using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ALI.Utility
{
public static class Log
{
private static object lockerForError = new object(); //错误日志锁
/// <summary>
/// 保存错误日志
/// </summary>
/// <param name="source"></param>
/// <param name="type"></param>
/// <param name="error"></param>
public static void SaveLog(string source, string type, string error)
{
try
{
lock (lockerForError)
{
string strCurPath = AppDomain.CurrentDomain.BaseDirectory.ToString();
string strErrorLogPath = Path.Combine(strCurPath, "ErrorLog");
string strErrorLogFile = Path.Combine(strErrorLogPath, DateTime.Now.ToString("yyyyMMdd") + ".log");
if (!Directory.Exists(strErrorLogPath))
{
Directory.CreateDirectory(strErrorLogPath);
}
FileStream fs = new FileStream(strErrorLogFile, FileMode.OpenOrCreate);
StreamWriter streamWriter = new StreamWriter(fs);
streamWriter.BaseStream.Seek(0, SeekOrigin.End);
streamWriter.WriteLine("[" + DateTime.Now.ToString() + "]/r/nSource : " + source + "/r/nError : [" + type + "]" + error + "/r/n");
streamWriter.Flush();
streamWriter.Close();
fs.Close();
}
}
catch (Exception exception)
{
}
}
}
}
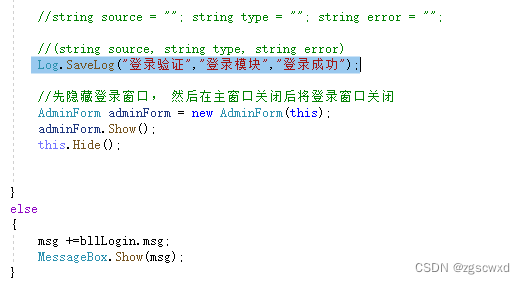
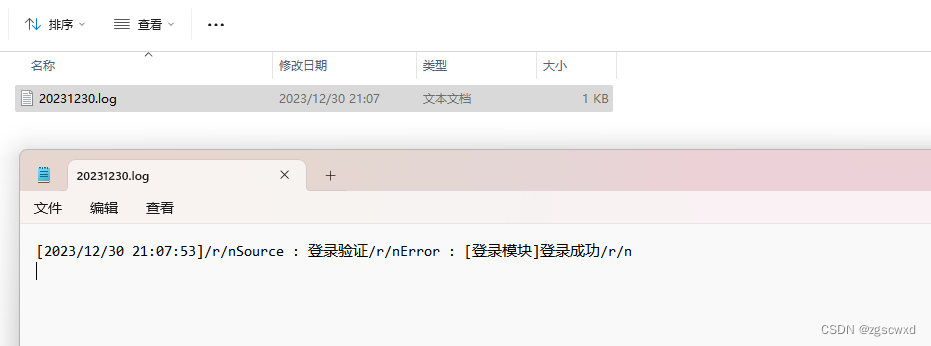
/// <summary>
/// 在log文件中写入错误日志
/// </summary>
/// <param name="path">log文件的路径</param>
/// <param name="msg">写入的错误信息</param>
public static void WriteErrorLog(string path, string msg)
{
try
{
lock (lockerForError)
{
string strCurPath = AppDomain.CurrentDomain.BaseDirectory.ToString();
string strErrorLogPath = Path.Combine(strCurPath, "loginLog");
string strErrorLogFile = Path.Combine(strErrorLogPath, DateTime.Now.ToString("yyyyMMdd") + ".log");
if (!Directory.Exists(strErrorLogPath))
{
Directory.CreateDirectory(strErrorLogPath);
}
// FileStream fs = new FileStream(strErrorLogFile, FileMode.OpenOrCreate);
