背景:
在我们做的项目中,有一个字符串的生成,我们需要double去拼接,结果发现了,拼接后的字符串,那个double值用科学计数法(科学计数法是科学家用来表示很大或很小的数字的一种方便的方法,其满足正则表达式[±][1-9]"."[0-9]+E[±][0-9]+,即数字的整数部分只有1位,小数部分至少有1位,该数字及其指数部分的正负号即使对正数也必定明确给出。)表示了。
用int、long试了一下,发现int、long不存在这个问题,只有double和float包括各自的包装类(Double和Float)也有这个问题。并且发现double类型转String类型数字只有过小或者过大时,会自动转为科学计数法。(0.00xxxx时候没问题,0.000xxxxx的时候就会自动转为科学计数,1000000时没问题,10000000的时候就会自动转为科学计数法。)
如何让结果不出现科学计数法哪?发现需要采用decimal format来格式化double与float的结果,这样转换成字符串不会采用科学计数法。
public static void main(String[] args) {
//int类型
int intValue1 = 1;
//Integer包装类
Integer intValue2 = 12700000;
//long类型
long longValue1 = 1L;
long longValue2 = 1000000000L;
//Long包装类
Long longValue3 = 1L;
Long longValue4 = 1000000000L;
//float类型
float floatValue1 = 0.001234F;
float floatValue2 = 0.00012345F;
//Float包装类
Float floatValue3 = 1234567.0F;
Float floatValue4 = 12345678.0F;
//double类型
double doubleValue1 = 0.001234;
double doubleValue2 = 0.00012345;
//double包装类
Double doubleValue3 = 1234567.00;
Double doubleValue4 = 12345678.00;
//
DecimalFormat df = new DecimalFormat("0.############");//#代表小数点后如果没有 就为空
System.out.println(
"处理前:\n" +
"int1:" + intValue1 + "\n" +
"int2:" + intValue2 + "\n" +
"long1:" + longValue1 + "\n" +
"long2:" + longValue2 + "\n" +
"long3:" + longValue3 + "\n" +
"long4:" + longValue4 + "\n" +
"float1:" + floatValue1 + "\n" +
"float2:" + floatValue2 + "\n" +
"float3:" + floatValue3 + "\n" +
"float4:" + floatValue4 + "\n" +
"double1:" + doubleValue1 + "\n" +
"double2:" + doubleValue2 + "\n" +
"double3:" + doubleValue3 + "\n" +
"double4:" + doubleValue4);
System.out.println(
"处理后:\n" +
"dfFloat1:" + df.format(floatValue1) + "\n" +
"dfFloat2:" + df.format(floatValue2) + "\n" +
