该资源是关于Java的师生管理系统,可以学习借鉴一下。
继续进行讲解,如果前面有不懂的,可以翻阅一下同专栏的其他文章,该专栏是针对Java的知识从0开始。
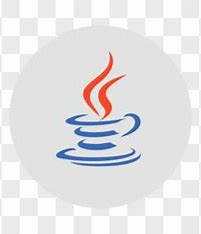
JavaBean
一个Java中的类,其对象可用于程序中封装数据
举例:学生类,手机类
要求:1、成员变量使用private修饰
2、提供每一个成员变量对应的setXxx()/getXxx()
3、提供一个无参构造方法
案例
public class crj {
public static void main(String[] args) {
Student s = new Student();
s.setName("林青霞");
s.setAge(30);
System.out.println(s.getName() + "," + s.getAge());
}
}
public class Student {
private String name;
private int age;
public Student() {
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAge(int age) {
this.age = age;
}
public int getAge() {
return age;
}
}
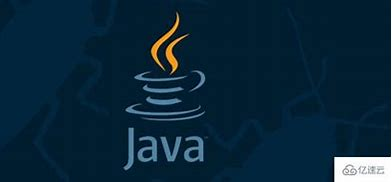
再举一个 手机JavaBean
public class crj {
public static void main(String[] args) {
Phone p1=new Phone();
p1.setBrand("小米");
p1.setPrice(2999);
System.out.println(p1.getBrand()+","+p1.getPrice());
Phone p2 =new Phone("小米",2999);
System.out.println(p2.getBrand()+","+p2.getPrice());
}
}
public class Phone {
private String brand;
private int price;
public Phone(){
}
public Phone(String brand, int price) {
this.brand = brand;
this.price = price;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
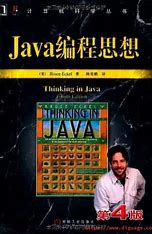
Scanner基本使用
文本扫描程序,可以获取基本类型数据和字符串数据
当我们需要从控制台或文件中读取输入时,Java中的Scanner类提供了一种
方便的方式来实现。Scanner类位于java.util包中,它可以解析基本类型和
字符串,并提供了多种方法来读取不同类型的输入。
案例
import java.util.Scanner;
public class crj {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个整数:");
int i = sc.nextInt();
System.out.println("你输入的整数是" + i);
}
}
一旦获得Scanner对象,我们可以使用各种方法来读取输入。下面介绍几个常用
的方法:
next(): 读取并返回输入中的下一个字符串,遇到空格或换行符时停止读取。
nextInt(): 读取并返回输入中的下一个整数。
nextDouble(): 读取并返回输入中的下一个浮点数。
nextLine(): 读取一行输入,并返回该行的字符串,可以包含空格。
再举一个数据求和的案例
import java.util.Scanner;
public class crj {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.println("请输入第一个数字:");
int First=sc.nextInt();
System.out.println("请输入第二个数字:");
int Second=sc.nextInt();
int sum =First+Second;
System.out.println("求和结果是:"+sum);
}
}
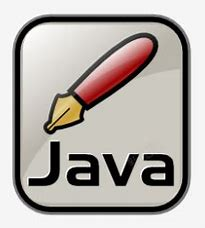
再举一个随机数的案例
import java.util.Random;
public class crj {
public static void main(String[] args) {
Random r=new Random();
int number=r.nextInt(101)+1;
System.out.println(number);
}
}
综合案例:猜数字游戏
import java.util.Random;
import java.util.Scanner;
public class crj {
public static void main(String[] args) {
Random r= new Random();
int number=r.nextInt(100)+1;
System.out.println("系统已经产生一个1-100的随机整数");
while (true) {
Scanner sc =new Scanner(System.in);
System.out.println("请输入你要猜的数字:");
int guessNumber=sc.nextInt();
if (guessNumber > number) {
System.out.println("你猜的数据" + guessNumber + "大了");
} else if (guessNumber < number) {
System.out.println("你猜的数据" + guessNumber + "小了");
} else {
System.out.println("恭喜你猜中了");
break;
}
}
}
}
GUI Graphical User Interface 图形用户接口
java.awt包:——抽象窗口工具包
javax.swing包:
组件:是具有图形表示的对象,该图形表示可以显示在屏幕上并且可以和
用户交互
JFrame 一个顶层窗口
构造方法 JFrame():构造一个最初不可见的窗体
成员方法
void setVisible(boolean b):显示或隐藏窗口
void setSize(int width,int height):调整大小(像素)
void setTitle(String title) 设置窗口标题
void setLocationRelativeTo(Component c)设置位置 值为null 则窗体
位于屏幕中央
void setDefaultCloseOperation(int operation)设置窗口关闭默认操
作 3表示窗口关闭时退出应用程序
void setAlwaysOnTop(boolean alwaysOnTop) 设置此窗口位于其他窗口
之上
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("百度一下就知道了");
jf.setSize(400,400);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setVisible(true);
}
}
JButton 按钮的实现
构造方法
JButton(String text):创建一个带文本的按钮
成员方法
void setSize(int width,int height) 设置大小
void setLocation(int x,int y) 设置位置(x,y坐标)
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("窗体中创建按钮");
jf.setSize(400,400);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JButton btn=new JButton("按钮");
btn.setBounds(100,100,100,20);
JButton btn2=new JButton("按钮2");
btn2.setBounds(100,120,100,20);
jf.add(btn);
jf.add(btn2);
jf.setVisible(true);
}
}
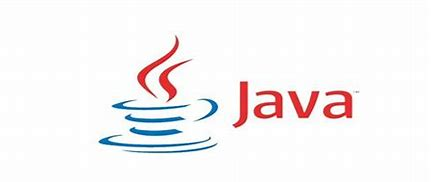
JLabel 短文本字符串或图像的显示区域
构造方法
JLabel(String text):使用指定的文本创建JLabel实例
JLabel(lcon image):使用指定的图像创建JLabel实例
Imagelcon(String filename):从指定的文件创建Imagelcon
文件路径:绝对路径和相对路径
成员方法
