Spring MVC基础之密码处理
1、使用IDEA创建项目如下:
2、在包my.controller
下新建控制器类UserController.java
UserController.java
package my.controller;
import my.model.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.portlet.ModelAndView;
@Controller
public class UserController {
//映射到初始页面:index.jsp
@RequestMapping(value = "index",method = RequestMethod.GET)
public ModelAndView indexPage(@ModelAttribute("user")User user){
return new ModelAndView("index","user",new User()); //ModelAndView("页面","模型名称",模型类);
}
//映射到结果页面:result.jsp
@RequestMapping(value = "login",method = RequestMethod.POST)
public String result(@ModelAttribute("user")User user, ModelMap modelMap){
modelMap.addAttribute("username",user.getUsername());
modelMap.addAttribute("password",user.getPassword());
return "/pages/result";
}
//映射到静态页面:staticPage.html
@RequestMapping(value = "redirect",method = RequestMethod.GET)
public String redirect(){
return "redirect:/staticPages/staticPage.html";
}
}
3、在my.model
下新建实例类User.java
User.java
package my.model;
public class User {
private String username;
private String password;
//构造方法自己补全(无参构造和带参构造)
//setter()和getter()方法自己补全
//toString()方法自己补全
}
4、前端页面:
result.jsp
//result.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>结果</title>
</head>
<body>
<p>
<h4>学生信息如下</h4>
<hr>
姓名:${username}
密码:${password}
</p>
<form action="/day0829/redirect">
<input type="submit" value="转到静态页面" >
</form>
</body>
</html>
index.jsp
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录</title>
</head>
<body>
<%--@elvariable id="user" type="my.model.User"--%>
<form:form action="/day0829/login" commandName="user" modelAttribute="user">
姓名:<form:input path="username"/>
密码:<form:password path="password"/>
<input type="submit" value="登录">
</form:form>
</body>
</html>
staticPage.html (静态页面)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
静态页面
</body>
</html>
5、xml文件配置
- dispatcher-servlet.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:p="http://www.springframework.org/schema/p" xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<context:component-scan base-package="my.controller"/>
<!--映射动态页面-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver" p:prefix="/" p:suffix=".jsp"/>
<!--开启对mvc的支持-->
<mvc:annotation-driven/>
<!--静态资源路径-->
<mvc:resources mapping="/staticPages/**" location="/staticPages/" />
</beans>
- web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/applicationContext.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!--编码过滤器-->
<filter>
<filter-name>characterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>characterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
6、运行
填写信息点击登录按钮:
点击按钮转到静态资源页面:
扫描二维码关注公众号,回复:
8504119 查看本文章
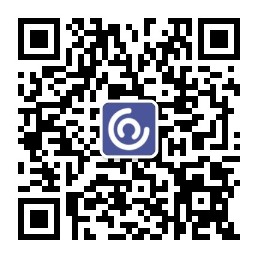
- 这里使用
<form:password path="password"/>
替代了传统的<input type="password" name="password" />
进行密码框的输入操作,其实该标签(<form:password path="password"/>
)会在页面生成<input type="password" name="password" />
然后实现密码框的处理操作… - 至于静态资源页面,只用于复习前面学习的静态页面内容…