"dfFloat3:" + df.format(floatValue3) + "\n" +
"dfFloat4:" + df.format(floatValue4) + "\n" +
"dfDouble1:" + df.format(doubleValue1) + "\n" +
"dfDouble2:" + df.format(doubleValue2) + "\n" +
"dfDouble3:" + df.format(doubleValue3) + "\n" +
"dfDouble4:" + df.format(doubleValue4));
}
结果如下:
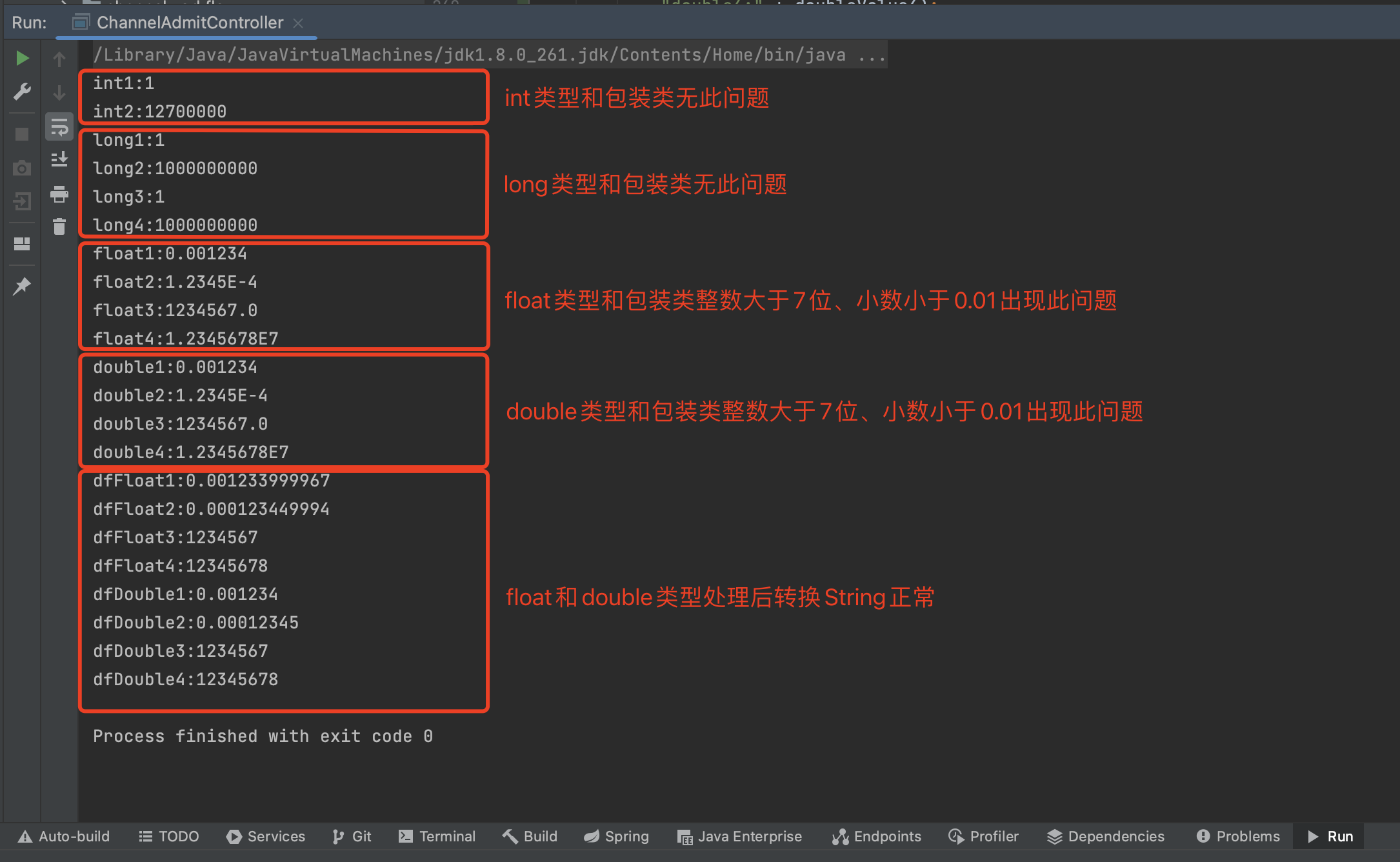
DecimalFormat用法
DecimalFormat 是 NumberFormat 的一个具体子类,用于格式化十进制数字。
DecimalFormat 包含一个模式 和一组符号
符号含义:
0 一个数字
# 一个数字,不包括 0
. 小数的分隔符的占位符
, 分组分隔符的占位符
; 分隔格式。
- 缺省负数前缀。
% 乘以 100 和作为百分比显示
? 乘以 1000 和作为千进制货币符显示;用货币符号代替;如果双写,用 国际货币符号代替。如果出现在一个模式中,用货币十进制分隔符代 替十进制分隔符。
X 前缀或后缀中使用的任何其它字符,用来引用前缀或后缀中的特殊字符。
1.保留两位小数(截断)
double num = 11.256;
DecimalFormat df = new DecimalFormat("#.##");
//指定RoundingMode
df.setRoundingMode(RoundingMode.DOWN);
String str = df.format(num);
double formatNum = Double.parseDouble(str);
System.out.println(formatNum);
2.保留两位小数(四舍五入)
double num = 11.256;
DecimalFormat df = new DecimalFormat("#.##");
//指定RoundingMode
df.setRoundingMode(RoundingMode.HALF_UP);
String str = df.format(num);
double formatNum = Double.parseDouble(str);
System.out.println(formatNum);
3.常用RoundingMode
HALF_EVEN -----DecimalFormat默认的RoundingMode为RoundingMode.HALF_EVEN,也称为“银行家舍入法”,主要在美国使用。四舍六入,五分向相邻的偶数舍入。
以下例子为保留小数点1位,那么这种舍入方式下的结果。
1.15–>1.2 1.25–>1.2
HALF_UP — 四舍五入
HALF_DOWN — 五舍六入
2.5 —> 2
2.6 —> 3
DOWN — 直接截断
UP — 始终对非零舍弃部分前面的数字加 1
4.占位符
0表示数字占位符,实际位数不够时补零
比实际数字的位数多,不足的地方用0补上。
new DecimalFormat(“00.00”).format(3.14) //结果:03.14
new DecimalFormat(“0.000”).format(3.14) //结果: 3.140
new DecimalFormat(“00.000”).format(3.14) //结果:03.140
整数部分比实际数字的位数少,整数部分不改动
小数部分比实际数字的位数少,根据小数部分占位符数量保留小数
new DecimalFormat(“0.000”).format(13.146) //结果:13.146
