文章目录
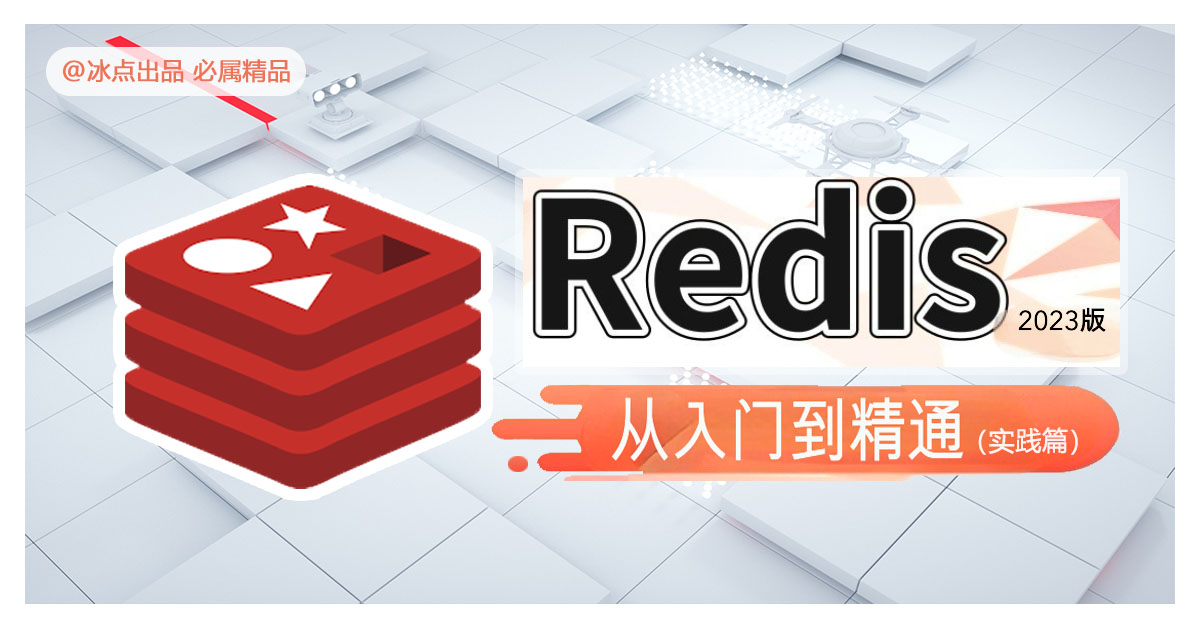
1.前言
Redisson是一个基于Redis的分布式Java对象和数据结构库,它提供了丰富的功能和易于使用的API,使开发人员能够轻松地在分布式环境中操作和管理数据。
作为一个分布式对象和数据结构库,Redisson提供了许多常见的数据结构和算法的实现,包括通用对象桶、二进制流、地理空间对象桶、BitSet、原子长整型、原子双精度浮点数、话题(订阅分发)、布隆过滤器和基数估计算法。这些数据结构和算法为开发人员提供了处理分布式数据的工具,从而简化了复杂性,提高了效率。简直就是一个Redis的最佳实践框架和最牛X的Redis客户端工具宝箱,基本上覆盖了所有场景
。
通过Redisson,开发人员可以使用简单而一致的API来存储和检索对象,处理二进制数据,管理地理位置信息,操作位集合,进行原子操作,进行发布-订阅消息传递,实现布隆过滤器和基数估计等功能。Redisson还提供了许多附加功能,如分布式锁、分布式信号量、分布式队列和分布式限流器等,进一步增强了分布式应用的能力。
Redisson的设计目标是提供高性能、可扩展和可靠的分布式数据操作解决方案。它与Redis数据库紧密集成,并利用Redis的特性来实现分布式对象和数据结构的存储和管理。Redisson还支持与Spring框架的无缝集成,使开发人员能够更方便地在Spring应用程序中使用Redisson功能。
所以我们本篇文章了解一下Redisson 在项目实践中最常用的5种场景,分别搞了一个示例方便大家理解。
2.使用方式
1. 添加Redisson依赖:
在Spring Boot项目的pom.xml文件中添加Redisson的依赖。
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson-spring-boot-starter</artifactId>
<version>3.15.5</version>
</dependency>
2. 配置Redis连接信息
在Spring Boot项目的application.properties或application.yml文件中配置Redis连接信息,包括主机地址、端口、密码等。例如:
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
3. 使用场景
3.1. 分布式锁
例如,你可以在Spring Boot的Service类中注入RedissonClient并使用它进行操作:
import org.redisson.api.RedissonClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Autowired
private RedissonClient redissonClient;
public void myMethod() {
// 获取分布式锁
RLock lock = redissonClient.getLock("myLock");
try {
// 尝试加锁,如果加锁成功,则执行加锁后的逻辑
if (lock.tryLock()) {
// 执行加锁后的逻辑
// ...
