1.1 Feign简介
Feign是简化Java HTTP客户端开发的工具(java-to-httpclient-binder),它的灵感来自于Retrofit、JAXRS-2.0和WebSocket。Feign的初衷是降低统一绑定Denominator到HTTP API的复杂度,不区分是否为restful
1.2 演示demo
(1)在上文创建的springCloudDemo_EurekaClient_A中创建com.xxx.controller包,并创建DemoCtroller类
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RequestMapping("/demo")
@CrossOrigin
@RestController
public class DemoController {
@Value("${server.port}")
private String serverPort;
@GetMapping("/getPort")
public String getPort(){
return "我来自client-A,我的端口是 : " + serverPort;
}
}
其中:@GetMapping为@RequestMapping(value = "xxx", method = RequestMethod.GET)的简写
@RestController注解相当于@ResponseBody + @Controller合在一起的作用
@CrossOrigin是用来处理跨域请求的注解
(2)运行启动类,在注册中心可以看到发布的服务
(3)修改application.yml中的port 并取消single instance only 启动多个服务
(4)创建服务springCloudDemo_eurekaServer_B,并引入相关依赖
扫描二维码关注公众号,回复:
9120128 查看本文章
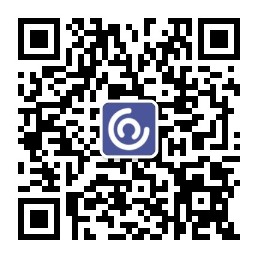
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
</dependencies>
(5)创建配置文件application.yml
eureka:
client:
service-url:
defaultZone: http://127.0.0.1:6868/eureka/
instance:
prefer-ip-address: true
server:
port: 7100
spring:
application:
name: clientb
(6)创建启动类
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
@SpringBootApplication
@EnableEurekaClient
@EnableDiscoveryClient
@EnableFeignClients
public class EurekaClientB {
public static void main(String[] args) {
SpringApplication.run(EurekaClientB.class,args);
}
}
(7)在springCloudDemo_eurekaServer_B模块下创建 com.xxx.client包,包下创建接口
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
@FeignClient("clienta")
public interface DemoClient {
@GetMapping("/demo/getPort")
public String getPort();
}
@FeignClient注解用于指定从哪个服务中调用功能 ,注意 里面的名称与被调用的服务名保持一致,并且不能包含下划线。
@GetMapping注解用于对被调用的微服务进行地址映射。注意 @PathVariable注解一定要指定参数名称,否则出错
(8)在com.xxx.contorller下创建DemoController
import com.hongyin.client.DemoClient;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RequestMapping("/demo")
@RestController
@CrossOrigin
public class DemoController {
@Autowired
private DemoClient demoClient;
@GetMapping("getPort")
public String getPort(){
return demoClient.getPort();
}
}
(9)启动springCloudDemo_eurekaClient_B测试:
可以看到我们通过微服务springCloudDemo_eurekaClient_B访问到了springCloudDemo_eurekaClient_A中的接口,完成了微服务之间的调用