目录
扫描二维码关注公众号,回复:
5069390 查看本文章
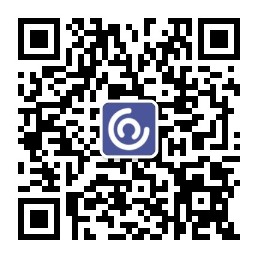
3.1 ByteArrayInputStream (字节数组输入流)
3.2 ByteArrayOutputStream (字节数组输出流)
一.流的分类
- 按方向
- 按功能
- 按数据类型
二.IO流四大抽象类
1.文件字节流
1.1 FileInputStream(文件字节输入流)
- 使用案例:读取文本中的数据打印到控制台
1.1.1 一次读取一个字节方式
最初版本
/**
* 第一个程序:理解操作步骤
* 1、创建源
* 2、选择流
* 3、操作
* 4、释放资源
*
*/
public class IOTest01 {
public static void main(String[] args) {
//1、创建源
File src = new File("abc.txt");
//2、选择流
try {
InputStream is =new FileInputStream(src);
//3、操作 (读取)
int data1 = is.read(); //第一个数据s
int data2 = is.read(); //第二个数据x
int data3 = is.read(); //第三个数据t
int data4 = is.read(); //????不是数据,文件的末尾返回-1
System.out.println((char)data1);
System.out.println((char)data2);
System.out.println((char)data3);
System.out.println(data4);
//4、释放资源
is.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
版本升级
//1、创建源
File src = new File("abc.txt");
//2、选择流
InputStream is =null;
try {
is =new FileInputStream(src);
//3、操作 (读取)
int temp ;
while((temp=is.read())!=-1) {
System.out.println((char)temp);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
1.1.2 一次读取一个字节数组
/**
* 四个步骤: 分段读取 文件字节输入流
* 1、创建源
* 2、选择流
* 3、操作
* 4、释放资源
*
* @author 裴新
*
*/
//1、创建源
File src = new File("abc.txt");
//2、选择流
InputStream is =null;
try {
is =new FileInputStream(src);
//3、操作 (分段读取)
byte[] flush = new byte[1024*10]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
//字节数组-->字符串 (解码)
String str = new String(flush,0,len);
System.out.println(str);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
1.2 FileOutputStream(文件字节输出流)
案例:将字符串以字节流的方式输出到文件中
/**
* 文件字节输出流
*1、创建源
*2、选择流
*3、操作(写出内容)
*4、释放资源
*
*/
//1、创建源
File dest = new File("dest.txt");
//2、选择流
OutputStream os =null;
try {
os = new FileOutputStream(dest,true);
//3、操作(写出)
String msg ="IO is so easy\r\n";
byte[] datas =msg.getBytes(); // 字符串-->字节数组(编码)
os.write(datas,0,datas.length);
os.flush();
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally{
//4、释放资源
try {
if (null != os) {
os.close();
}
} catch (Exception e) {
}
}
1.3 利用文件字节流进行文件拷贝
public static void copy(String srcPath,String destPath) {
//1、创建源
File src = new File(srcPath); //源头
File dest = new File(destPath);//目的地
//2、选择流
InputStream is =null;
OutputStream os =null;
try {
is =new FileInputStream(src);
os = new FileOutputStream(dest);
//3、操作 (分段读取)
byte[] flush = new byte[1024]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
os.write(flush,0,len); //分段写出
}
os.flush();
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally{
//4、释放资源 分别关闭 先打开的后关闭
try {
if (null != os) {
os.close();
}
} catch (IOException e) {
e.printStackTrace();
}
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
2.文件字符流
2.1 FileReader (文件字符输入流)
案例:使用文件字符输入流读取文件数据打印到控制台
/**
* 四个步骤: 分段读取 文件字符输入流
* 1、创建源
* 2、选择流
* 3、操作
* 4、释放资源
*
*
*/
//1、创建源
File src = new File("abc.txt");
//2、选择流
Reader reader =null;
try {
reader =new FileReader(src);
//3、操作 (分段读取)
char[] flush = new char[1024]; //缓冲容器
int len = -1; //接收长度
while((len=reader.read(flush))!=-1) {
//字符数组-->字符串
String str = new String(flush,0,len);
System.out.println(str);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if(null!=reader) {
reader.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
2.2 FileWriter (文件字符输出流)
案例:将字符串输出到文件中
/**
* 文件字符输出流
*1、创建源
*2、选择流
*3、操作(写出内容)
*4、释放资源
*
*/
//1、创建源
File dest = new File("dest.txt");
//2、选择流
Writer writer =null;
try {
writer = new FileWriter(dest);
//3、操作(写出)
//写法一
// String msg ="IO is so easy\r\n北京欢迎你";
// char[] datas =msg.toCharArray(); // 字符串-->字符数组
// writer.write(datas,0,datas.length);
//写法二
/*String msg ="IO is so easy\r\n北京欢迎你";
writer.write(msg);
writer.write("add");
writer.flush();*/
//写法三
writer.append("IO is so easy\r\n").append("北京欢迎你");