}
} finally {
// 释放锁
lock.unlock();
}
}
}
3.2. 限流器(Rate Limiter)
import org.redisson.api.RRateLimiter;
import org.redisson.api.RedissonClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyService {
@Autowired
private RedissonClient redissonClient;
public void myMethod() {
// 获取限流器
RRateLimiter limiter = redissonClient.getRateLimiter("myLimiter");
// 定义限流速率,例如每秒最多允许10个操作
limiter.trySetRate(RateType.OVERALL, 10, 1, RateIntervalUnit.SECONDS);
// 尝试获取许可
boolean acquired = limiter.tryAcquire();
if (acquired) {
// 执行需要限流的操作
// ...
} else {
// 限流逻辑,例如返回错误信息或执行降级处理
// ...
}
}
}
3.3. 可过期的对象(Expirable Object)
在Redis中,Hash结构是一种用于存储键值对的数据结构。每个Hash结构都可以包含多个字段(field)和对应的值(value)。而要为Hash结构中的二级key设置过期时间,可以使用Redisson的RMapCache接口。如果使用Redisson 来实现对Hash结构中二级key的值设置过期时间,其实很简单了。
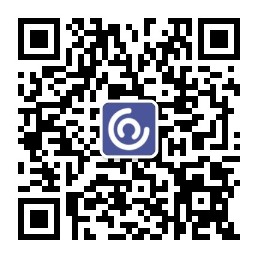
我们使用redissonClient.getMapCache("myHash")
获取一个名为"myHash"的可过期对象的Hash结构。然后,我们可以使用put()
方法将具有过期时间的二级键值对存储到Hash结构中,并指定过期时间和时间单位。存储的二级键值对将会在指定的过期时间后自动过期。
在setHashValueWithExpiration()
方法中,我们传入Hash结构的一级键(hashKey)、二级键(fieldKey)、值(value)、过期时间和时间单位,将值存储到Hash结构中的二级键,并为其设置过期时间。
在getHashValue()
方法中,我们根据Hash结构的一级键和二级键从Hash结构中获取对应的值。
通过使用Redisson的RMapCache
接口,你可以方便地为Hash结构中的二级键值对设置过期时间,并且无需手动处理过期逻辑。Redisson会自动管理过期和清理操作,简化了在分布式环境中使用可过期的Hash结构的开发工作。
import org.redisson.api.RMapCache;
import org.redisson.api.RedissonClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.concurrent.TimeUnit;
@Component
public class HashService {
@Autowired
private RedissonClient redissonClient;
public void setHashValueWithExpiration(String hashKey, String fieldKey, Object value, long expirationTime, TimeUnit timeUnit) {
RMapCache<String, Object> hash = redissonClient.getMapCache("myHash");
hash.put(hashKey, fieldKey, value, expirationTime, timeUnit);
}
public Object getHashValue(String hashKey, String fieldKey) {
RMapCache<String, Object> hash = redissonClient.getMapCache("myHash");
return hash.get(hashKey, fieldKey);
}
}
3.4. 信号量(Semaphore)
redissonClient.getSemaphore(“mySemaphore”)获取一个名为"mySemaphore"的信号量。然后,使用trySetPermits()方法设置信号量的初始数量,例如设置为10个。在myMethod()方法中,我们使用acquire()方法尝试获取信号量,如果获取到信号量,则执行需要受信号量限制的操作。在操作完成后,使用release()方法释放信号量。
- 通过使用Redisson的信号量功能,你可以控制在分布式环境中对某个资源的并发访问数量,限制并发访问的能力,从而保护资源的稳定性和可用性。
import org.redisson.api.RSemaphore;
import org.redisson.api.RedissonClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ResourceService {
private final RedissonClient redissonClient;
@Autowired
public ResourceService(RedissonClient redissonClient) {
this.redissonClient = redissonClient;
}
public void accessResource() {
RSemaphore resourceSemaphore = redissonClient.getSemaphore("resourceSemaphore");
resourceSemaphore.trySetPermits(10);
try {
resourceSemaphore.acquire();
// 执行需要受信号量限制的操作,访问资源
// ...
