作者:小迅
链接:https://leetcode.cn/problems/add-two-numbers-ii/solutions/2328613/mo-ni-zhu-shi-chao-ji-xiang-xi-by-xun-ge-67qx/
来源:力扣(LeetCode)
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
题目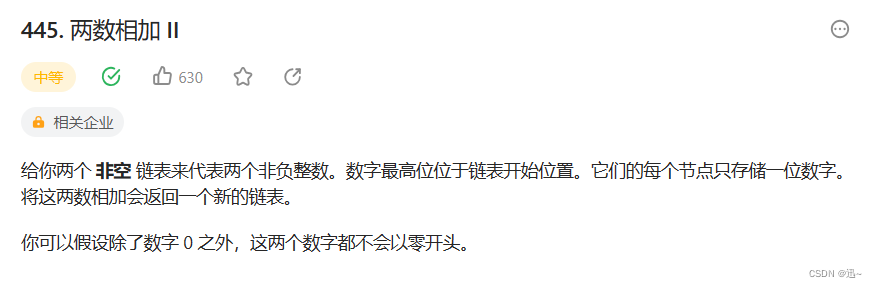
示例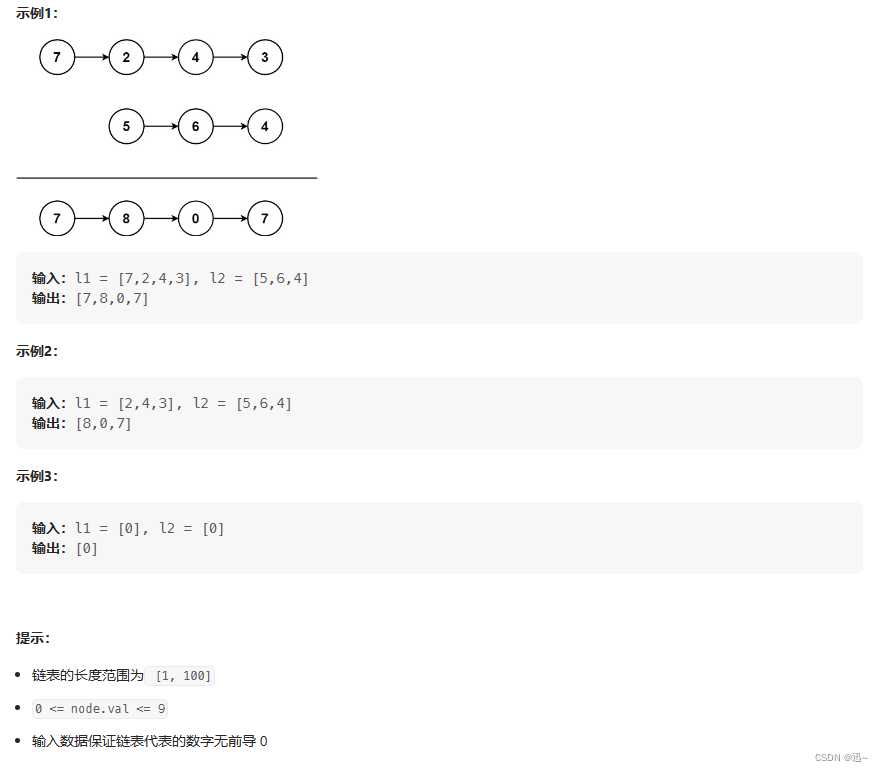
思路
题意 -> 给定两个链表,数字最高位位于链表开始位置。它们的每个节点只存储一位数字。将这两数相加返回一个新的链表。
最简单直接的方法是将两个链表反转,反转后从链表头开始枚举并进行累和,使用新链表保存其值,最后返回新链表头节点即可。注意新链表应该使用尾插法。
对于链表也可以转换为数组进行处理,枚举链表的每一个元素,将其等价存入数组中,因此题意 -> 两数之和,只不过高位在前,因此在枚举求和过程中,应该从尾到头枚举,最后返回新链表即可
代码注释超级详细
代码
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* addTwoNumbers(struct ListNode* l1, struct ListNode* l2){
int arr1[100] = {0}, arr2[100] = {0};
int index1 = 0, index2 = 0;
while (l1) {//l1转换为数组
arr1[index1++] = l1->val;
l1 = l1->next;
}
while (l2) {//l2转换为数组
arr2[index2++] = l2->val;
l2 = l2->next;
}
int arr3[101] = {0};//记录累和
int index3 = 0;
int c = 0;
for (index3; index3 < 101; index3++) {//枚举数组求和
if (index1 > 0 && index2 > 0) { //l1,l2都有元素
arr3[index3] = (arr1[--index1] + arr2[--index2] + c) % 10;
c = (arr1[index1] + arr2[index2] + c) / 10;
} else if (index1 > 0) {// l1有元素
arr3[index3] = (arr1[--index1] + c) % 10;
c = (arr1[index1] + c) / 10;
} else if (index2 > 0) {// l2有元素
arr3[index3] = (arr2[--index2] + c) % 10;
c = (arr2[index2] + c) / 10;
} else if (c){// 进位还有元素
arr3[index3] = c;
c = 0;
} else {//都没元素了
break;
}
}
struct ListNode *haed = (struct ListNode *)malloc(sizeof(struct ListNode));
haed->next = NULL;
struct ListNode *next = haed;
while (index3 > 0) {//数组转换为链表,这里可以和上面合并在一起
struct ListNode *node = (struct ListNode *)malloc(sizeof(struct ListNode));
node->next = NULL;
node->val = arr3[--index3];
next->next = node;
next = node;
}
return haed->next;
}
作者:小迅
链接:https://leetcode.cn/problems/add-two-numbers-ii/solutions/2328613/mo-ni-zhu-shi-chao-ji-xiang-xi-by-xun-ge-67qx/
来源:力扣(LeetCode)
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。