G. Magic multisets
time limit per test
4 seconds
memory limit per test
256 megabytes
input
standard input
output
standard output
In the School of Magic in Dirtpolis a lot of interesting objects are studied on Computer Science lessons.
Consider, for example, the magic multiset. If you try to add an integer to it that is already presented in the multiset, each element in the multiset duplicates. For example, if you try to add the integer 22 to the multiset {1,2,3,3}{1,2,3,3}, you will get {1,1,2,2,3,3,3,3}{1,1,2,2,3,3,3,3}.
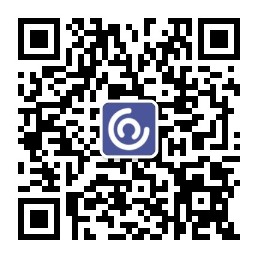
If you try to add an integer that is not presented in the multiset, it is simply added to it. For example, if you try to add the integer 44 to the multiset {1,2,3,3}{1,2,3,3}, you will get {1,2,3,3,4}{1,2,3,3,4}.
Also consider an array of nn initially empty magic multisets, enumerated from 11 to nn.
You are to answer qq queries of the form "add an integer xx to all multisets with indices l,l+1,…,rl,l+1,…,r" and "compute the sum of sizes of multisets with indices l,l+1,…,rl,l+1,…,r". The answers for the second type queries can be large, so print the answers modulo 998244353998244353.
Input
The first line contains two integers nn and qq (1≤n,q≤2⋅1051≤n,q≤2⋅105) — the number of magic multisets in the array and the number of queries, respectively.
The next qq lines describe queries, one per line. Each line starts with an integer tt (1≤t≤21≤t≤2) — the type of the query. If tt equals 11, it is followed by three integers ll, rr, xx (1≤l≤r≤n1≤l≤r≤n, 1≤x≤n1≤x≤n) meaning that you should add xx to all multisets with indices from ll to rrinclusive. If tt equals 22, it is followed by two integers ll, rr (1≤l≤r≤n1≤l≤r≤n) meaning that you should compute the sum of sizes of all multisets with indices from ll to rr inclusive.
Output
For each query of the second type print the sum of sizes of multisets on the given segment.
The answers can be large, so print them modulo 998244353998244353.
Examples
input
Copy
4 4 1 1 2 1 1 1 2 2 1 1 4 1 2 1 4
output
Copy
10
input
Copy
3 7 1 1 1 3 1 1 1 3 1 1 1 2 1 1 1 1 2 1 1 1 1 1 2 2 1 1
output
Copy
4 8
Note
In the first example after the first two queries the multisets are equal to [{1,2},{1,2},{},{}][{1,2},{1,2},{},{}], after the third query they are equal to [{1,1,2,2},{1,1,2,2},{1},{1}][{1,1,2,2},{1,1,2,2},{1},{1}].
In the second example the first multiset evolves as follows:
{}→{3}→{3,3}→{2,3,3}→{1,2,3,3}→{1,1,2,2,3,3,3,3}{}→{3}→{3,3}→{2,3,3}→{1,2,3,3}→{1,1,2,2,3,3,3,3}.
解题思路:先把N个集合的大小用一个线段树维护,这个线段树支持区间乘法,区间加法,区间求和。关键在于更新操作。什么时候区间要*2或+1,而且是哪些区间要*2或+1.
