其它的动画了解
1. 为 ViewGroup 对象的布局更改添加动画效果
名称 | 说明 |
---|---|
APPEARING | 当一个View在ViewGroup中出现时,对此View设置的动画 |
CHANGE_APPEARING | 当一个View在ViewGroup中出现时,对此View对其他View位置造成影响,对其他View设置的动画。 |
DISAPPEARING | 当一个View在ViewGroup中消失时,对此View设置的动画。 |
CHANGE_DISAPPEARING | 当一个View在ViewGroup中消失时,对此View对其他View位置造成影响,对其他View设置的动画。 |
LayoutTransition setLayoutTransition
举个栗子
LayoutTransition transition = new LayoutTransition();
transition.setDuration(1000);
transition.setAnimator(LayoutTransition.APPEARING,
transition.getAnimator(LayoutTransition.APPEARING)); //
transition.setAnimator(LayoutTransition.CHANGE_APPEARING,
transition.getAnimator(LayoutTransition.CHANGE_APPEARING));
transition.setAnimator(LayoutTransition.DISAPPEARING,
transition.getAnimator(LayoutTransition.DISAPPEARING));
transition.setAnimator(LayoutTransition.CHANGE_DISAPPEARING,
transition.getAnimator(LayoutTransition.CHANGE_DISAPPEARING));
viewGroup.setLayoutTransition(transition); // 设置 Layout Transition
2. 使用 StateListAnimator 为视图状态更改添加动画效果
AnimatorInflater.loadStateListAnimator
# xml 中设置 stateListAnimator
<Button
android:id="@+id/add_btn"
android:stateListAnimator="@xml/animate_scale"
# 代码中设置
button.setStateListAnimator(AnimatorInflater.loadStateListAnimator(this, R.xml.animate_scale));
# animate_scale.xml
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- the pressed state; increase x and y size to 150% -->
<item android:state_focused="true">
<set>
<objectAnimator android:propertyName="scaleX"
android:duration="@android:integer/config_shortAnimTime"
android:valueTo="1.5"
android:valueType="floatType"/>
<objectAnimator android:propertyName="scaleY"
android:duration="@android:integer/config_shortAnimTime"
android:valueTo="1.5"
android:valueType="floatType"/>
</set>
</item>
<!-- the default, non-pressed state; set x and y size to 100% -->
<item android:state_focused="false">
<set>
<objectAnimator android:propertyName="scaleX"
android:duration="@android:integer/config_shortAnimTime"
android:valueTo="1"
android:valueType="floatType"/>
<objectAnimator android:propertyName="scaleY"
android:duration="@android:integer/config_shortAnimTime"
android:valueTo="1"
android:valueType="floatType"/>
</set>
</item>
</selector>
3. Fling 动画
// 导入方式
dependencies {
implementation 'com.android.support:support-dynamic-animation:28.0.0'
}
// 代码方式
FlingAnimation fling = new FlingAnimation(view, DynamicAnimation.SCROLL_X);
设置速度,摩擦力,动画值的范围
// 以下示例展示了水平投掷。速度跟踪器捕获的速度为 velocityX,且滚动边界值设置为 0 和 maxScroll。摩擦力设置为 1.1。
fling.setStartVelocity(-velocityX)
.setMinValue(0)
.setMaxValue(maxScroll)
.setFriction(1.1f)
.start();
setStartVelocity(float)
:设置起始加速度,单位是改变的属性每秒,默认是0
setMinValue(float)
:设置动画的最小值。这个值是创建FlingAnimation中的属性值的最小值,也就是说属性值不过小于该值。
setMaxValue(float)
:与上面类似,只不过是最大值,min<=属性值<=max。
setFriction(float)
:设置摩擦力,学过力学的都知道,没有摩擦力,那么将一直运动下去;而摩擦力越大,那么将会越快停止,默认值是1。
参考资料:
https://developer.android.com/guide/topics/graphics/fling-animation
4. spring physics 动画
导入方式
dependencies {
def dynamicanimation_version = "1.0.0"
implementation 'androidx.dynamicanimation:dynamicanimation:$dynamicanimation_version'
}
小栗子
final View img = findViewById(R.id.imageView);
// Setting up a spring animation to animate the view’s translationY property with the final
// spring position at 0.
