版权声明:独学而无友,则孤陋寡闻。q群582951247 https://blog.csdn.net/mp624183768/article/details/85599104
选自-----------------
修改Main.dart
import 'package:flutter/material.dart';
import 'package:nihao_flutter/demo/navigator_demo.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
debugShowCheckedModeBanner: false,
home: NavigatorDemo(),
routes: {
'/about':(context)=>Page(title: 'About'),
'/home':(context)=>Page(title: 'Home'),
},
theme: ThemeData(
primarySwatch: Colors.yellow,
highlightColor: Color.fromRGBO(255, 255, 255, 0.5),
splashColor: Colors.white70),
);
}
}
import 'package:flutter/material.dart';
class NavigatorDemo extends StatelessWidget{
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
body: Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
FlatButton(
child: Text('Home'),
onPressed: (){
Navigator.pushNamed(context, '/home');
},
),
FlatButton(
child: Text('About'),
onPressed: (){
Navigator.of(context).push(
MaterialPageRoute(builder: (BuildContext context) {
return Page(title:"About");
}
)
);
},
)
],
),
),
);
}
}
class Page extends StatelessWidget {
final String title;
Page({
this.title
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
elevation: 0.0,
),
floatingActionButton: FlatButton(
child: Icon(Icons.arrow_back),
onPressed: (){
Navigator.pop(context);
},
),
);
}
}
Push 和pop 是进入和退出页面 也可以主动调用,除了这种方式写路由还有一种是使用initialRoute
方式如下
修改main.dart
import 'package:flutter/material.dart';
import 'package:nihao_flutter/demo/navigator_demo.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
debugShowCheckedModeBanner: false,
// home: NavigatorDemo(),
initialRoute: '/',
routes: {
'/':(context)=>NavigatorDemo(),
'/about':(context)=>Page(title: 'About'),
'/home':(context)=>Page(title: 'Home'),
},
theme: ThemeData(
primarySwatch: Colors.yellow,
highlightColor: Color.fromRGBO(255, 255, 255, 0.5),
splashColor: Colors.white70),
);
}
}
'/':(context)=>NavigatorDemo(),
表示初始路由对应的文件。
initialRoute: '/',
初始路由,代表根路由对应的路径
也就是说 如果initiaRoute 换成初始值/about
扫描二维码关注公众号,回复:
4812600 查看本文章
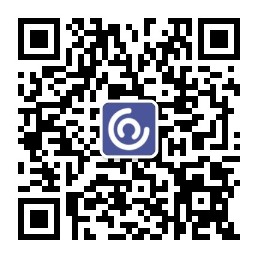
initialRoute: '/about',
routes: {
'/':(context)=>NavigatorDemo(),
'/about':(context)=>Page(title: 'About'),
'/home':(context)=>Page(title: 'Home'),
},
那么 刚进入主页就会先显示about页面
so 我们根据这一特性就会知道。这么写可以直接路由到主页
import 'package:flutter/material.dart';
import 'package:nihao_flutter/demo/navigator_demo.dart';
import 'package:nihao_flutter/demo/home_demo.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
debugShowCheckedModeBanner: false,
// home: NavigatorDemo(),
initialRoute: '/',
routes: {
'/':(context)=>HomeDemo(),
},
theme: ThemeData(
primarySwatch: Colors.yellow,
highlightColor: Color.fromRGBO(255, 255, 255, 0.5),
splashColor: Colors.white70),
);
}
}
之前我们写过一个listviewdemo 我们这次加上一个水波纹特效(溅墨)
precode
import 'package:flutter/material.dart';
import 'package:nihao_flutter/model/post.dart';
class ListViewDemo extends StatelessWidget{
Widget _listItemBuilder(BuildContext context,int index){
return Container(
color: Colors.white,
margin: EdgeInsets.all(8.0),
child: Column(
children: <Widget>[
Image.network(posts[index].imgeUrl),
SizedBox(height: 16.0),
Text(
posts[index].title,
style: Theme.of(context).textTheme.title,
),
Text(
posts[index].author,
style: Theme.of(context).textTheme.subhead,
),
SizedBox(height: 16.0),
],
),
);
}
@override
Widget build(BuildContext context) {
// TODO: implement build
return ListView.builder(
itemCount:posts.length,
itemBuilder: _listItemBuilder,
);
}
}
changedCode
import 'package:flutter/material.dart';
import 'package:nihao_flutter/model/post.dart';
class ListViewDemo extends StatelessWidget {
Widget _listItemBuilder(BuildContext context, int index) {
return Container(
color: Colors.white,
margin: EdgeInsets.all(8.0),
child: Stack(
children: <Widget>[
Column(
children: <Widget>[
AspectRatio(
aspectRatio: 16/9,
child: Image.network(posts[index].imgeUrl,fit: BoxFit.cover),
),
SizedBox(height: 16.0),
Text(
posts[index].title,
style: Theme.of(context).textTheme.title,
),
Text(
posts[index].author,
style: Theme.of(context).textTheme.subhead,
),
SizedBox(height: 16.0),
],
),
Positioned.fill(
child: Material(
color: Colors.transparent,
child: InkWell(
splashColor: Colors.white.withOpacity(0.3),
highlightColor: Colors.white.withOpacity(0.1),
onTap: (){
debugPrint('Tap');
},
),
),
)
],
));
}
@override
Widget build(BuildContext context) {
// TODO: implement build
return ListView.builder(
itemCount: posts.length,
itemBuilder: _listItemBuilder,
);
}
}
最后温习一下push ListView和详情页的交互
新建 post_show.dart 详情页面
import 'package:flutter/material.dart';
import '../model/post.dart';
class PostShow extends StatelessWidget {
final Post post;
PostShow({
@required this.post,
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('${post.title}'),
elevation: 0.0,
),
body: Column(
children: <Widget>[
Image.network(post.imgeUrl),
Container(
padding: EdgeInsets.all(32.0),
width: double.infinity,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
'${post.title}',
style: Theme.of(context).textTheme.title,
),
Text(
'${post.author}',
style: Theme.of(context).textTheme.subhead,
),
SizedBox(height: 32.0,),
Text(
'${post.description}',
style: Theme.of(context).textTheme.body1,
),
],
),
)
],
),
);
}
}
之前的post.dart 加上description属性
class Post {
const Post({
this.title,
this.author,
this.imgeUrl,
this.description,
});
final String title;
final String author;
final String imgeUrl;
final String description;
}
final List<Post> posts = [
Post(
title: '天天练123141',
author: 'Mohamed Chahin1',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '1',
),
Post(
title: '天天练',
author: 'Mohamed Chahin',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '2',
),
Post(
title: '天天练4124',
author: 'Mohamed Chahin',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '2',
),
Post(
title: '天天练123',
author: 'Mohamed Chahin',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '2',
),
Post(
title: '天天练123',
author: 'Mohamed Chahin',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '2',
),
Post(
title: '天天练12',
author: 'Mohamed Chahin',
imgeUrl: 'https://www.baidu.com/img/bd_logo1.png?where=super',
description: '2',
)
];
listview_demo.dart持续修改Inwell的ontap节点
onTap: (){
Navigator.of(context).push(
MaterialPageRoute(builder: (BuildContext context) {
return PostShow(post:posts[index]);
})
);
},
导入什么的我实在是 贴上把 ~~
import 'package:flutter/material.dart';
import 'package:nihao_flutter/demo/post_show.dart';
import 'package:nihao_flutter/model/post.dart';
效果我就不贴了 ~~自己去试试看吧