new DecimalFormat(“00.00”).format(13.146) //结果:13.15
new DecimalFormat(“0.00”).format(13.146) //结果:13.15
#表示数字占位符,实际位数不够时不补零
比实际数字的位数多,不变
new DecimalFormat("##.##").format(3.14) //结果:3.14
new DecimalFormat("#.###").format(3.14) //结果: 3.14
new DecimalFormat("##.###").format(3.14) //结果:3.14
整数部分比实际数字的位数少,整数部分不改动
小数部分比实际数字的位数少,根据小数部分占位符数量保留小数
new DecimalFormat("#.###").format(13.146) //结果:13.146
new DecimalFormat("##.##").format(13.146) //结果:13.15
new DecimalFormat("#.##").format(13.146) //结果:13.15
常用实例:
public static void main(String[] args) {
DecimalFormat df = new DecimalFormat();
double data = 1234.56789;
System.out.println("格式化之前的数字: " + data);
String style = "0.0";//定义要显示的数字的格式
df.applyPattern(style);// 将格式应用于格式化器
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
style = "00000.000 kg";//在格式后添加诸如单位等字符
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的"#"表示如果该位存在字符,则显示字符,如果不存在,则不显示。
style = "##000.000 kg";
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的"-"表示输出为负数,要放在最前面
style = "-000.000";
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的","在数字中添加逗号,方便读数字
style = "-0,000.0#";
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的"E"表示输出为指数,"E"之前的字符串是底数的格式,
// "E"之后的是字符串是指数的格式
style = "0.00E000";
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的"%"表示乘以100并显示为百分数,要放在最后。
style = "0.00%";
df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df.format(data));
// 模式中的"\u2030"表示乘以1000并显示为千分数,要放在最后。
style = "0.00\u2030";
//在构造函数中设置数字格式
DecimalFormat df1 = new DecimalFormat(style);
//df.applyPattern(style);
System.out.println("采用style: " + style + " 格式化之后: " + df1.format(data));
}
结果展示:
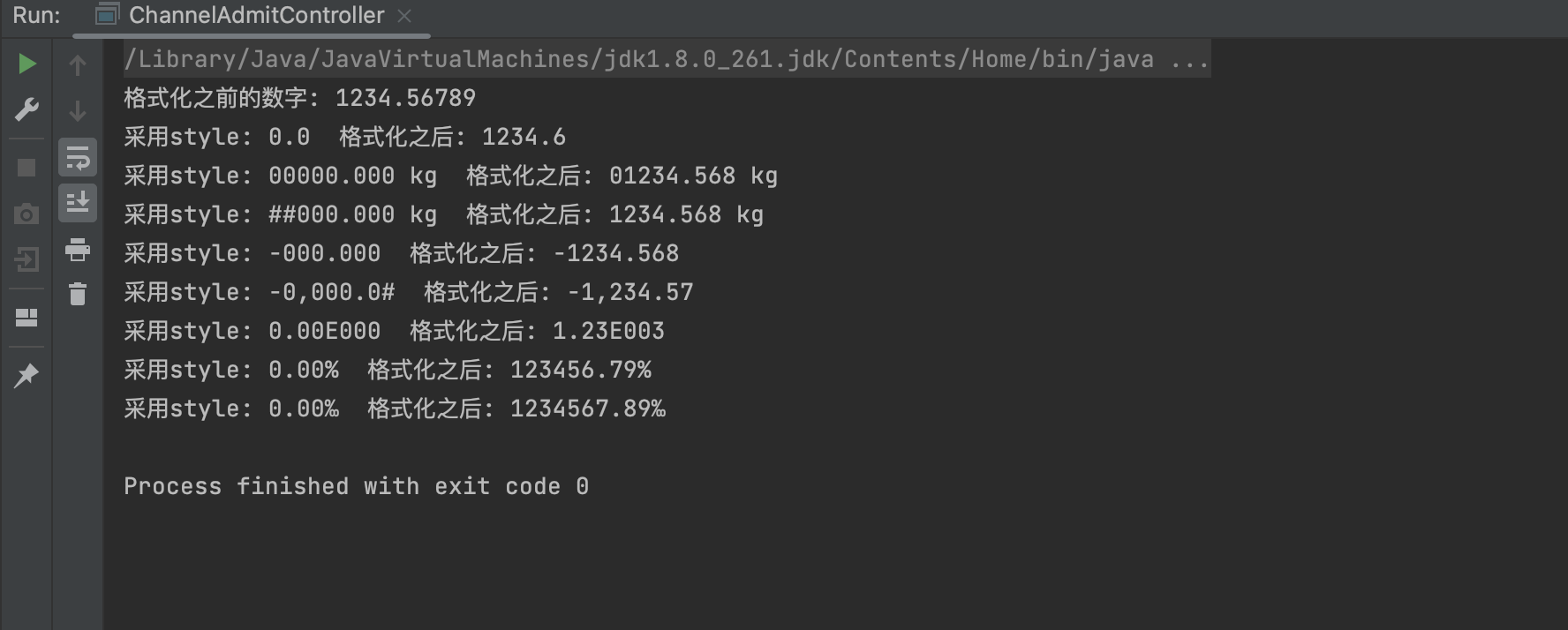