准备工作
eclipse,
MySQL。
今天给大家带来一个外卖平台,以供学习使用。
新建GuLuLu项目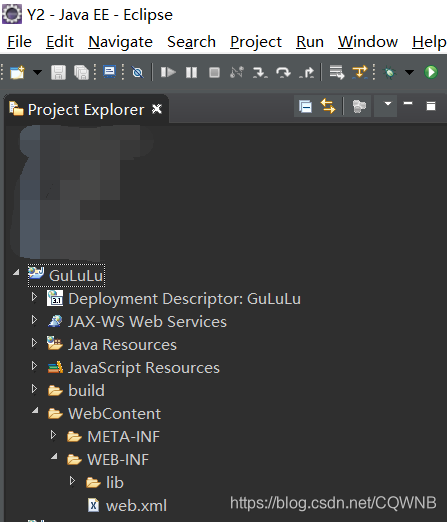
然后导入jar包。项目所需jar链接
配置web.xml文件,里面是配置springMVC核心控制器、延迟加载、加载applicationContext.xml等等
源码:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<!-- 加载application文件,会把spring大容器发布到application作用域 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext*.xml</param-value>
</context-param>
<listener>
<listener-class>
org.springframework.web.context.ContextLoaderListener
</listener-class>
</listener>
<!-- 延迟加载 -->
<filter>
<filter-name>opensession</filter-name>
<filter-class>
org.springframework.orm.hibernate4.support.OpenSessionInViewFilter
</filter-class>
</filter>
<filter-mapping>
<filter-name>opensession</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!-- utf-8编码 -->
<filter>
<filter-name>encoding</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encoding</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!-- 核心控制器 -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc-servlet.xml</param-value>
</init-param>
<!-- tomact加载完工程后,servletContext初始化完成后,马上创建核心控制器 -->
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<!-- 这里不能写/*,否则出大事 -->
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- 避免静态资源,例如图片、js、css等等不要进入springMVC的处理器,否则会被当成映射来看待 -->
<servlet-mapping>
<servlet-name>default</servlet-name>
<url-pattern>*.js</url-pattern>
<url-pattern>*.css</url-pattern>
<url-pattern>*.html</url-pattern>
<url-pattern>*.htm</url-pattern>
<url-pattern>/images/*</url-pattern>
</servlet-mapping>
</web-app>
创建下面这些pk包,后面要装接口和类的。
新建jdbc.properties,这个文件是创建数据连接。实际开发一般也会这样创建,维护容易而且修改数据库时不需要修改源代码。第四行我的密码是空的是因为我的MySQL本来就是无密码,大家的有密码的就可以给它填上。
源码:
jdbc.url=jdbc:mysql://localhost:3306/gululu?useUnicode=true&characterEncoding=utf-8
jdbc.driver=com.mysql.jdbc.Driver
jdbc.username=root
jdbc.password=
新建applicationContext.xml文件,这个文件是读取文件等等的左右
源码:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.springframework.org/schema/beans" xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:cache="http://www.springframework.org/schema/cache" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/cache
http://www.springframework.org/schema/cache/spring-cache-4.0.xsd">
<context:component-scan base-package="com.bdqn.it.dao" />
<context:component-scan base-package="com.bdqn.it.service" />
<context:component-scan base-package="com.bdqn.it.util" />
<bean id="propertyConfigurer"
class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
<property name="location" value="classpath:jdbc.properties" />
</bean>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<!-- 基本信息 -->
<property name="jdbcUrl" value="${jdbc.url}"></property>
<property name="driverClass" value="${jdbc.driver}"></property>
<property name="user" value="${jdbc.username}"></property>
<property name="password" value="${jdbc.password}"></property>
</bean>
<bean id="sessionFactory"
class="org.springframework.orm.hibernate4.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource"></property>
<!--hibernate的相关选项在以下这里配置 -->
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect</prop>
<prop key="hibernate.show_sql">true</prop>
<prop key="hibernate.format_sql">true</prop>
<prop key="current_session_context_class">
org.springframework.orm.hibernate4.SpringSessionContext
</prop>
</props>
</property>
<!--映射hbm文件,只需提供hbm文件的目录位置,不再需要单独一个个挂钩 -->
<property name="mappingDirectoryLocations">
<list>
<value>classpath:com/bdqn/it/entity</value>
</list>
