练习1:(2)创建一个类,它包含一个int域和一个char域,它们都没有被初始化,将它们的值打印出来,以验证Java执行了默认初始化。
public class PrimitiveTest {
static int i;
static char ch;
public static void main(String[] args) {
System.out.println("int = " + i);
System.out.println("char= " + ch);
//输出
//int = 0
//char =
}
}
补充了其它类型默认值
\u0000代表的是null,一个控制字符,表示没有值,不可见,输出到控制台是空,但不是真正的空格
相关知识-其他数据类型默认值:[java中的数据类型] (https://blog.csdn.net/Laputa219/article/details/103209437)
练习2:(1)参照本章的HelloDate.java这个例子,创建一一个“Hello, World” 程序,该程序只要输出这句话即可。你所编写的类里只需-一个方法(即"main"方法,在程序启动时被执行)。
记住要把它设为static形式,并指定参数列表-即使根本不会用到这个列表。用javac进行编译,再用java运行它。如果你使用的是不同于JDK的开发环境,请了解如何在你的环境中进行编译和运行。
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
练习3:(1)找出含有ATypeName的代码段,将其改写成完整的程序,然后编译、运行。
public class ATNTest {
public static void main(String[] args) {
class ATypeName {
int i;
double d;
boolean b;
void show() {
System.out.println(i);
System.out.println(d);
System.out.println(b);
}
}
ATypeName a = new ATypeName();
a.i = 3;
a.d = 2.71828;
a.b = false;
a.show();
}
}
练习4:(1)将DataOnly代码段改写成一个程序,然后编译、运行。
public class DataOnlyTest {
public static void main(String[] args) {
class DataOnly {
int i;
double d;
boolean b;
void show() {
System.out.println(i);
System.out.println(d);
System.out.println(b);
}
}
DataOnly data = new DataOnly();
data.i = 3;
data.d = 2.71828;
data.b = false;
data.show();
}
}
练习5:(1)修改前一个练习,将DataOnly中的数据在main()方法中赋值并打印出来。
public class DataOnlyTest {
public static void main(String[] args) {
class DataOnly {
int i;
double d;
boolean b;
void show() {
System.out.println(i);
System.out.println(d);
System.out.println(b);
}
}
DataOnly data = new DataOnly();
data.i = 3;
data.d = 2.71828;
data.b = false;
data.show();
}
}
你没有看错,第四第五题是一样的。
练习6:(2)编写一个程序,让它含有本章所定义的storage()方法的代码段,并调用之。
public class Test6 {
public static void main(String[] args) {
class StoreStuff {
int storage(String s) {
return s.length() * 2;
}
}
StoreStuff x = new StoreStuff();
System.out.println(x.storage("hi"));
}
}
练习7:(1)将Incrementable的代码段改写成一个完整的可运行程序。
class StaticTest {
static int i = 47;
}
class Incrementable {
static void increment() { StaticTest.i++; }
}
public class ITest {
public static void main(String[] args) {
System.out.println("StaticTest.i= " + StaticTest.i);
StaticTest st1 = new StaticTest();
StaticTest st2 = new StaticTest();
//此处不符合代码规范,一般不这样使用,此处仅做测试使用;
//避免通过一个类的对象引用访问此类的静态变量或静态方法,
//无谓增加编译器解析成本,直接用类名来访问即可--<阿里java开发手册>
System.out.println("st1.i= " + st1.i);
System.out.println("st2.i= " + st2.i);
Incrementable sf = new Incrementable();
sf.increment();
System.out.println("After sf.increment() called: ");
System.out.println("st1.i = " + st1.i);
System.out.println("st2.i = " + st2.i);
Incrementable.increment();
System.out.println("After Incrementable.increment called: ");
System.out.println("st1.i = " + st1.i);
System.out.println("st2.i = " + st2.i);
}
}
输出结果:
StaticTest.i= 47
st1.i= 47
st2.i= 47
After sf.increment() called:
st1.i = 48
st2.i = 48
After Incrementable.increment called:
st1.i = 49
st2.i = 49
练习8:(3)编写一个程序,展示无论你创建了某个特定类的多少个对象,这个类中的某个特定的static域只有-一个实例。
class StaticTest {
static int i = 47;
}
class Incrementable {
static void increment() { StaticTest.i++; }
}
public class OneStaticTest {
public static void main(String[] args) {
System.out.println("StaticTest.i= " + StaticTest.i);
StaticTest st1 = new StaticTest();
