The n-queens puzzle is the problem of placing n queens on an n×n chessboard such that no two queens attack each other.
Given an integer n, return all distinct solutions to the n-queens puzzle.
Each solution contains a distinct board configuration of the n-queens' placement, where 'Q'
and '.'
both indicate a queen and an empty space respectively.
Example:
Input: 4 Output: [ [".Q..", // Solution 1 "...Q", "Q...", "..Q."], ["..Q.", // Solution 2 "Q...", "...Q", ".Q.."] ] Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above.这道题我参考了https://blog.csdn.net/u013564276/article/details/51244581这位博主的思想,用一维数组来存储皇后的位置。具体算法的解释可以看原博主,介绍的很清楚,对我很有帮助。
import java.util.*;
public class Solution{
public List<List<String>> solveNQueens(int n) {
List<List<String>>res=new ArrayList<>();
int []queenplace=new int [n];
placeQueen(queenplace,0,n,res);
return res;
}
public void placeQueen(int []queenplace,int row,int n,List<List<String>> res)
{
for(int col=0;col<n;col++)
{
if(isValid(queenplace,row,col))
{
queenplace[row]=col;
placeQueen(queenplace,row+1,n,res);
}
}
if(row==n)
{
ArrayList<String>list=new ArrayList<>();
for(int i=0;i<n;i++)//控制行数
{
String str="";
for(int col=0;col<n;col++)
{
if(queenplace[i]==col)
str+="Q";
else
str+=".";
}
list.add(str);
}
res.add(list);
}
}
public static boolean isValid(int []queenplace,int row,int col)
{
for(int i=0;i<row;i++)
{
if(col==queenplace[i])
return false;
if(col==queenplace[i]+row-i)
return false;
if(col==queenplace[i]-row+i)
return false;
}
return true;
}
}
第52题就是计算一共有多少种n皇后问题方案,只要在原来的基础上把return res改成return res.size()即可。
当然第52题也可以用一种取巧的方法。
int totalNQueens(int n) {
int ans[] = {0, 1, 0, 0, 2, 10, 4, 40, 92, 352};
return ans[n];
}
1
import java.util.*;
扫描二维码关注公众号,回复:
2067971 查看本文章
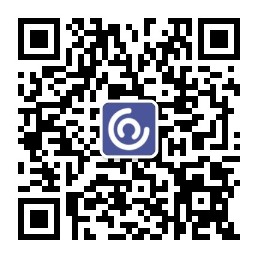