bank.cpp
#include <iostream>
using namespace std;
//namespace {
void print (int money) {
cout << money << endl;
}
//}
// 农行名字空间
namespace abc {
int balance = 0;
void save (int money) {
balance += money;
}
void draw (int money) {
balance -= money;
}
}
namespace abc {
void salary (int money) {
balance += money;
}
void print (int money) {
cout << "农行:";
::print (money);
}
}
// 建行名字空间
namespace ccb {
int balance = 0;
void save (int money) {
balance += money;
}
void draw (int money) {
balance -= money;
}
void salary (int money) {
balance += money;
}
}
int main (void) {
using namespace abc; // 名字空间指令
save (5000);
cout << "农行:" << balance << endl;
draw (3000);
cout << "农行:" << balance << endl;
ccb::save (8000);
cout << "建行:" << ccb::balance << endl;
ccb::draw (5000);
cout << "建行:" << ccb::balance << endl;
using ccb::salary; // 名字空间声明
// using abc::salary;
salary (6000);
cout << "建行:" << ccb::balance << endl;
abc::print (abc::balance);
return 0;
}
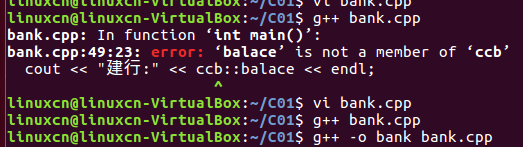
先进行编译,然后再取别名运行文件;
g++ bank.cpp
g++ -o bank bank.cpp
./bank
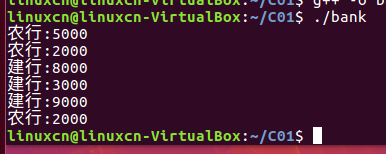
std:: 名字空间:
作用域限定运算符,相当于:“的”字符。
bool.cpp
#include <iostream>
using namespace std;
void print (bool sex) {
if (sex)
cout << "男生" << endl;
else
cout << "女生" << endl;
}
int main (void) {
bool b = true;
cout << sizeof (b) << endl;
cout << b << endl;
b = false;
cout << b << endl;
b = 3.14;
cout << b << endl;
char* p = NULL;
b = p;
cout << b << endl;
cout << boolalpha << b << endl;
bool sex;
sex = 3;
print (sex);
return 0;
}

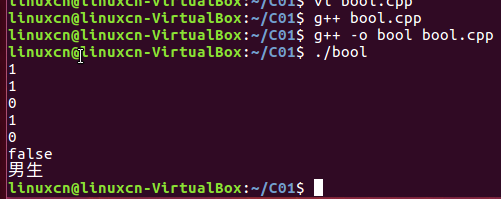
-----------------------------------------------------
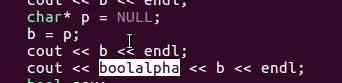
因为,char *p = null, b = p; 此时,boolalpha = false;
boolalpha以字符显示方式进行格式控制;
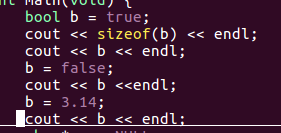
因为b是bool类型的,b = 3.14 , cout << b << endl; 后的值为1.