StaticTest st2 = new StaticTest();
System.out.println("st1.i= " + st1.i);
System.out.println("st2.i= " + st2.i);
Incrementable.increment();
System.out.println("After Incrementable.increment() called: ");
System.out.println("st1.i = " + st1.i);
System.out.println("st2.i = " + st2.i);
Incrementable.increment();
System.out.println("After Incrementable.increment called: ");
System.out.println("st1.i = " + st1.i);
System.out.println("st2.i = " + st2.i);
st1.i = 3;
System.out.println("After st1.i = 3, ");
System.out.println("st1.i = " + st1.i);
System.out.println("st2.i = " + st2.i);
System.out.println("Create another StaticTest, st3.");
StaticTest st3 = new StaticTest();
System.out.println("st3.i = " + st3.i);
}
}
输出结果:
StaticTest.i= 47
st1.i= 47
st2.i= 47
After Incrementable.increment() called:
st1.i = 48
st2.i = 48
After Incrementable.increment called:
st1.i = 49
st2.i = 49
After st1.i = 3,
st1.i = 3
st2.i = 3
Create another StaticTest, st3.
st3.i = 3
练习9:(2) 编写一个程序,展示自动包装功能对所有的基本类型和包装器类型都起作用。
public class AutoboxTest {
public static void main(String[] args) {
boolean b = false;
char c = 'x';
byte t = 8;
short s = 16;
int i = 32;
long l = 64;
float f = 0.32f;
double d = 0.64;
Boolean B = b;
System.out.println("boolean b = " + b);
System.out.println("Boolean B = " + B);
Character C = c;
System.out.println("char c = " + c);
System.out.println("Character C = " + C);
Byte T = t;
System.out.println("byte t = " + t);
System.out.println("Byte T = " + T);
Short S = s;
System.out.println("short s = " + s);
System.out.println("Short S = " + S);
Integer I = i;
System.out.println("int i = " + i);
System.out.println("Integer I = " + I);
Long L = l;
System.out.println("long l = " + l);
System.out.println("Long L = " + L);
Float F = f;
System.out.println("float f = " + f);
System.out.println("Float F = " + F);
Double D = d;
System.out.println("double d = " + d);
System.out.println("Double D = " + D);
}
}
练习10:(2编写一个程序,打印出从命令行获得的三个参数。为此,需要确定命令行数组中String的下标。
public class CommandArgTest {
public static void main(String[] args) {
if(args.length < 3) {
System.err.println("Need 3 arguments");
System.exit(1);
}
System.out.println("args[0] = " + args[0]);
System.out.println("args[1] = " + args[1]);
System.out.println("args[2] = " + args[2]);
}
}
args用来储存命令行参数
练习11:(1)将AlITheColorsOfTheRainbow这个示例改写成-一个程序,然后编译、运行。
class AllTheColorsOfTheRainbow {
int anIntegerRepresentingColors = 0;
int hue = 0;
void changeTheHueOfTheColor(int newHue) {
hue = newHue;
}
int changeColor(int newColor) {
return anIntegerRepresentingColors = newColor;
}
}
public class Rainbow {
public static void main(String[] args) {
AllTheColorsOfTheRainbow atc = new AllTheColorsOfTheRainbow();
System.out.println("atc.anIntegerRepresentingColors = " + atc.anIntegerRepresentingColors);
atc.changeColor(7);
atc.changeTheHueOfTheColor(77);
System.out.println("After color change, atc.anIntegerRepresentingColors = " + atc.anIntegerRepresentingColors);
System.out.println("atc.hue = " + atc.hue);
}
}
练习12:(2)找出HelloDate.java的第二版本,也就是那个简单注释文档的示例。对该文件执行javadoc,然后通过Web浏览器观看运行结果。
import java.util.Date;
/** The first Thinking in Java example program.
* Displays a string and today's date.
* @author Burce Eckel
* @author www.MindView.net
* @version 4.0
*/
public class DocTest {
/** Entry poing to class and application.
