1); pstmt.setObject(2, pwd1); ResultSet rs = pstmt.executeQuery(); if (rs.next()) { b=0; }else { b=1; } } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return b; } }
其中我是根据rs.next()方法来判断登录时输入的数据是否跟数据库的数据相同,如果为true,则证明数据库有这个数据,登录成功,相反登录失败。
扫描二维码关注公众号,回复:
149329 查看本文章
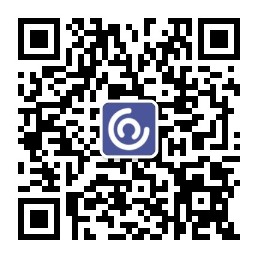