【任务介绍】
1.任务描述
商城仓库中有多种商品,商品每次进货需要生成一条进货记录保存到文件。本案
例要求编写一个记录商城进货交易的程序,使用字节流将商场的进货信息记录在本地
的 CSV 文件中。程序具体要求如下。
程序启动后,先打印库存中现有的所有商品信息。
进货时,用户输入商品编号,程序根据该编号查询到相应商品信息,并打印该商
品信息。用户输入进货的数量后,将当次的入库信息保存至本地的 CSV 文件中,并更
新程序中的商品的库存数量(新数量=旧数量+进货数量)。
在本项目保存的 CSV 文件中,首行是标题行,每条记录包含商品编号、商品名称、
购买数量、单价、总价、联系人字段,字段之间直接用英文逗号“,”分隔。
每天的多次进货记录都保存在同一个文件中。文件名的命名格式为“进货记录表”
- 当天日期 + “.csv”,如“进货记录表 20200430.csv”。保存文件时,需要判断本地中
是否已存在当天的文件,如果存在则追加数据;如果不存在则先新建文件。
【任务目标】
学会分析"商城进货交易记录"程序的实现思路。
根据思路独立完成"商城进货交易记录"的源代码编写、编译及运行。
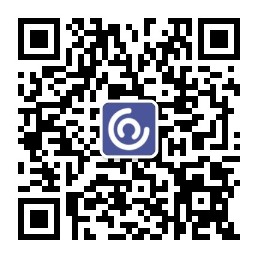
掌握 File 类和字节流操作本地文件的方法。
掌握 ArrayList 和 Date、 StringBuffer 的使用,以及异常的处理。
了解 CSV 文件格式。
【实现思路】
1、 为了方便保存商品的相关信息,可以将商品信息封装为一个实体类 Good。
商品类的属性有:
int id; // 编号
String name; // 名称
double price; // 单价
int amount; // 数量
double totalPrice; // 总价
String people; //联系人
程序需要打印商品的相关信息,所以需要重写 toString()方法,使其能返回规定格式
的字符串。要观察打印结果,自己分析字符串的格式。
2、 为了演示商城进货,我们需要将商城封装为一个类 Mall。
商城仓库有多种商品,在 Mall 类中定义一个 ArrayList 集合(静态成员)用于模拟
商城仓库。向集合中添加有具体信息的商品对象,这样仓库就有了商品。
Mall 类的方法有:
主方法 main
库存商品初始化方法:void initStock()
显示库存商品信息方法:void showStock()
商品入库操作方法:void intoStock()
根据商品编号查找商品信息的方法: Good getGoodById(int goodId)
这些方法中,商品入库方法 intoStock()是最核心和最复杂的部分。其过程为:获取
用户输入的商品编号和购买数量,如果商品编号正确,且购买数量也正常,则商品进
货成功,并将本次商品的进货信息保存到 csv 文件中,同时要将库存数量增加。
可通过 Scanner 类的 nextInt()方法获取商品编号,根据这个编号到库存中查询此商
品的信息。如果查到了商品的信息,获取进货的数量,并创建一个代表进货商品的对
象 good,注意该对象的数量为进货量,总价为进货总价。
最后将本次进货对象信息保存到文件。文件操作比较复杂,也比较独立。可单独
设计一个文件操作工具类 FileUtil,在该类中定义一个保存进货数据的方法 void
saveGood(Good good)。
3、文件操作工具类 FileUtil,它只有一个静态方法 void saveGood(Good good),实现将
销售商品对象 good 的信息写入到 csv 文件。
首先需先拼凑好 csv 文件名(比如“进货记录表 20211111.csv”),再判断本地是否已
存在此文件,这里可通过 File 类对象的成员方法 isExist()方法进行判断。若这个文件已
存在,那么就通过输出流向文件末尾追加销售信息,如果不存在,说明之前并没有生
成当日的销售信息,则需要新建此文件。
数据保存的流程是: Good 对象属性StringBufferStringbyte[]输出流文件
【实现代码】
( 1) 将商品信息封装成一个实体类 Good。
(2)定义 Mall 类来封装商城,实现入库和显示商品信息。
(3)定义工具类 FileUtil 保存进货信息
package cn.edu.gpnu.bank.cn.edu.gpnu.exp11;
public class Good {
int id;
String name;
double price;
int amount;
double totalPrice;
String people;
public Good(int id, String name, String people,double price, int amount) {
this.id = id;
this.name = name;
this.price = price;
this.amount = amount;
this.people = people;
this.totalPrice=price*amount;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getAmount() {
return amount;
}
public void setAmount(int amount) {
this.amount = amount;
}
public double getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(double totalPrice) {
this.totalPrice = totalPrice;
}
public String getPeople() {
return people;
}
public void setPeople(String people) {
this.people = people;
}
public String toString() {
return "进货商品编号:" + getId() + ", 商品名称" + getName() + ", 联系人:" + getPeople() + ", 单价" + getPrice() + ", 库存数量:" + getAmount() + "}";
}
}
package cn.edu.gpnu.bank.cn.edu.gpnu.exp11;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.Scanner;
public class Mall {
//创建商品库存列表
static ArrayList<Good> stock = new ArrayList<>();
public static void main(String[] args) {
initStock(); //库存商品初始化
showStock(); //显示库存信息
intoStock(); //商品入库操作
}
/**
* 库存商品信息初始化
*/
private static void initStock() {
stock.add(new Good(1001, "百事可乐", "张三", 4.5, 100));
stock.add(new Good(1002, "可口可乐", "李四", 4.0, 100));
stock.add(new Good(1003, "百事雪碧", "张三", 3.8, 100));