* @param args array of string arguments
*/
public static void main(String[] args) {
System.out.println("Hello, it's: ");
System.out.println(new Date());
}
} /* Output: (55% match)
*/
javadoc是注释提取工具,输出是一个html文件
ps:可能是jdk版本问题,我用的是1.8,输出时会有两个异常,所以对源文件做了稍微修改。
命令:
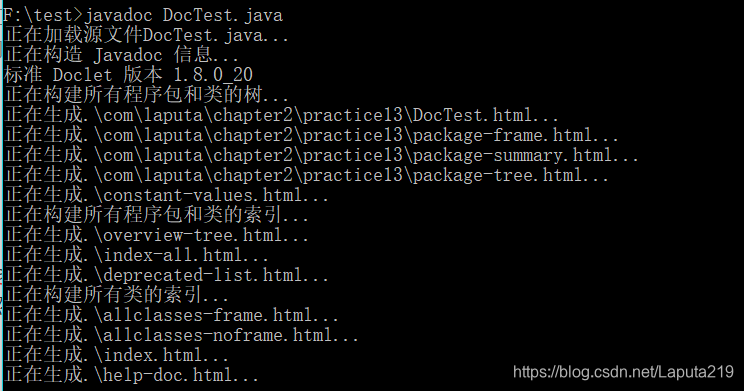
index.html
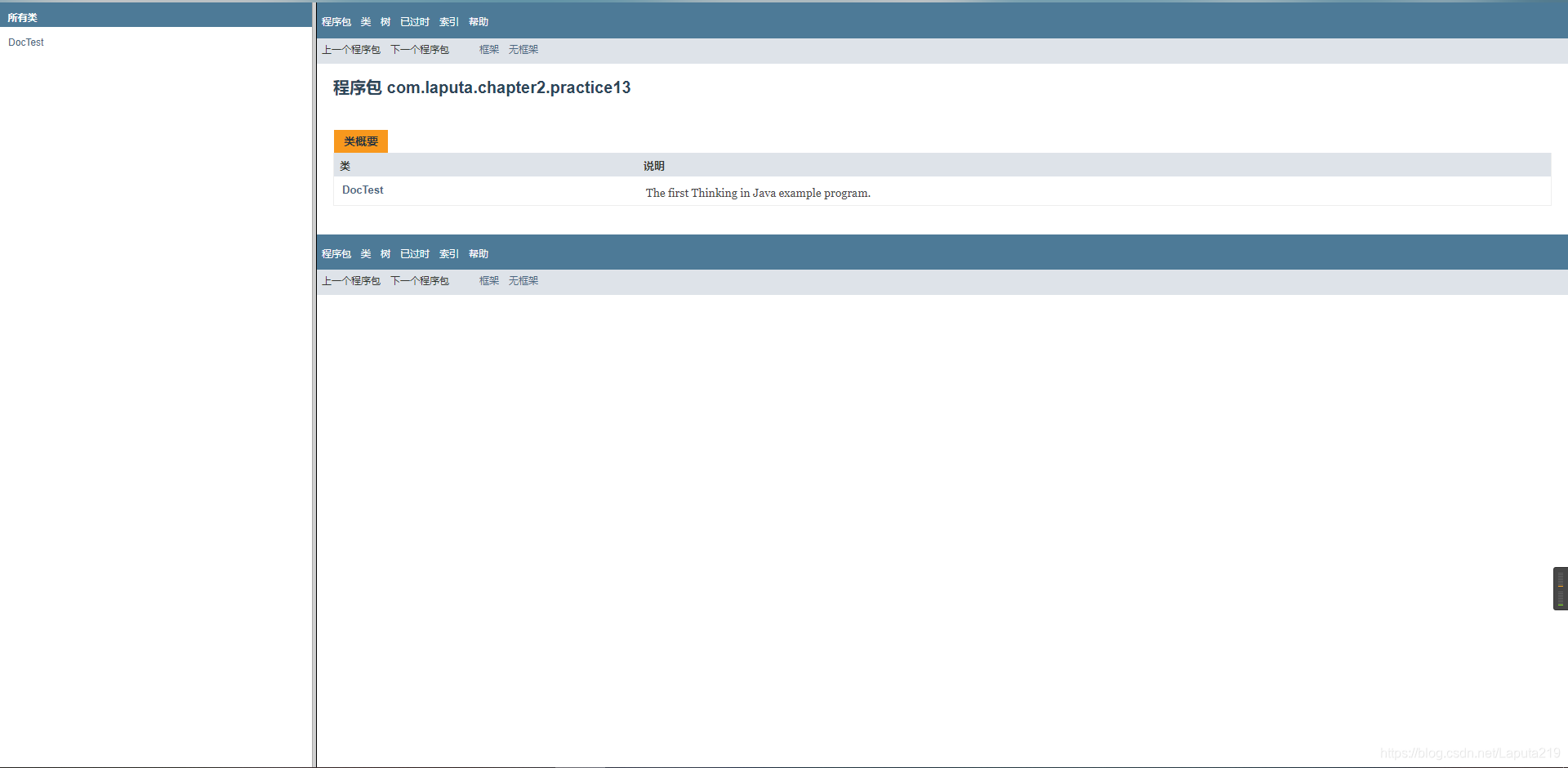
练习13:(1)通过Javadoc运行Documentation1.java,Documentation2.java和Documentation3.java,然后通过Web浏览器验证所产生的文档。
//: object/Documentation1.java
/** A class comment */
public class Documentation1 {
/** A field comment */
public int i;
/** A method comment */
public void f() {}
}///:~
/**
* <pre>
* Uses
* System.out.println(new Date());
* </pre>
*/
public class Documentation2 {
Date d = new Date();
void showDate() {
System.out.println("Date = " + d);
}
}
/**
* You can even insert a list:
* <ol>
* <li> Item one
* <li> Item two
* <li> Item three
* </ol>
*/
public class Documentation3 {
public static void main(String[] args) {
Date d = new Date();
System.out.println("d = " + d);
}
}
Documentation2
Documentation3
练习14: (1) 在前一个练习的文档中加入各项的HTML列表。
/**
* You can even insert a list:
* <ol>
* <li> Item one
* <li> Item two
* <li> Item three
* </ol>
* Another test list
* <ol>
* <li> One
* <li> Two
* <li> Three
* </ol>
*/
public class Documentation4 {
/** Let's try a public field list
* <ol>
* <li> One
* <li> Two
* <li> Three
* </ol>
*/
public int i = 2;
/**
* A private field list (-private to see)
* <ol>
* <li> One
* <li> Two
* <li> Three
* </ol>
*/
private int j = 3;
/**
* Another list can be inserted here to help explain the
* following method call
* <ol>
* <li> One
* <li> Two