writer.flush();
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally{
//4、释放资源
try {
if (null != writer) {
writer.close();
}
} catch (Exception e) {
}
}
3.字节数组流
3.1 ByteArrayInputStream (字节数组输入流)
案例:读取字节数组中的内容打印到控制台
/**
* 四个步骤:字节数组输入流
* 1、创建源 : 字节数组 不要太大
* 2、选择流
* 3、操作
* 4、释放资源: 可以不用处理
*
*
*/
//1、创建源
byte[] src = "talk is cheap show me the code".getBytes();
//2、选择流
InputStream is =null;
try {
is =new ByteArrayInputStream(src);
//3、操作 (分段读取)
byte[] flush = new byte[5]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
//字节数组-->字符串 (解码)
String str = new String(flush,0,len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
3.2 ByteArrayOutputStream (字节数组输出流)
案例:将字符串转化成字节数组并转入目标数组
/**
* 字节数组输出流 ByteArrayOutputStream
*1、创建源 : 内部维护
*2、选择流 : 不关联源
*3、操作(写出内容)
*4、释放资源 :可以不用
*
* 获取数据: toByteArray()
*
*/
//1、创建源
byte[] dest =null;
//2、选择流 (新增方法)
ByteArrayOutputStream baos =null;
try {
baos = new ByteArrayOutputStream();
//3、操作(写出)
String msg ="show me the code";
byte[] datas =msg.getBytes(); // 字符串-->字节数组(编码)
baos.write(datas,0,datas.length);
baos.flush();
//获取数据
dest = baos.toByteArray();
System.out.println(dest.length +"-->"+new String(dest,0,baos.size()));
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally{
//4、释放资源
try {
if (null != baos) {
baos.close();
}
} catch (Exception e) {
}
}
3.3 图片读取到字节数组
/**
* 1、图片读取到字节数组
* 1)、图片到程序 FileInputStream
* 2)、程序到字节数组 ByteArrayOutputStream
*/
public static byte[] fileToByteArray(String filePath) {
//1、创建源与目的地
File src = new File(filePath);
byte[] dest =null;
//2、选择流
InputStream is =null;
ByteArrayOutputStream baos =null;
try {
is =new FileInputStream(src);
baos = new ByteArrayOutputStream();
//3、操作 (分段读取)
byte[] flush = new byte[1024*10]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
baos.write(flush,0,len); //写出到字节数组中
}
baos.flush();
return baos.toByteArray();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
3.4 字节数组写出到图片
/**
* 2、字节数组写出到图片
* 1)、字节数组到程序 ByteArrayInputStream
* 2)、程序到文件 FileOutputStream
*/
public static void byteArrayToFile(byte[] src,String filePath) {
//1、创建源
File dest = new File(filePath);
//2、选择流
InputStream is =null;
OutputStream os =null;
try {
is =new ByteArrayInputStream(src);
os = new FileOutputStream(dest);
//3、操作 (分段读取)
byte[] flush = new byte[5]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
os.write(flush,0,len); //写出到文件
}
os.flush();
} catch (IOException e) {
e.printStackTrace();
}finally {
//4、释放资源
try {
if (null != os) {
os.close();
}
} catch (Exception e) {
}
}
}
4.拷贝文件工具类
4.1 最初版本
/**
* 对接输入输出流
* @param is
* @param os
*/
public static void copy(InputStream is,OutputStream os) {
try {
//3、操作 (分段读取)
byte[] flush = new byte[1024]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
os.write(flush,0,len); //分段写出
}
os.flush();
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}finally{
//4、释放资源 分别关闭 先打开的后关闭
close(is,os);
}
}
/**
* 释放资源 版本1
* @param is
* @param os
*/
public static void close(InputStream is ,OutputStream os) {
try {
if (null != os) {
os.close();
}
} catch (IOException e) {
e.printStackTrace();
}
try {
if(null!=is) {
is.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 释放资源 版本2(建议)
* @param ios
*/
public static void close(Closeable... ios) {
for(Closeable io:ios) {
try {
if(null!=io) {
io.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
4.2 升级版本(jdk1.7可用)
/**
* 对接输入输出流
* try ...with...resource
* @param is
* @param os
*/
public static void copy(InputStream is,OutputStream os) {
try(is;os) {
//3、操作 (分段读取)
byte[] flush = new byte[1024]; //缓冲容器
int len = -1; //接收长度
while((len=is.read(flush))!=-1) {
os.write(flush,0,len); //分段写出
}
os.flush();
}catch(FileNotFoundException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}
}