--------------------------------------------------
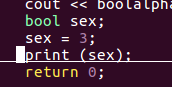
sex = 3 是bool类型的,猜测sex是True,男生”;
--------------------------------
验证:
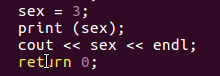
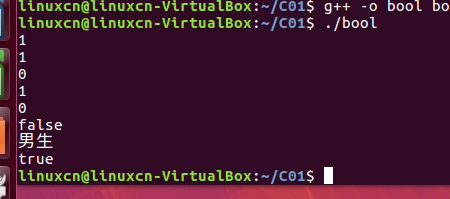
----------------------------
sizeof(空结构)-> 1
cfunc.c
void foo (int a) {}
defarg.cpp
#include <iostream>
using namespace std;
void foo (int a = 10, double b = 0.01,
const char* c = "tarena");
void foo (void) {}
void bar (int) {
cout << "bar(int)" << endl;
}
void bar (int, double) {
cout << "bar(int,double)" << endl;
}
int main (void) {
foo (1, 3.14, "hello");
foo (1, 3.14);
foo (1);
// foo (); // 歧义
bar (100);
bar (100, 12.34);
return 0;
}
void foo (int a /* = 10 */, double b /* = 0.01 */,
const char* c /* = "tarena" */) {
cout << a << ' ' << b << ' ' << c << endl;
}
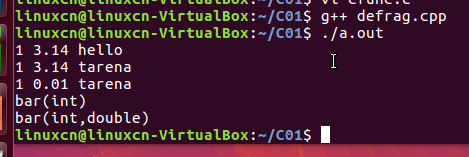
-----------------------
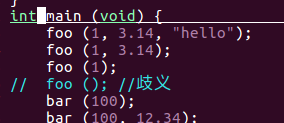
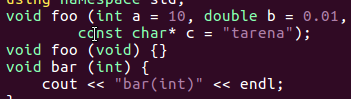
重载:在同一作用域中,函数名相同,参数表不同的函数,构成重载关系
int foo (int a, double b) {}
int foo (int b, double a)
函数名相同,参数名相同的不构成重载关系;
关注的是参数表的类型,不关心其名字;
重载解析:
(1)最优匹配原则;
(2)最小工作量原则;
函数名标识的是函数的地址,C++中函数名 可以重复,但是地址不一样。
const char * c= "tarena" 表示缺省值;缺省值一律靠右;
缺省参数放在声明语句当中 ,声明是给编译器看的;
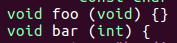
重载:需要用同一个函数(函数名是死的)来实现两个不同的功能;
v1 : void decode (int arg) {...}
v2: void decode (void) {}
---------------------------------------------
两个不同 函数用同一个名;
只有类型而没有名字的形参,渭之“哑元”。
占着位置而不起任何作用;
--------------------------------------------------
gcc -std=c++ 0x
使用2011标准去编译;
g++ 1.cpp -std=c++ 0x
--------------------------------------------
(1)如果调用一个函数时,没有提供实参,那么对应的形参就取缺省值;
(2)如果一个参数带有缺省值,那么它后面的所有参数都必须都带有缺省值;
(3)如果一个函数声明和定义分开,那么缺省参数只能放在声明中;
(4)避免和重载发生歧义;
(5)只有类型而没有名字的形参,谓之哑元;
enum.cpp
#include <iostream>
using namespace std;
int main (void) {
enum E {a, b, c};
E e;
e = a;
e = b;
e = c;
// e = 1000;
// e = 1;
return 0;
}
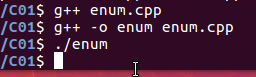
在这种 情况下,程序可以顺利进行编译;
hello.cpp
#include <iostream>
int main (void) {
std::cout << "Hello, World !" << std::endl;
int i;
double d;
char s[256];
// scanf ("%d%lf%s", &i, &d, s);
std::cin >> i >> d >> s;
// printf ("%d %lf %s\n", i, d, s);
std::cout << i << ' ' << d << ' ' << s << '\n';
return 0;
}

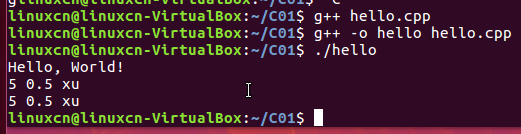
输出: cout - 标准输出对象;(控制台输出对象)
输入: cin - 标准输入对象;
插入运算符: << (把字符串的字面值插入到cout内)
提取运算符: >>
std::cout
