21.Max Consecutive Ones
Given a binary array, find the maximum number of consecutive 1s in this array.
给定一个二进制数组,找出由该数组中连续出现的数字一的最大个数。
示例:nums=[1,1,0,1,1,1],输出3
程序:
def fm(nums):
current = 0
max_n = 0
for num in nums:
if num == 1:
current += 1
max_n = max(max_n, current)
else:
current = 0
return current
22.K-diff Pairs in an Array
Given an array of integers and an integer K, you need to find the number of unique k-diff pairs in the array. Here a k-diff pair is defined as an integer pair (i, j), where i and j are both numbers in the array and their absolute difference is k.
给定一个整数数组和一个整数K,你需要在该数组中找出独一无二的k差异对。K差异对由整数对(i, j)组成,i与j都是数组中的数字,并且它们的绝对值差值等于K。
示例:给定[3,1,4,1,5]与k=2,输出2(因为(1,3)与(3,5))
程序:
import collections
def ij(nums,k):
if k>0:
return len(set(nums) + set(num+k for num in nums))
elif k=0:
return sum(v>1 for v in collections.Counter(nums).values())/2
else:
return 0
23.Array Partition Ⅰ
Given an array of 2n integers, your task is to group these integers into n pairs of integer, say(a1, b1), (a2, b2),…, (an,bn) which makes sum of min(ai, bi) for all i from 1 to n as large as possible.
给定一个由2n个整数组成的数组,你的任务是把这些整数划分为n对,如(a1, b1), (a2, b2),…, (an,bn),最后使得min(ai,bi)的总和最大。
示例:
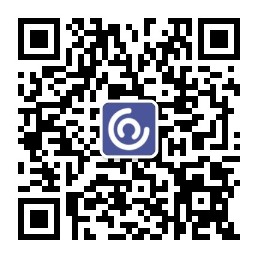
给定[1,4,3,2],输出4
程序:
def partition(nums):
return sum(sorted(nums)[::2])
24.Reshape the Matrix
In MATLAB, there is a very useful function called ‘reshape’, which can reshape a matrix into a new one with different size but keep its original data. You’re given a matrix represented by a two-dimensional array, and two positive integers r and c representing the row number and column number of the wanted reshaped matrix, respectively. The reshaped matrix need to be filled with all the elements of the original matrix in the same row-traversing order as they were. If the ‘reshape’ operation with given parameters is possible and legal, output the new reshaped matrix; Otherwise, output the original matrix.
在MATLAB中,有一个常用函数名叫reshape,该函数可以将矩阵重塑为大小不一的新矩阵且不改变原始数值。你需要将一个矩阵使用二维矩阵表示出来,两个正整数r和c分别代表重构矩阵的行数和列数。重构矩阵需要以相同的行遍历顺序遍历原矩阵中的所有元素。如果给定重构矩阵的参数可用且合法,输出新的重构矩阵;否则,输出原始矩阵。
示例:
输入 nums=[[1,2],[3,4]],r=1,c=4
输出 [[1,2,3,4]]
程序:
def reshape(nums, r, c):
if(len(nums)*len(nums[0]) == r*c):
mid = [nums[i][j] for i in range(len(nums)) for j in range(len(nums[0]))]
return [mid[t*c:(t+1)*c] for t in range(r)]
else:
return nums
25.Shortest Unsorted Continuous Subarray
Given an integer array, you need to find one continuous subarray that if you only sort this subarray in ascending order, then the whole array will be sorted in ascending order, too. You need to find the shorted such subarray and output its length.
给定一个整数数组,你需要找出一个连续的子数组,如果你仅采取升序排序对该子数组排序,整个原数组也会呈现升序状态。你需要找出最短的子数组,并且输出其长度。
示例:
输入 [2,6,4,8,10,9,15] 输出5
程序:
def reshape(nums, r, c):
if(len(nums)*len(nums[0]) == r*c):
mid = [nums[i][j] for i in range(len(nums)) for j in range(len(nums[0]))]
return [mid[t*c:(t+1)*c] for t in range(r)]
else:
return nums