1.控制的创建继承CI_Controller系统类
application/controllers/Blog.php(一定要大写类继承的时候也要大写)
访问example.com/index.php/blog/index/abc/123输出 abc123代码如下
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Blog extends CI_Controller {
public function index($a,$b)
{
echo $a.$b;
}
}
2.设置默认访问文件 application/config/routes.php
3.控制器中执行析构函数时一定要覆盖父级析构使用parent::__construct();
public function __construct(){
parent::__construct();
// you code.....
}
4.非常重点,不允许类的名称和方法重名,否则将会被当成析构函数执行比如说Index中的index方法,非要使用的话,创建一个析构函数(******)
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Index extends CI_Controller {
public function __construct(){
parent::__construct();
echo 333;
}
public function index()
{
echo 222;
}
}
5.在控制器中抛出视图功能$this->load->view('yname');类似于tp的view(); 如模板:application/views/yname.php
public function index(){
$data['page_title'] = 'Your title';
$this->load->view('header');
$this->load->view('menu');
$this->load->view('content', $data);
$this->load->view('footer');
}
很有特点,公共部分可以直接在控制器中加载完成,而不需要去模板中调用,函数也可带参数输出到视图中
6.视图中循环遍历的写法注意起始位置为:号
//后端
public function index(){
$data['arr'] = ['1','2','3'];
$this->load->view('blog_index',$data);
}
//前台
<?php foreach ($arr as $item):?>
<li><?php echo $item;?></li>
<?php endforeach;?>
输出:1 2 3
<?php if($arr[0] == '1'):?>
XXXXXXXXXX
<?php endif; ?>
输出:xxxxxxxxx
7.模型继承系统CI_Model
//model类
<?php
class User extends CI_Model {
public function __construct()
{
parent::__construct();
}
public function get_last_ten_entries(){
}
}
//控制器中
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Blog extends CI_Controller {
public function index(){
$this->load->model('user');
$data['query'] = $this->user_model->get_last_ten_entries();
$this->load->view('blog', $data);
}
}
8.外部类存放地址 application/libraries
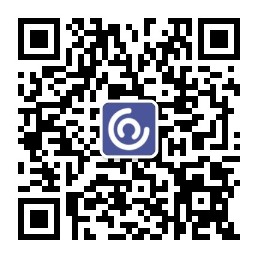
$params = array('type' => 'large', 'color' => 'red');
$this->load->library('someclass', $params);
9.路由设置application/config/routes.php
$route['blog/(:num)'] = 'blog/product_lookup_by_id/$1';
当你访问blog/3时间系统实际访问blog/product_lookuo_id/3
典型正则路由
$route['products/([a-z]+)/(\d+)'] = '$1/id_$2';
products/shirts/123会访问products/shirts/id_123
10:例如,当一个用户访问你的 Web 应用中的某个受密码保护的页面时,如果他没有 登陆,会先跳转到登陆页面,你希望在他们在成功登陆后重定向回刚才那个页面, 那么这个例子会很有用:
$route['login/(.+)'] = 'auth/login/$1';
11:开发完成后在入口文件修改development(开发版本)为production(线上版本)
define('ENVIRONMENT', isset($_SERVER['CI_ENV']) ? $_SERVER['CI_ENV'] : 'development');
CI公共函数
创建 common_helper.php 文件,定义所需公共函数,存放至 application/helpers 目录中。
在 application/config/autoload.php 中配置 $autoload['helper'] = array('common'); 即可。
-------------------------------------------
视图补充
1.分配数据到模板中
$this->load->vars($array);
--------------------------------------------
2.超级对象
当前控制器对象,提供了很多属性
a.装载器对象$this->load;(system/core.loader.php)
当调用$this->load系统会调用$sys = new CI_Loader();$this->load = $sys;
这个类在$this->load->view(),
vars(),
database(),
...