} catch (InterruptedException e) {
// 处理中断异常
// ...
} finally {
resourceSemaphore.release();
}
}
}
3.5. 分布式调度器(Distributed Scheduler)
我们使用redissonClient.getMapCache("myHash")
获取一个名为"myHash"的可过期对象的Hash结构。然后,我们可以使用put()
方法将具有过期时间的二级键值对存储到Hash结构中,并指定过期时间和时间单位。存储的二级键值对将会在指定的过期时间后自动过期。
在setHashValueWithExpiration()
方法中,我们传入Hash结构的一级键(hashKey)、二级键(fieldKey)、值(value)、过期时间和时间单位,将值存储到Hash结构中的二级键,并为其设置过期时间。
在getHashValue()
方法中,我们根据Hash结构的一级键和二级键从Hash结构中获取对应的值。
通过使用Redisson的RMapCache
接口,你可以方便地为Hash结构中的二级键值对设置过期时间,并且无需手动处理过期逻辑。Redisson会自动管理过期和清理操作,简化了在分布式环境中使用可过期的Hash结构的开发工作。
import org.redisson.api.RScheduledExecutorService;
import org.redisson.api.RedissonClient;
import org.redisson.api.annotation.RInject;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.concurrent.TimeUnit;
@Component
public class DistributedScheduler {
private final RedissonClient redissonClient;
@Autowired
public DistributedScheduler(RedissonClient redissonClient) {
this.redissonClient = redissonClient;
}
/**
* 安排一个延迟执行的分布式任务
*
* @param task 要执行的任务
* @param delay 延迟时间
* @param timeUnit 时间单位
*/
public void scheduleTask(Runnable task, long delay, TimeUnit timeUnit) {
RScheduledExecutorService executorService = redissonClient.getExecutorService("myScheduler");
executorService.schedule(task, delay, timeUnit);
}
/**
* 安排一个以固定速率重复执行的分布式任务
*
* @param task 要执行的任务
* @param initialDelay 初始延迟时间
* @param period 重复执行的周期
* @param timeUnit 时间单位
*/
public void scheduleTaskAtFixedRate(Runnable task, long initialDelay, long period, TimeUnit timeUnit) {
RScheduledExecutorService executorService = redissonClient.getExecutorService("myScheduler");
executorService.scheduleAtFixedRate(task, initialDelay, period, timeUnit);
}
}
5. 源码地址
https://github.com/wangshuai67/Redis-Tutorial-2023
6. Redis从入门到精通系列文章
- 《Redis使用Lua脚本和Redisson来保证库存扣减中的原子性和一致性》
- 《SpringBoot Redis 使用Lettuce和Jedis配置哨兵模式》
- 《Redis【应用篇】之RedisTemplate基本操作》
- 《Redis 从入门到精通【实践篇】之SpringBoot配置Redis多数据源》
- 《Redis 从入门到精通【进阶篇】之三分钟了解Redis HyperLogLog 数据结构》
- 《Redis 从入门到精通【进阶篇】之三分钟了解Redis地理位置数据结构GeoHash》
- 《Redis 从入门到精通【进阶篇】之高可用哨兵机制(Redis Sentinel)详解》
- 《Redis 从入门到精通【进阶篇】之redis主从复制详解》
- 《Redis 从入门到精通【进阶篇】之Redis事务详解》
- 《Redis从入门到精通【进阶篇】之对象机制详解》
- 《Redis从入门到精通【进阶篇】之消息传递发布订阅模式详解》
- 《Redis从入门到精通【进阶篇】之持久化 AOF详解》
- 《Redis从入门到精通【进阶篇】之持久化RDB详解》
- 《Redis从入门到精通【高阶篇】之底层数据结构字典(Dictionary)详解》
- 《Redis从入门到精通【高阶篇】之底层数据结构快表QuickList详解》
- 《Redis从入门到精通【高阶篇】之底层数据结构简单动态字符串(SDS)详解》
- 《Redis从入门到精通【高阶篇】之底层数据结构压缩列表(ZipList)详解》
- 《Redis从入门到精通【进阶篇】之数据类型Stream详解和使用示例》
大家好,我是冰点,今天的【Redis实践篇】使用Redisson 优雅实现项目实践过程中的5种场景,全部内容就是这些。如果你有疑问或见解可以在评论区留言。
7.参考文档
Redisson官方文档 https://github.com/redisson/redisson/wiki/Table-of-Content