JAVA运行错误处理机制
基本写法
try{
语句组
}catch(Exception ex){
异常处理语句
}
public static void main(String[] args)
{
try{
BufferedReader in=new BufferedReader(
new InputStreamReader(System.in));
System.out.print("Please input a number:");
String s=in.readLine();
int n=Integer.parseInt(s);
}catch(IOException ex){
ex.printStackTrace();
}catch(NumberFormatException ex){
ex.printStackTrace();
}
}
Java中处理
抛出异常,运行时系统在调用栈中查找(从生成异常的方法开始进行回溯,知道找到),捕获异常的代码
相关语句
抛出异常 throw 异常对象;(一旦执行了throw语句 就不执行其后买你的语句了)
捕获异常
try{
语句组
}catch(异常类名 异常形式参数名){
异常处理语句组;
}catch(异常类名 异常形式参数名){
异常处理语句组;
}filally{
异常处理语句组;
}
其中catch语句有0个或至多个,可以没有finally语句
异常分类
Error:JVM错误
Exception :异常
一般所说的异常是Exception及其子类。
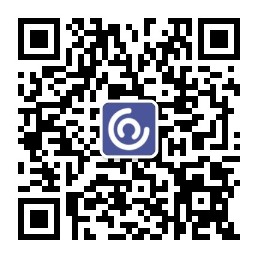
Exception类
构造方法
public Exception();
public Exception(String message);
Exception(String message,Throwable cause);
方法
getMessage()
getCause()
printStackTrace()
多异常处理 子异常要排在父异常的前面
finally:无论是否有异常都要执行 即使其中有break returnt等语句,编译时,finally生成了多遍
授检的异常
Exception分两分钟
RuntimeException及其子类,可以不明确处理
否则,称为,受检的异常
受检异常,要求明确进行语法处理
要么捕获(catch)
要么抛(throws):在方法的签名后面用throwsxxx声明
在子类中,如果要覆盖父类的一个方法,若父类中的方法声明了
throws异常,则子类的方法也可以throws异常
可以抛出子类异常(更具体的异常),但不能抛出一般的异常
//下面调用readFile()
public class ExceptionTrowsToOther(){
public static void main(String[] args){
try{
System.out.println("====Before====");
readFile();
System.out.println("====After====");
}catch(IOException e){System.out.println(e);}
}
}
//比如读写文件的时候,文件不存在
public static void readFile()throws IOException{
FileInputStream in=new FileInputStream("myfile.txt");
int b;
b=in.read();
while(b!=-1){
System.out.print((char)b);
b=in.read();
}
in.close();
}
有一种 try with resource
try(类名 变量名=new类型())
{
xxxxxx
}//自动添加了finally{变量.close();}不论是否出现异常,都会执行
static String ReadOneLline2(String path)
throws IOException
{
try(BufferedReader br=new BufferedReader(new FileReader(pat))){
return br.readLine();
}
}
//这样写避免了以前 打开文件 如果不为空,读取一行后还要关闭, 这样写编译器会自动关闭文件
自定义异常
自定义异常的时候 继承自Exception或某个子Exception类,定义属性和方法,或重载父类的方法
对于异常,除了捕获处理,有时还需要将此异常进一步传递给调用者,让它也感受到,这时,可以在catch语句块或finally语句块中使用下面三种方式
重抛异常 throw e
重新生成一个异常,抛出 throw new Exception("some message");
重新生成并抛出一个新异常,该异常包含了当前异常的信息。如:
throw new Exception("some message",e); 可用e.getCause()来得到内部异常
//假设是一个银行ATM系统,然后去核对一些账户余额
//这是个异常处理
public class ExceptionCause{
public static void main(String [] args){
try
{
BankATM.GetBalanceInfo(12345L);
}catch(Exception e){
System.out.println("something wrong: "+e);
System.out.println("cause: "+e.getCause());
}
}
}
class DataHouse{
public static void FindData(long ID)
throws DataHouseException
{
if(ID>0 && ID<1000)
System.out.println("id:"+ID);
else
throw new DataHouseException("cannot find the ID");
}
}//如果数据方面异常 抛出数据方面异常的信息
class BankATM{
public static void GetBalanceInfo(long ID)
throws MyAppException
{
try{
DataHouse.FindData(ID);
}catch(DataHouseException e){
throw new MyAppException("invalid id",e);
}
}
}
class DataHouseException extends Exception{
public DataHouseException(String message){
super(message);
}
}
class MyAppException extends Exception{
public MyAppException(String message){
super(message);
}
public MyAppException(String message,Exception cause){//构造函数 把它内部的原因和信息作为参数
super(message,cause);
}
}
断言assertion
assert格式:
assert表达式:
assert表达式:信息;
在调式程序时 如果表达式不为true 则程序会产生异常,并输出相关的错误信息
class Assertion{
public static void main(String[] args){
assert hypotenuse(3,4)==5:"算法不正确";
}
static double hypotenuse(double x,double y){
return Math.sqrt(x*x+y*y+1);
}
}//1.4以上才能用断言
程序的测试及JUnit
编写程序代码的同时,还编写测试代码来判断这些程序是否正确
这个过程称为“测试驱动”的开发过程
Java测试中,经常用到JUnit框架
在eclipse中new 新建Junit文件会生成一个模板
import static org.junit.Assert.*;
public class testHello1{
@Test //表明这是一个测试函数
public void testSum1(){
HelloWorld a=new HelloWorld();
assertEquals(a,sum(1,2),3);
assertEquals(a,sum(1,2),a.sum(2,1));
assertEquals(a,sum(0,100),100);
//fail("Not yet implemented")
}
}
assertEquals(参数1,参数2);//表示程序要两个参数相等
assertNull(参数1);//表示参数要 为null
fail(信息);表示程序出错了