那么我们就要维护每一个集合内有哪些数,如果我们用主席树来做发现有点难度。那么就考虑暴力。
每次更新区间,我们都暴力查询每一个集合,看看是否存在那个数,这样子做明显不可取。
那么我们不妨用另外一种方式思考,我们用一个集合数组S[i],维护第i个数字在哪些区间出现过。
当我们有更新操作时,我们直接查询S[w]集合,然后判断这个集合里面的所有区间有哪些是跟查询的【L,R】相交的,相交的部分就用*2,不相交的部分就+1,这种做法看似优了很多,但是最坏复杂的还是很大。所以我们要高效的查询有哪些区间是相交的。所以S[w]集合不能保存区间,应该保存区间的端点,这样我们就可以用set自带的lower_bound操作,高效的更新区间和集合了。具体看代码的操作。
学习一下set维护区间。
#include <iostream>
#include <vector>
#include <string.h>
#include <algorithm>
#include <set>
#include <stdio.h>
#include <map>
#include <bitset>
using namespace std;
typedef unsigned long long ull;
typedef long long ll;
const int MAXN = 200005;
const ll MOD = 998244353;
ll lazyAdd[MAXN<<2];
ll sum[MAXN<<2];
ll lazyMul[MAXN<<2];
int N,Q;
void build(int l,int r,int rt){
lazyMul[rt]=1;
if(l==r)
return;
int m=(l+r)/2;
build(l,m,rt<<1);
build(m+1,r,rt<<1|1);
}
void pushup(int rt){
sum[rt]=(sum[rt<<1]+sum[rt<<1|1])%MOD;
}
void pushdown(int rt,int ln,int rn){
if(lazyMul[rt]!=1){
sum[rt<<1]=(sum[rt<<1]%MOD*lazyMul[rt]%MOD)%MOD;
lazyMul[rt<<1]=(lazyMul[rt<<1]%MOD*lazyMul[rt]%MOD)%MOD;
lazyAdd[rt<<1]=(lazyAdd[rt<<1]%MOD*lazyMul[rt]%MOD)%MOD;
sum[rt<<1|1]=(sum[rt<<1|1]%MOD*lazyMul[rt]%MOD)%MOD;
lazyMul[rt<<1|1]=(lazyMul[rt<<1|1]%MOD*lazyMul[rt]%MOD)%MOD;
lazyAdd[rt<<1|1]=(lazyAdd[rt<<1|1]%MOD*lazyMul[rt]%MOD)%MOD;
lazyMul[rt]=1;
}
if(lazyAdd[rt]){
sum[rt<<1]=(sum[rt<<1]+lazyAdd[rt]*ln%MOD)%MOD;
lazyAdd[rt<<1]=(lazyAdd[rt<<1]+lazyAdd[rt]%MOD)%MOD;
sum[rt<<1|1]=(sum[rt<<1|1]+lazyAdd[rt]*rn%MOD)%MOD;
lazyAdd[rt<<1|1]=(lazyAdd[rt<<1|1]+lazyAdd[rt])%MOD;
lazyAdd[rt]=0;
}
}
void updateAdd(int L,int R, ll C,int l,int r,int rt){
if(L<=l&&r<=R){
lazyAdd[rt]=(lazyAdd[rt]+C)%MOD;
sum[rt]=(sum[rt]+C*(r-l+1)%MOD)%MOD;
return;
}
int m=(l+r)/2;
pushdown(rt,m-l+1,r-m);
if(L<=m)
updateAdd(L,R,C,l,m,rt<<1);
if(R>m)
updateAdd(L,R,C,m+1,r,rt<<1|1);
pushup(rt);
}
void updateMul(int L,int R,ll C,int l,int r,int rt){
if(L<=l&&r<=R){
lazyMul[rt]=(lazyMul[rt]*C)%MOD;
lazyAdd[rt]=(lazyAdd[rt]*C)%MOD;
sum[rt]=(sum[rt]*C)%MOD;
return;
}
int m=(l+r)/2;
pushdown(rt,m-l+1,r-m);
if(L<=m)
updateMul(L,R,C,l,m,rt<<1);
if(R>m)
updateMul(L,R,C,m+1,r,rt<<1|1);
pushup(rt);
}
ll query(int L,int R,int l,int r,int rt){
if(L<=l&&r<=R)
return sum[rt]%MOD;
int m=(l+r)/2;
pushdown(rt,m-l+1,r-m);
ll ans=0;
if(L<=m)
ans=(ans+query(L,R,l,m,rt<<1))%MOD;
if(R>m)
ans=(ans+query(L,R,m+1,r,rt<<1|1))%MOD;
return ans%MOD;
}
set<pair<int,bool> > S[MAXN];
/***集合查询区间***/
void update(int l,int r,int w){
auto &s=S[w];
pair<int,bool> node1=make_pair(l,0);
pair<int,bool> node2=make_pair(r,1);
while(l<=r){
auto it=s.lower_bound(make_pair(l,0));
if(it==s.end()){
updateAdd(l,r,1,1,N,1);
break;
}
if(it->second==1){
updateMul(l,min(it->first,r),2,1,N,1);
l=min(it->first,r)+1;
if(r>=it->first)
s.erase(it);
}
else{
if(l<=min(it->first-1,r))//有可能刚好搜到了l这个位置
updateAdd(l,min(it->first-1,r),1,1,N,1);
l=min(it->first-1,r)+1;
if(r>=it->first)
s.erase(it);
}
}
auto it=s.lower_bound(node1);
auto it2=s.lower_bound(node1);
if(it==s.begin()||(--it)->second)
s.insert(node1);
if(it2==s.end()||it2->second==0)
s.insert(node2);
}
int main()
{
scanf("%d%d",&N,&Q);
build(1,N,1);
int op,l,r,w;
for(int i=0;i<Q;i++){
scanf("%d",&op);
if(op==1)
{
scanf("%d%d%d",&l,&r,&w);
update(l,r,w);
}
else
{
scanf("%d%d",&l,&r);
printf("%lld\n",query(l,r,1,N,1)%MOD);
}
}
return 0;
}