(控制台输出对象) << "Hello,world !" <<(多次插入) std::endl;(换行\n)
math.cpp
extern "C" {
int add (int a, int b) {
return a + b;
}
int sub (int a, int b) {
return a - b;
}
}
/*
extern "C" int add (int a, int b, int c) {
return a + b + c;
}
*/
calc.c3
#include <stdio.h>
int add (int, int);
int main (void) {
printf ("%d\n", add (10, 20));
return 0;
}
nsover.cpp
#include <iostream>
using namespace std;
namespace ns1 {
int foo (int a) {
cout << "ns1::foo(int)" << endl;
return a;
}
};
namespace ns2 {
double foo (double a) {
cout << "ns2::foo(double)" << endl;
return a;
}
};
int main (void) {
using namespace ns1;
using namespace ns2;
cout << foo (10) << endl;
cout << foo (1.23) << endl;
using ns1::foo;
cout << foo (10) << endl;
cout << foo (1.23) << endl;
using ns2::foo;
cout << foo (10) << endl;
cout << foo (1.23) << endl;
return 0;
}
using ns1::foo;
ns1中的foo被引入到作用域中了;
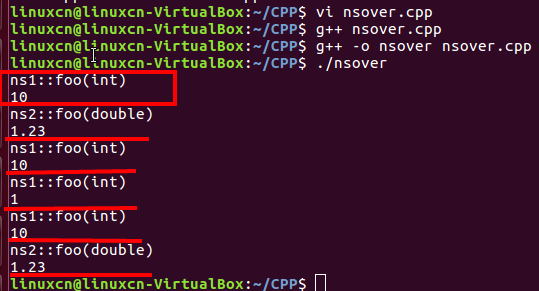
overload.cpp
#include <iostream>
using namespace std;
void foo (int a) {
cout << "foo(int)" << endl;
}
void bar (int a) {}
//int foo (int a) {}
int foo (int a, double b) {
cout << "foo(int,double)" << endl;
}
int foo (double a, int b) {
cout << "foo(double,int)" << endl;
}
//int foo (int b, double a) {}
int main (void) {
foo (100);
foo (100, 1.23);
foo (1.23, 100);
// foo (100, 100);
return 0;
}
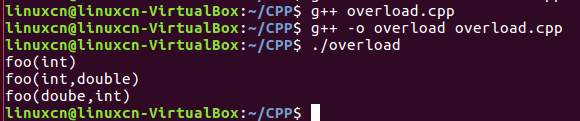
struct.c
#include <stdio.h>
int main (void) {
struct A {};
printf ("%d\n", sizeof (struct A));
struct A a;
struct A* pa = &a;
printf ("%p\n", pa);
return 0;
}
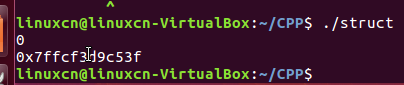
C语言中的空结构大小为0;
struct.cpp
#include <iostream>
using namespace std;
struct Student {
char name[128];
int age;
void who (void) {
cout << "我叫" << name << ",今年" << age
<< "岁了。" << endl;
}
};
int main (void) {
Student student = {"张飞", 25}, *ps = &student;
student.who ();
ps->who ();
struct A {};
cout << sizeof (A) << endl;
return 0;
}
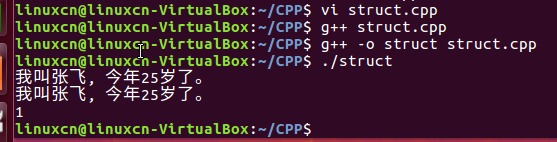
C++中类和结构没有区别;
sizeof (空结构) -> 1;
union.cpp
#include <iostream>
using namespace std;
int main (void) {
// 匿名联合
union {
int x;
char c[4] /*= {'A', 'B', 'C', 'D'}*/;
};
cout << (void*)&x << ' ' << (void*)c << endl;
x = 0x12345678;
for (int i = 0; i < 4; ++i)
cout << hex << (int)c[i] << ' ';
cout << endl;
return 0;
}
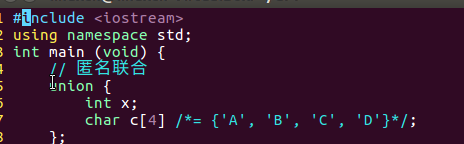
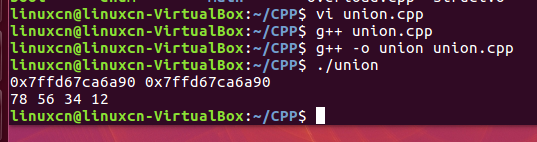

gcc -std=c++0x 使用2011编译器去编译;-2011标准;