FileStream fs = new FileStream(strErrorLogFile, FileMode.Append);
StreamWriter sw = new StreamWriter(fs);
string errorInfo = string.Format("时间:{0},错误信息:{1}", DateTime.Now.ToString(), msg);
sw.WriteLine(errorInfo);
sw.Close();
fs.Close();
}
}
catch (Exception exception)
{
}
}
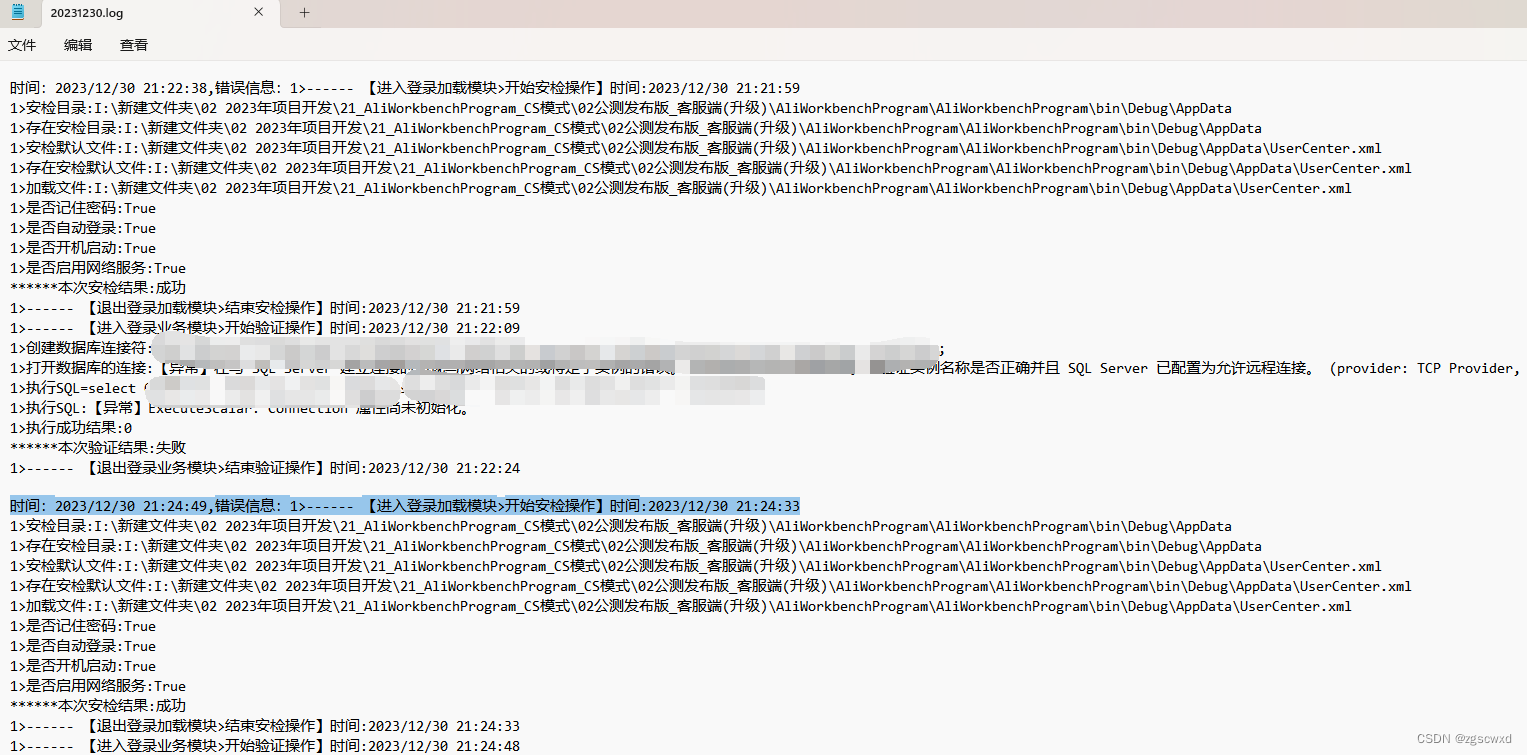
/// <summary>
/// 保存txt文档
/// </summary>
/// <param name="zhi">保存内容</param>
/// <param name="tongdao">通道</param>
public static void Txt(string zhi, string tongdao)
{
string strCurPath = AppDomain.CurrentDomain.BaseDirectory.ToString();
string strErrorLogPath = Path.Combine(strCurPath, @"Log3\\");
string path = strErrorLogPath;// "D:\\log\\";//日志文件路径&配置到Config文件中直接获取
string filename = DateTime.Now.ToString("yyyy-MM-dd") + ".txt";//文件名
string year = DateTime.Now.ToString("yyyy-MM");//年月日文件夹
string passageway = tongdao;//通道文件夹
//判断log文件路径是否存在,不存在则创建文件夹
if (!System.IO.Directory.Exists(path))
{
System.IO.Directory.CreateDirectory(path);//不存在就创建目录
}
path += passageway + "\\";
//判断通道文件夹是否存在,不存在则创建文件夹
if (!System.IO.Directory.Exists(path))
{
System.IO.Directory.CreateDirectory(path);//不存在就创建目录
}
path += year + "\\";
//判断年月文件夹是否存在,不存在则创建文件夹
if (!System.IO.Directory.Exists(path))
{
System.IO.Directory.CreateDirectory(path);//不存在就创建目录
}
//拼接完整文件路径
path += filename;
if (!File.Exists(path))
{
//文件不存在,新建文件
FileStream fs = new FileStream(path, FileMode.OpenOrCreate);
StreamWriter sw = new StreamWriter(fs);
sw.Close();
}
using (FileStream fs = new FileStream(path, FileMode.OpenOrCreate, FileAccess.Write))
{
using (StreamWriter sw = new StreamWriter(fs))
{
sw.BaseStream.Seek(0, SeekOrigin.End);
sw.Write("操作时间:" + DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss") + " 内容:{0}\n", zhi, DateTime.Now);
sw.Flush();
}
}
}