final SpringAnimation springAnim = new SpringAnimation(img, DynamicAnimation.TRANSLATION_Y, 0);
TRANSLATION_X
表示左侧坐标。
TRANSLATION_Y
表示顶部坐标。
TRANSLATION_Z
表示视图相对于其高度的深度。
ROTATION、ROTATION_X 和 ROTATION_Y
:这些属性用于控制视图围绕轴心点进行的 2D(rotation属性)和 3D 旋转。
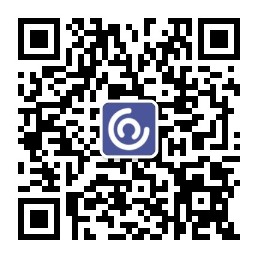
SCROLL_X 和 SCROLL_Y
:这些属性分别表示视图距离源左侧和顶部边缘的滚动偏移量(以像素为单位)。它还以页面滚动的距离来表示位置。
ALPHA
:表示视图的 Alpha 透明度。该值默认为 1(不透明),值为 0 则表示完全透明(不可见)。
参考资料:
https://developer.android.com/guide/topics/graphics/spring-animation
5. 开源动画兼容库 NineOldAndroids
Api level 11(Android 3.0才开始) 推出 的 Property Animation API,但是在 3.0以下的版本无法使用。
为了解决3.0以下的问题,大神 JakeWharton 编写了 NineOldAndroids 这个库,任意的Android版本上都可以使用 API 了!!!
这个库中会根据我们运行的机器判断其SDK版本,如果是API3.0以上则使用Android自带的动画类,否则就使用Nine Old Androids库中,这是一个兼容库。
6. SVG 动画
实现复杂动画的一种方式,SVG(Scalable vector Graphics,可缩放矢量图形),Android 5.0 开始支持 SVG图形;5.0以下的机型,添加 appcompat-v7:23.4.0
支持兼容。
一个根据 状态切换 的简单的DEMO —— SVG动画
// strikethru_on.xml => 通过SVG图片转换成 VectorDrawable
<vector xmlns:android="http://schemas.android.com/apk/res/android"
android:width="64dp"
android:height="64dp"
android:viewportWidth="24.0"
android:viewportHeight="24.0">
// 眼睛图案
<path
android:fillColor="#FFFFFF"
android:pathData="M12,4.5C7,4.5 2.73,7.61 1,12c1.73,4.39 6,7.5 11,7.5s9.27,-3.11 11,-7.5c-1.73,-4.39 -6,-7.5 -11,-7.5zM12,17c-2.76,0 -5,-2.24 -5,-5s2.24,-5 5,-5 5,2.24 5,5 -2.24,5 -5,5zM12,9c-1.66,0 -3,1.34 -3,3s1.34,3 3,3 3,-1.34 3,-3 -1.34,-3 -3,-3z" />
// 斜线的终点
<path
android:name="strike"
android:fillColor="#FFFFFF"
android:pathData="M4,4L20,20L19,21L3,5Z" />
</vector>
反之 strikethru_off.xml 的预览图效果(唯一不同是 strike 的 pathData为 “M4,4L20,20L19,21L3,5Z” 一条斜线)
根据焦点状态切换不同的 SVG动画
// strikethru_selector.xml
<?xml version="1.0" encoding="utf-8"?>
<animated-selector xmlns:android="http://schemas.android.com/apk/res/android">
// 上焦点的时候为 strikethru_on
<item
android:id="@+id/checked"
android:drawable="@drawable/strikethru_on"
android:state_focused="true" />
// 失去焦点的时候为 strikethru_off
<item
android:id="@+id/unchecked"
android:drawable="@drawable/strikethru_off" />
// unchecked -> 过渡(strikethru_off_to_on) -> checked(最终状态)
<transition
android:fromId="@id/unchecked"
android:toId="@id/checked"
android:drawable="@drawable/strikethru_off_to_on" />
// checked -> 过渡(strikethru_on_to_off) -> unchecked(最终状态)
<transition
android:fromId="@id/checked"
android:toId="@id/unchecked"
android:drawable="@drawable/strikethru_on_to_off" />
</animated-selector>
这里拿出上焦后的所有SVG动画来了解整个思路
从 strikethru_off 到 strikethru_on 的效果,中间穿插了过渡的动画效果.
