Spring 框架学习(九)---- 整合 Mybatis 框架
一、整合 Mybatis的过程
不管在整合前还是整合后,sqlSessionFactory都是非常重要的(mybatis的核心)
整合前
mybatis-config.xml配置文件 -> sqlSessionFactory ->sqlSession
整合后
sqlSessionFactory(通过注入内部包含了各种核心配置) ->sqlSessionTemplate
sqlSessionFactory 是通过构造器注入的方式注入到SqlSession中的,因为源码中没有set方法
我们在实现类中构造sqlSession的时候,需要使用构造器的方式注入该属性。
接口与映射的xml文件时通过SqlSessionFactory进行绑定的
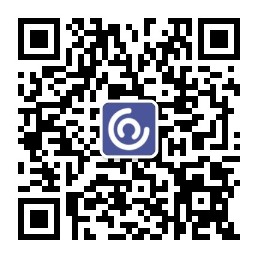
二、Spring 整合 Mybatis导入的依赖
整合前把spring-webmvc spring的一套全部导入,防止之后还有导入其他的spring依赖,下面是还需要导入的
注意mybatis 的版本和 spring的版本得匹配
(1)Mybatis 依赖(未整合spring)
<!-- https://mvnrepository.com/artifact/org.mybatis/mybatis -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
(2)mybatis-spring 整合相关的jar包
<!-- https://mvnrepository.com/artifact/org.mybatis/mybatis-spring -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.7</version>
</dependency>
(3)spring相关依赖
spring-jdbc提供驱动以及数据源
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
spring环境
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.18</version>
</dependency>
spring 的事务相关的依赖
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
(4)MySQL数据库的驱动jar包
<!-- https://mvnrepository.com/artifact/mysql/mysql-connector-java -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.16</version>
</dependency>
(5)mybatis数据源的内容
xml格式
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://127.0.0.1:3306/mybatis?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
properties格式
driver=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://127.0.0.1:3306/mybatis?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT
username=root
password=123456
三、Spring 整合 Mybatis 方式一
(1)注册数据源(使用DriverManageDataSource),使用SqlSessionFactory完全替代mybatis-config 核心配置文件,注册sqlSessionTemplate将SqlSessionFactory通过构造器注入。
-
通过数据源使得spring、mybatis连接数据库
-
SqlSessionFactory,使用SqlSessionFactoryBean类 作为mybatis的替代,所有的mybatis核心配置都注入到其中
-
SqlSessionTemplate ,就是之前我们使用的SqlSession,程序员或者用户在调用sql代码时得使用sqlSession
-
SqlSessionFactory只能通过构造器注入 注入到 SqlSessionTemplate,底层没有set方法,只有构造方法。
<!-- 数据源一般是固定代码-->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://127.0.0.1:3306/mybatis?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<!-- 完全替代了配置文件-->
<property name="dataSource" ref="dataSource" />
<property name="mapperLocations" value="classpath:com/bit/mapper/UserMapper.xml"/>
</bean>
<bean id="sqlSession" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg ref="sqlSessionFactory"/>
</bean>
(2)Mappepr层接口定义方法
package com.bit.mapper;
import com.bit.pojo.User;
import java.util.List;
public interface UserMapper {
List<User> selectAll();
}
(3)与接口绑定的xml配置文件,实现具体的方法
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.bit.mapper.UserMapper">
<select id="selectAll" resultType="com.bit.pojo.User">
select * from user;
</select>
</mapper>
(4)自定义一个类实现接口,注入了SqlSessionTemplate,在类中将方法使用SqlSessionTemplate进行调用,用户在使用的时候就不需要创建SqlSessionTemplate了
package com.bit.mapper;
import com.bit.pojo.User;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class UserMapperImpl implements UserMapper{
@Autowired
private SqlSessionTemplate sqlSessionTemplate;
// 通过 @Autowired进行注入,反射原理,set方法不需要写
// 根据类型能在配置文件中查找到SqlSessionTemplate,然后在配置文件中注入SqlSessionFactory
public List<User> selectAll() {
UserMapper userMapper = sqlSessionTemplate.getMapper(UserMapper.class);
return userMapper.selectAll();
}
// 相当于对方法封装了一层,用户在使用的时候可以直接调用,不需要再创建SqlSession
}
四、Spring 整合 Mybatis 方式二
第二种整合方式,和第一种没有本质区别
实现类继承sqlSessionDapSupport,相当于这个类对sqlSessionTemplate 又封装了一次,我们不需要注入SqlSessionTemplate了,可以继承之后直接调用父类的getSession方法拿到SqlSessionTemplate
同时继承的sqlSessionDaoSupport 得在配置文件中将 SqlSessionFactory 装配作为属性,之后的效果就和sqlSession差不多
要给实现类的父类注入sqlSessionFactort的属性,之后实现类就能达到调用自身方法就可拿到SqlSessiontemplate,不需要SqlSessionTemplate的注入装配了
(1)给实现接口的类的父类DaoSupport进行注入属性,注入SqlSessionFactory
<bean id="userMapperImpl" class="com.bit.mapper.UserMapperImpl" >
<!-- 实现类的父类daoSupport需要注入sqlSessionFactory,然后daoSupport的作用就和SqlSession差不多,不需要sqlSession注入以及装配了-->
<property name="sqlSessionFactory" ref="sqlSessionFactory"/>
</bean>
(2)实现接口的自定义类继承SqlSessionDaoSupport类,之后调用getSession方法获取SqlSession,通过sqlSession调用方法返回
package com.bit.mapper;
import com.bit.pojo.User;
import org.mybatis.spring.support.SqlSessionDaoSupport;
import java.util.List;
public class UserMapperImpl extends SqlSessionDaoSupport implements UserMapper{
public List<User> selectAll() {
return getSqlSession().getMapper(UserMapper.class).selectAll();
}
}
五、总结
最后其实本质都是一样的,第一种更接近整合的原本流程,我们要好好记住
1、注册数据源(datasource)
2、注册sqlSessionFactory,作为myabatis-config.xml的替代,在里面注入内容(数据源、注册mapper、别名等)
3、注册sqlSessionTemplate,将sqlSessionFactory通过构造器注入
4、自定义类实现接口,将SqlSessionTemplate注入进行调用方法
此时已经整合成功了
5、程序员在使用的时候,为了不用每次都创建sqlSession,在接口之外创建接口的实现类,注册实现类,在实现类中调用注入sqlSessionTemplate,然后通过sqlSession调用接口的方法
整合之后的效果
-
使用spring的配置文件彻底代替了mybatis的配置文件,
-
不需要每次在测试的时候都使用工具类进行创建sqlSession
-
spring整合 mybatis之后,事务默认是自动提交的,不需要openSession(ture)手动开启事务。