//补充若干行代码
}
/**
* 打印库存的商品信息
*/
private static void showStock() {
System.out.println("库存商品信息如下:");
Iterator<Good> it = stock.iterator();
while (it.hasNext()) {
Good good = it.next();
System.out.println(good.toString());
}
// 补充若干行代码
}
/**
* 商品入库操作
*/
private static void intoStock() {
while (true) {
int goodId;
Scanner scanner = new Scanner(System.in);
System.out.println("商品入库,请输入商品编号:");
goodId = scanner.nextInt();
//补充若干行代码
Good stockGood = getGoodById(goodId);
if (stockGood != null) {
// 判断是否存在此商品
int orderAmount;
System.out.println("请输入进货数量:");
orderAmount = scanner.nextInt();
//补充若干行代码
// 将入库商品信息封装成 Good 类对象 addGood
Good addGood = new Good(stockGood.getId(), stockGood.getName(), stockGood.getPeople(), stockGood.getPrice(),orderAmount);
FileUtil.saveGood(addGood);// 将本条数据保存至本地文件
// 更新库存中该商品数量=原数量+进货数量
stockGood.setAmount(stockGood.getAmount()+orderAmount);
stockGood.setTotalPrice(stockGood.getAmount()* stockGood.getPrice());
// 补充代码
// 显示库存信息
System.out.println("------该商品当前库存信息------");
showStock();
} else {
System.out.println("商品编号输入错误!");
}
}
}
/**
* 根据输入的商品编号查找商品信息
* 循环遍历库存中商品信息,找到商品编号相等的取出该对象
*/
private static Good getGoodById(int goodId) {
Iterator<Good> it = stock.iterator();
while (it.hasNext()) {
Good good = it.next();
if(good.getId()==goodId){
return good;
}
}// 补充若干行代码
return null;
}
}
package cn.edu.gpnu.bank.cn.edu.gpnu.exp11;
import java.io.*;
import java.text.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class FileUtil {
/**
* 保存商品信息
*/
public static void saveGood(Good good) {
BufferedOutputStream bos = null; // 带缓冲的字节输出流
StringBuffer strBuff = new StringBuffer(); // 拼接输出的内容
Date date = new Date(); //获取当前时间
DateFormat dateFormat = new SimpleDateFormat("20211115"); //定义日期格式
String dateStr = dateFormat.format(date); //将日期转换为格式化字符串
String fileName = "d:\\进货记录表" + dateStr + ".csv"; // 保存的文件名
try {
//判断本地是否存在此文件
File file = new File(fileName);
if (!file.exists()) {
//不存在文件,采取新建新文件方式
file.createNewFile();
bos = new BufferedOutputStream(new FileOutputStream(fileName));
System.out.println("文件 " + fileName + " 不存在,已新建文件。");
// 将首行标题进行拼接
String[] fields = {
"商品编号", "商品名称", "购买数量", "单价", "总价", "联系人"}; // 创建表头
for (String s : fields) {
strBuff.append(s); //追加字段标题
strBuff.append(","); //追加字段分隔符
}
strBuff.append("\r\n"); //追加换行符
} else {
//存在文件,采取修改文件方式
bos = new BufferedOutputStream(new FileOutputStream(fileName,
true));//若文件存在,则追加
System.out.println("文件 " + fileName + " 已存在,将入库记录附加到文件后面。 ");
}
// 开始追加商品记录
strBuff.append(good.getId()).append(",");//先追加 id,再继续追加“,”分隔符
strBuff.append(good.getName()).append(",");
strBuff.append(good.getAmount()).append(",");
strBuff.append(good.getPrice()).append(",");
strBuff.append(good.getTotalPrice()).append(",");
strBuff.append(good.getPeople()).append(",");
//补充若干行代码
strBuff.append("\r\n"); //追加换行符
String str = strBuff.toString();//将 StringBuffer 对象转换为字符串
byte[] bytes = str.getBytes("GBK");//以 GBK 字符编码方式将字符串
bos.write(bytes);
//补充代码,将 bytes 数组的全部内容保存到 bos 流。
System.out.println("成功保存入库信息。");
} catch (Exception e) {
//捕获各种异常
e.printStackTrace();
System.out.println("保存入库信息失败。");
} finally {
try {
if (bos != null)
bos.close();
} catch (Exception e2) {
e2.printStackTrace();
}
//补充代码,安全地关闭 bos 流。
}
}
}
【运行结果】