http://acm.hdu.edu.cn/showproblem.php?pid=6168
Problem Description
zk has n numbers a1,a2,...,an. For each (i,j) satisfying 1≤i<j≤n, zk generates a new number (ai+aj). These new numbers could make up a new sequence b1,b2,...,bn(n−1)/2.
LsF wants to make some trouble. While zk is sleeping, Lsf mixed up sequence a and b with random order so that zk can't figure out which numbers were in a or b. "I'm angry!", says zk.
Can you help zk find out which n numbers were originally in a?
Input
Multiple test cases(not exceed 10).
For each test case:
∙The first line is an integer m(0≤m≤125250), indicating the total length of a and b. It's guaranteed m can be formed as n(n+1)/2.
∙The second line contains m numbers, indicating the mixed sequence of a and b.
Each ai is in [1,10^9]
Output
For each test case, output two lines.
The first line is an integer n, indicating the length of sequence a;
The second line should contain n space-seprated integers a1,a2,...,an(a1≤a2≤...≤an). These are numbers in sequence a.
It's guaranteed that there is only one solution for each case.
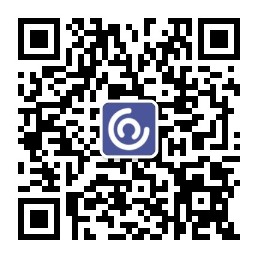
Sample Input
6 2 2 2 4 4 4 21 1 2 3 3 4 4 5 5 5 6 6 6 7 7 7 8 8 9 9 10 11
Sample Output
3 2 2 2 6 1 2 3 4 5 6
Source
2017 Multi-University Training Contest - Team 9
思路分析:
本题我们的思路的是首先求出a序列的元素的个数。然后来分析这个混合序列,通过简单的分析我们可以知道,本序列的最小的两个元素一定是a序列的成员(最小的两个无法由其他元素相加得到),找到这两个数并去掉之后,再在混合序列中去掉这两个数产生的和,通过分析我们可以得知此时的序列中的数又是a序列中的数(与上同理此数必然不可能通过其他的数相加得到)。然后再在混合序列中删除新找出的数和原来已经找出的的数的和,不断循环,直至找出来所有的数
了解到这些之后,我们选取multiset来动态维护(应该也可以使用priority_queue没试过)并使用multiset自带的lower_bound来查找应当删除的数(切不可直接删除值,由于存在多个,很容易产生错误)
代码:
#include<algorithm>
#include<iostream>
#include<cstring>
#include<string>
#include<cstdio>
#include<vector>
#include<stack>
#include<cmath>
#include<queue>
#include<set>
#include<map>
using namespace std;
typedef long long LL;
multiset<LL> s;
multiset<LL>::iterator it;
const int size=125260;
int get_ans(int x)
{
return (sqrt(1+8*x)-1)/2;
}
int main()
{
int n;
while(cin>>n)
{
int i;
LL tem;
for(i=0;i<n;i++)
scanf("%lld",&tem),s.insert(tem);
int cnt=get_ans(n);
LL ans[505];
int num=0;
while(num!=cnt)
{
it=s.begin();
ans[num++]=*it;
s.erase(it);
for(i=0;i<num-1;i++)
{
LL temp=ans[num-1]+ans[i];
s.erase(s.lower_bound(temp));
}
}
cout<<num<<endl;
for(i=0;i<num;i++)
{
printf("%lld",ans[i]);
if(i!=num-1) printf(" ");
else printf("\n");
}
}
return 0;
}
这里有两个地方需要注意的,一是在查找处,使用find或者lower_bound都无妨,但是注意必须使用multiset内自带的这两个函数,而不能使用stl库里的。还有就是输入输出要使用scanf和printf而不能用cin,cout否则会超时。