strikethru_off_to_on.xml 将 需要做动画效果的 strike 的这条直线 与 动画 strike_toggle_on 关联起来.
strike 是绘制一条斜线
// strikethru_off_to_on.xml
<?xml version="1.0" encoding="utf-8"?>
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@drawable/strikethru_off">
<!-- Vector图形(strike)与动画关联(strike_toggle_on) -->
<target
android:name="strike"
android:animation="@animator/strike_toggle_on" />
</animated-vector>
// animated-vector ,把 VectorDrawable 和 objectAnimator 连起来
// strike_toggle_on.xml pathData表示的是 vector,valueFrom的矢量 到 valueTo的矢量 的动画
<objectAnimator xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="@android:integer/config_shortAnimTime"
android:interpolator="@android:interpolator/accelerate_decelerate"
android:propertyName="pathData"
android:valueFrom="M4,4L20,20L19,21L3,5Z"
android:valueTo="M4,4L4,4L3,5L3,5Z"
android:valueType="pathType" />
最后 SVG + 动画 就完成了,就像我们开头看到的效果一样
实现一个复杂的SVG动画效果
代码太多,我就不贴代码,感兴趣的可以自己去看看!!!
SVG生成的连接
SVG生成的drawable文件
手把手教学, android 使用 SVG
性能上的比较
Android Vector svg相关资料
https://www.youtube.com/watch?v=fgbl34me3kk
http://blog.chengyunfeng.com/?p=1028 SVG复杂动画教程
https://zhuanlan.zhihu.com/p/61678845
https://zhuanlan.zhihu.com/p/22013005
一些SVG相关工具
http://inloop.github.io/svg2android/ SVG转换工具
https://github.com/sketch-hq/svgo-compressor
https://romannurik.github.io/AndroidIconAnimator/ SVG动画生成工具
7. Lottie
动效 AE软件
使用 bodymovin插件
导出 json数据文件
(data.json),最后在Android 中使用. (Lottie做动画,专业的东西交给专业人做,真正解放程序员的生产力。)
导入方式
dependencies {
...
implementation "com.airbnb.android:lottie:3.4.0"
...
}
使用方式
<com.airbnb.lottie.LottieAnimationView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:lottie_fileName="data.json"
app:lottie_loop="true"
app:lottie_autoPlay="true" />
// 或者代码中导入
animationView.setAnimation("data.json");
看看几个官方的效果
使用场景
- 启动(splash)动画:典型场景是APP logo动画的播放
- 上下拉刷新动画:所有APP都必备的功能,利用 Lottie 可以做的更加简单酷炫了
- 加载(loading)动画:典型场景是网络请求的loading动画
- 提示(tips)动画:典型场景是空白页的提示
- 按钮(button)动画:典型场景如switch按钮、编辑按钮、播放按钮等按钮的切换过渡动画
- 礼物(gift)动画:典型场景是直播类APP的高级动画播放
- 视图转场动画(通过 LOTAnimationTransitionController 来实现 presentViewController 和 dismissViewControllerAnimated 转场动画)
感兴趣的可以查查资料
Android动画了解—RecyclerView Animator<=上个章节 下个章节=> Android动画了解—扩展知识