想写一个守护进程,到github上找源码,发现基本都是比较过时的。其中比较典型的是,google已经修复了不少原来可以利用的漏洞,而且NotificationCompat.Builder也已经强制要求输入NotificationChannel的信息,所以这里你必须自己用NotificationManager 来创建NotificationChannel,并把ID传给Builder,后面有详细源码,不再细述。
这里给出的,是一个android上标准的前台进程。大致思路如下,
一般在后台运行的Service的系统优先级都比较低的,当系统出现内存不足情况时,会成为优先回收的对象。当希望Service可以一直保持运行状态时,就要提高Service的优先级,其中的一个办法就是使用前台Service。其相关函数:使用startForeground可把Service设置为前台Service。取消则使用stopForeground。
至于broadcast模式,严格来说只能说是同类唤醒,好比你安装了某公司的Aapp,在安装Bapp时,它会检查你有没有装Aapp,如果有启动Aapp,就会把她唤醒(这真是一个糟糕的做法,我们的手机,大部分情况下就是这么被拖死的)。
言归正传,先看效果图,其中显示Foreground那个,就是我们的进程。
再看源码,
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<Button
android:id="@+id/btn_white"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/service_white" />
<Button
android:id="@+id/btn_background_service"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/service_general" />
</LinearLayout>
MainActivity.java
package com.spacesoftwares.spacecapture;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import com.spacesoftwares.spacecapture.service.BackgroundService;
import com.spacesoftwares.spacecapture.service.GrayService;
import com.spacesoftwares.spacecapture.service.WhiteService;
public class MainActivity extends AppCompatActivity {
private Button mBtnWhite, mBtnGray, mBtnBlack, mBtnGeneral;
private final static String TAG = MainActivity.class.getSimpleName();
/**
* 黑色唤醒广播的action
*/
private final static String BLACK_WAKE_ACTION = "com.wake.black";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//View view = getWindow().getDecorView();
mBtnWhite = findViewById(R.id.btn_white);
mBtnGray = findViewById(R.id.btn_gray);
mBtnBlack = findViewById(R.id.btn_black);
mBtnGeneral = findViewById(R.id.btn_background_service);
setListener();
}
private void setListener(){
OnClick onClick = new OnClick();
mBtnWhite.setOnClickListener(onClick);
mBtnGray.setOnClickListener(onClick);
mBtnBlack.setOnClickListener(onClick);
mBtnGeneral.setOnClickListener(onClick);
}
private class OnClick implements View.OnClickListener{
@Override
public void onClick(View view) {
int viewId = view.getId();
if (viewId == R.id.btn_white) { // 正常的Service
Intent whiteIntent = new Intent(MainActivity.this, WhiteService.class);
startService(whiteIntent);
} else if (viewId == R.id.btn_background_service) {//普通的后台进程
Log.i(TAG, "MAIN: btn_background");
//Context context = getApplicationContext();
Intent bgIntent = new Intent(MainActivity.this, BackgroundService.class);
startService(bgIntent);
}
}
}
}
WhiteService.java
package com.spacesoftwares.spacecapture.service;
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.app.Service;
import android.content.Context;
import android.content.Intent;
import android.graphics.Color;
import android.os.Build;
import android.os.IBinder;
import android.provider.Settings;
import android.support.annotation.Nullable;
import android.support.v4.app.NotificationCompat;
import android.util.Log;
import com.spacesoftwares.spacecapture.MainActivity;
import com.spacesoftwares.spacecapture.R;
import java.io.IOException;
import java.nio.channels.Channel;
public class WhiteService extends Service {
private final static String TAG = WhiteService.class.getSimpleName();
private final static int FOREGROUND_ID = 1000;
public WhiteService(){}
@Nullable
@Override
public IBinder onBind(Intent intent) {
//return null;
throw new UnsupportedOperationException("Not yet implemented");
}
@Override
public void onCreate() {
Log.i(TAG, "WhiteService->onCreate");
super.onCreate();
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.i(TAG, "WhiteService->onStartCommand");
//NotificationManager manager = (NotificationManager)getSystemService(NOTIFICATION_SERVICE);
NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
String NOTIFICATION_CHANNEL_ID = "my_channel_id_01";
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel notificationChannel = new NotificationChannel(NOTIFICATION_CHANNEL_ID, "My Notifications", NotificationManager.IMPORTANCE_HIGH);
// Configure the notification channel.
notificationChannel.setDescription("Channel description");
notificationChannel.enableLights(true);
notificationChannel.setLightColor(Color.RED);
notificationChannel.setVibrationPattern(new long[]{0, 1000, 500, 1000});
notificationChannel.enableVibration(true);
notificationManager.createNotificationChannel(notificationChannel);
}
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, NOTIFICATION_CHANNEL_ID);
builder.setSmallIcon(R.mipmap.ic_launcher);
builder.setContentTitle("Foreground");
builder.setContentText("Text shown on Notification bar");
builder.setContentInfo("Content Info");
builder.setWhen(System.currentTimeMillis());
Intent activityIntent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 1, activityIntent, PendingIntent.FLAG_UPDATE_CURRENT);
builder.setContentIntent(pendingIntent);
Notification notification = builder.build();
startForeground(FOREGROUND_ID, notification);
return super.onStartCommand(intent, flags, startId);
}
@Override
public void onDestroy() {
Log.i(TAG, "WhiteService->onDestroy");
super.onDestroy();
}
}
backgroundService.java
扫描二维码关注公众号,回复:
2581219 查看本文章
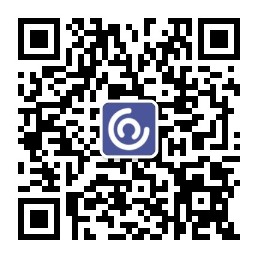
package com.spacesoftwares.spacecapture.service;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.support.annotation.Nullable;
import android.util.Log;
public class BackgroundService extends Service {
public BackgroundService(){
}
@Nullable
@Override
public IBinder onBind(Intent intent) {
//return null;
// TODO: Return the communication channel to the service.
throw new UnsupportedOperationException("Not yet implemented");
}
private final static String TAG = BackgroundService.class.getSimpleName();
@Override
public void onCreate() {
Log.i(TAG, "onCreate");
super.onCreate();
}
@Override
public void onDestroy() {
Log.i(TAG, "onDestroy");
super.onDestroy();
}
@Override
public int onStartCommand(Intent intent, int flags, final int startId) {
Log.i(TAG, "service start command, id: "+String.valueOf(startId));
new Thread(new Runnable() {
@Override
public void run() {
int i = 0;
Log.i(TAG, "SERVICE"+String.valueOf(startId)+" IS RUNNING for " + String.valueOf(i++) + " seconds");
try {
Thread.sleep(1000);
}catch (InterruptedException e){
e.printStackTrace();
}
stopSelf();
}
}).start();
return super.onStartCommand(intent, flags, startId);
}
}
最后,不要忘记在AndroidManifest.xml中添加service。
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.spacesoftwares.spacecapture">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service
android:name=".service.WhiteService"
android:enabled="true"
android:exported="false"
android:process=":white" />
<service
android:name=".service.BackgroundService"
android:enabled="true"
android:exported="true"
android:process=":bg"/>
</application>
</manifest>