</property>
</bean>
<!--TransactionManager的替代品 -->
<bean id="txManager"
class="org.springframework.orm.hibernate4.HibernateTransactionManager"
p:sessionFactory-ref="sessionFactory" />
<!-- TransactionAdvice代替品 -->
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="login*" propagation="REQUIRED" />
<tx:method name="find*" read-only="true" propagation="REQUIRED" />
<tx:method name="add*" propagation="REQUIRED" />
<tx:method name="edit*" propagation="REQUIRED" />
<tx:method name="delete*" propagation="REQUIRED" />
<tx:method name="remove*" />
<tx:method name="*" propagation="NEVER" />
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="pointcut-tx"
expression="execution(public * com.bdqn.it.service.impl.*Impl.*(..))" />
<aop:advisor advice-ref="txAdvice" pointcut-ref="pointcut-tx" />
</aop:config>
</beans>
新建BaseDao接口,这里写一些我们项目常用的方法接口,后面编码继承就可以用了。除此之外,用到其他方法的时候再编写。
源码:
package com.bdqn.it.dao;
import java.io.Serializable;
import java.util.List;
import org.hibernate.criterion.DetachedCriteria;
public interface BaseDao<T> {
// 新增
public void insert(T o);
// 修改
public void update(T o);
// 删除
public void delete(T o);
// Serializable是包装类的父接口,根据id查询单个对象
public T selectById(Serializable id);
// 根据id延迟加载查询
public T LoadById(Serializable id);
// 根据条件查询单个对象
public T selectOne(DetachedCriteria dc);
// 查询所有
public List<T> selectAll();
// 查询所有
public List<T> selectList(DetachedCriteria dc);
// 根据条件查询分页的
public List<T> selectList(DetachedCriteria dc, int start, int row);
// 查询总行数,分页用
public long selectCount(DetachedCriteria dc);
}
新建两个实体类,但先不写字段
新建这两个实体的接口,而且还要继承上面BaseDao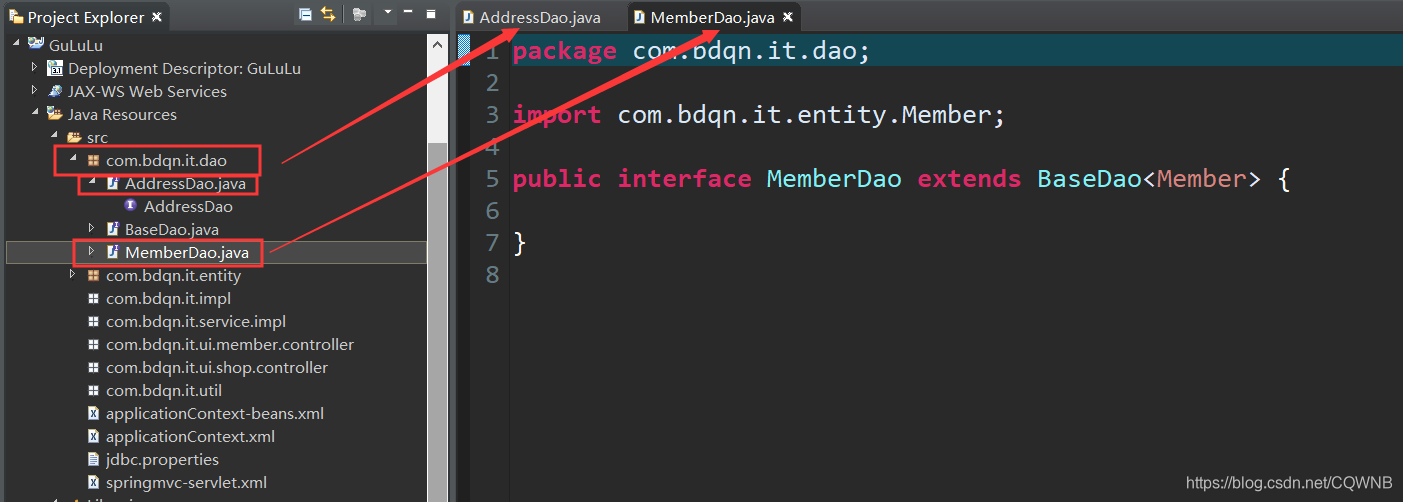
新建BaseDaoImpl泛型类,然后编写方法代码。
源码:
package com.bdqn.it.dao.impl;
import java.io.Serializable;
import java.util.List;
import org.hibernate.Criteria;
import org.hibernate.SessionFactory;
import org.hibernate.criterion.DetachedCriteria;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import com.bdqn.it.dao.BaseDao;
import java.lang.reflect.*;
public abstract class BaseDaoImpl<T> implements BaseDao<T> {
Class<T> tClass =
(Class<T>)((ParameterizedType)getClass().getGenericSuperclass()).getActualTypeArguments()[0];
@Autowired
@Qualifier("sessionFactory")
private SessionFactory fty;
@Override
public void insert(T o) {
fty.getCurrentSession().save(o);
}
@Override
public void update(T o) {
fty.getCurrentSession().update(o);
}
@Override
public void delete(T o) {
fty.getCurrentSession().delete(o);
}
@Override
public T selectById(Serializable id) {
return (T) fty.getCurrentSession().get(tClass, id);
}
@Override
public T LoadById(Serializable id) {
// 填充外键对象的时候很好用
return (T) fty.getCurrentSession().load(tClass, id);
}
@Override
public T selectOne(DetachedCriteria dc) {
Criteria c = dc.getExecutableCriteria(fty.getCurrentSession());
return (T) c.uniqueResult();
}
@Override
public List<T> selectAll() {
Criteria c = fty.getCurrentSession().createCriteria(tClass);
return c.list();
}
@Override
public List<T> selectList(DetachedCriteria dc) {
Criteria c = dc.getExecutableCriteria(fty.getCurrentSession());
return c.list();
}
@Override
public List<T> selectList(DetachedCriteria dc, int start, int row) {
Criteria c = dc.getExecutableCriteria(fty.getCurrentSession());
c.setFirstResult(start);
c.setMaxResults(row);
return c.list();
}
@Override
public long selectCount(DetachedCriteria dc) {
Criteria c = dc.getExecutableCriteria(fty.getCurrentSession());
return (long) c.uniqueResult();
}
}