* <li> Three
* </ol><br>
* but may be formatted differently in Method Summary
*/
public static void main(String[] args) {
/**
* Let's try another test list here
* <ol>
* <li> One
* <li> Two
* <li> Three
* </ol>
*/
Date d = new Date();
System.out.println("d = " + d);
}
}
练习15:(1)使用练习2的程序,加入注释文档。用javadoc提取此注释文档,并产生一个HTML文件,然后通过Web浏览器查看结果。
/**
* Public class contained in file of the same name that includes main()
*/
public class HelloDocTest {
/** main method executed by java
*/
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
练习16:(1)找到第5章中的Overloading.java示例,并为它加入javadoc文档。然后用javadoc提取此注释文档,并产生一个HTML文件,最后,通过Web浏览器查看结果。
/** creates type Tree wth two constructors and one info method
*/
class Tree {
int height;
/** no-argument constructor
* assigns height = 0
*/
Tree() {
System.out.println("Planting a seedling");
height = 0;
}
/** constructor taking an int argument,
* assigns height that int argument
*/
Tree(int initialHeight) {
height = initialHeight;
System.out.println("Creating new tree that is " + height + " feet tall");
}
/** method to print height of tree object
*/
void info() {
System.out.println("Tree is " + height + " feet tall");
}
/** overloaded method to print string argument
* and height of a tree object
*/
void info(String s) {
System.out.println(s + ": Tree is " + height + " feet tall");
}
}
/** class to test construction of tree objects
*/
public class Overloading {
public static void main(String[] args) {
for(int i = 0; i < 5; i++) {
Tree t = new Tree(i);
t.info();
t.info("overloading method");
}
// Overloaded constructor:
new Tree();
}
}
在原有答案基础上做了稍微修改和说明,希望对你有用。
----学海无涯,我需更加努力才能追上大佬的步伐。