void setBounds
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("显示文本和图像");
jf.setSize(400,400);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JLabel jLabel=new JLabel("好好学习");
jLabel.setBounds(0,0,100,20);
ImageIcon icon1 = new ImageIcon("文件位置");
JLabel jLabel1 = new JLabel(icon1);
jLabel1.setBounds(50,50,57,57);
jf.add(jLabel);
jf.add(jLabel1);
jf.setVisible(true);
}
}
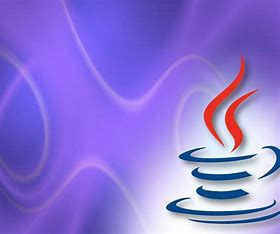
GUI案例1 用户登录
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("用户登录");
jf.setSize(400,300);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JLabel usernameLable=new JLabel("用户名");
usernameLable.setBounds(50,50,50,20);
jf.add(usernameLable);
JTextField usernameFiled=new JTextField();
usernameFiled.setBounds(150,50,180,20);
jf.add(usernameFiled);
JLabel passworldLable=new JLabel("密码");
passworldLable.setBounds(50,100,50,20);
jf.add(passworldLable);
JPasswordField passworldFiled=new JPasswordField();
passworldFiled.setBounds(150,100,180,20);
jf.add(passworldFiled);
JButton loginButton=new JButton("登录");
loginButton.setBounds(50,200,280,20);
jf.add(loginButton);
jf.setVisible(true);
}
}
GUI案例2 聊天室
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("聊天室");
jf.setSize(400,300);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JTextArea massageArea=new JTextArea();
massageArea.setBounds(10,10,360,200);
jf.add(massageArea);
JTextField massageField=new JTextField();
massageField.setBounds(10,230,180,20);
jf.add(massageField);
JButton sendButton=new JButton("发送");
sendButton.setBounds(200,230,70,20);
jf.add(sendButton);
JButton clearButton=new JButton("清空聊天");
clearButton.setBounds(280,230,100,20);
jf.add(clearButton);
jf.setVisible(true);
}
}
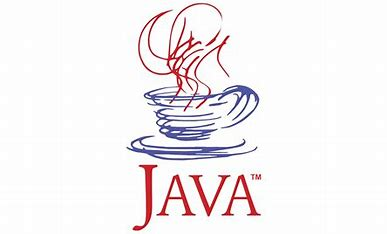
GUI案例3 猜数字
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("猜数字");
jf.setSize(400,300);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JLabel messageLable=new JLabel("系统产生了一个1~100之间的数字,请猜一猜");
messageLable.setBounds(70,50,350,20);
jf.add(messageLable);
JTextField numberFiled=new JTextField();
numberFiled.setBounds(120,100,150,20);
jf.add(numberFiled);
JButton guessButton=new JButton("我猜");
guessButton.setBounds(150,150,100,20);
jf.add(guessButton);
jf.setVisible(true);
}
}
GUI案例4 手机日期和时间显示
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("手机日期和时间显示");
jf.setSize(400,300);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JLabel dataLable=new JLabel("日期");
dataLable.setBounds(50,50,100,20);
jf.add(dataLable);
JLabel showDataLable=new JLabel("xxxx年xx月xx日");
showDataLable.setBounds(50,80,200,20);
jf.add(showDataLable);
JLabel timeLAble=new JLabel("时间");
timeLAble.setBounds(50,150,100,20);
jf.add(timeLAble);
JLabel showTimeLable=new JLabel("xx:xx");
showTimeLable.setBounds(50,180,200,20);
jf.add(showTimeLable);
jf.setVisible(true);
}
}
GUI案例5 考勤查询
import com.itheima_05.DateChooser;
import javax.swing.*;
public class crj {
public static void main(String[] args) {
JFrame jf=new JFrame();
jf.setTitle("考勤查询");
jf.setSize(400,300);
jf.setDefaultCloseOperation(3);
jf.setLocationRelativeTo(null);
jf.setAlwaysOnTop(true);
jf.setLayout(null);
JLabel dataLable=new JLabel("考勤日期");
dataLable.setBounds(50,20,100,20);
jf.add(dataLable);
JLabel startDateLable =new JLabel("开始时间");
startDateLable.setBounds(50,70,100,20);
jf.add(startDateLable);
DateChooser dateChooser1 = DateChooser.getInstance("yyyy/MM/dd");
DateChooser dateChooser2 = DateChooser.getInstance("yyyy/MM/dd");
JTextField startDateField=new JTextField();
startDateField.setBounds(50,100,100,20);
dateChooser1.register(startDateField);
jf.add(startDateField);
JLabel endDateLable =new JLabel("结束时间");
endDateLable.setBounds(250,70,100,20);
jf.add(endDateLable);
JTextField endDateField=new JTextField();
endDateField.setBounds(250,100,100,20);
dateChooser2.register(endDateField);
jf.add(endDateField);
JButton confirmButton=new JButton("确定");
confirmButton.setBounds(250,180,60,20);
jf.add(confirmButton);
jf.setVisible(true);
}
}
通过丰富的案例和详细的知识点讲解,丰富的案例和详细的知识点讲解,希望能对大家带来点帮助。