----------------------------------------------
CI_URI($this->uri);(system/core.loader.php),获取参数是方法
提供的方法
$this->uri->segment(num); //获取链接中某一段的值可以让链接急简化
如:http://127.2.2.2/index.php/admin/index/index/5
echo $this->uri->segment(4); //输出5
也可直接在方法中传值
public function index($a)
{
echo $a; //输出5
}
----------------------------------------------
CI_INPUT($this->input);(system/core.Input.php):接受前端数据类似于tp3中的I
$this->input->post('name');
$this->input->get('name');
$this->input->server('DOCUMENT_ROOT');
----------------------------------------------
数据库的访问:
1.config/database.php中配置好数据库信息
2.$this->load->database();实例化数据库(可以在autoload.php中配置自动加载:$autoload['libraries'] = array('database', 'email', 'session');)
3.SQL语句 $res = $this->db->query('select');
4.转化语句 $res->result_array();
5.返回一条 $res->row_array() //返回一条
public function add(){
$data[0] = '111';
$data[1] = '222';
$sql = "insert into swap_user (username,password) values (?,md5(?))"; //swap_为交换前缀,编译时会自动转换为配置前缀
$res = $this->db->query($sql,$data); //参数绑定直接使用?代替
if($res){
echo $this->db->affected_rows(); //受影响的行数
echo $this->db->insert_id(); //返回的ID
}
}
----------------------------------------------
数据库AR操作:
查:
$this->db->get(tablename)->result_array(); //返回一个资源句柄,查询所有数据类似于->select()
增:
$data = array('username'=>'sss','password'=>md5('ddd'));
$res = $this->db->insert('user',$data); //user表名,$data关联数组
echo $this->db->insert_id();
改:
$data = array(
'login_ip'=>'127.0.0.1'
);
$bool = $this->db->update('user',$data,array('id'=>29)); //1.表 2.数据 3.条件
删除:
$this->db->delete('user',array('id'=>29));
连贯操作
$res = $this->db->select('username')->from('user')->where(array('id >'=>2))->limit(2,3)->order_by('id desc')->get()->result_array(); //where那里一定有空格
p($res);
显示最后一条SQL
$this->db->last_query();
-----------------------------------------------
CI基类创建方法:
1.application/core/MY_Controller.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class MY_Controller extends CI_Controller {
public function __construct(){
parent::__construct();
}
}
class AdminBase extends MY_Controller{
public function __construct(){
parent::__construct();
echo '你的后台基类验证方法';
}
}
class HomeBase extends MY_Controller{
public function __construct(){
parent::__construct();
echo '你的前台基类验证方法';
}
}
2.application/controller/Welcome.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Welcome extends AdminBase {
public function index()
{
echo 11;
}
}
-----------------------------------------------
前端提交链接方法类似于tp3的{:u()}方法需要先$this->load->helper('url');可在自动加载处加载
site_url();
base_url(); //网站的根目录
-----------------------------------------------
CI框架去除index.php
打开apache的配置文件,conf/httpd.conf;
LoadModule rewrite_module modules/mod_rewrite.so
把该行前的#去掉。
搜索 AllowOverride None(配置文件中有多处),看注释信息,将相关.htaccess的该行信息改为:
AllowOverride All
在CI的根目录下,即在index.php,system的同级目录下,建立.htaccess,直接建立该文件名的不会成功,可以先建立记事本文件,另存为该名的文件即可。内容如下(CI手册上也有介绍):
RewriteEngine on
RewriteCond $1 !^(index\.php|images|robots\.txt)
RewriteRule ^(.*)$ /index.php/$1 [L]
如果不在根目录下:
RewriteEngine on
RewriteCond $1 !^(index\.php|images|robots\.txt)
RewriteRule ^(.*)$ /目录/index.php/$1 [L]
将CI中配置文件(application/config/config.php)中
$config['index_page'] = "index.php";
改成:
$config['index_page'] = "";
重启apache,完成。
-----------------------------------------------------------------------------------
分页:
public function add(){
$this->load->library('pagination');
$this->load->helper('url');
$config['base_url'] = site_url('admin/index/add');
$config['total_rows'] = 200;
$config['per_page'] = 1;
$config['first_link'] = '第一页';
$config['last_link'] = '末页';
$this->pagination->initialize($config);
$data['link'] = $this->pagination->create_links();
$this->load->view('welcome_message',$data);
}
--------------------------------------------------------------------------------------------
文件上传:
前端:
<form action="<?php echo site_url('admin/index/upload'); ?>" method="post" enctype="multipart/form-data">
<input type="file" name="files" />
<input type="submit" />
</form>
后台:
public function upload(){
$config['upload_path'] = './Upload/'; //上传的路径
$config['file_name'] = uniqid() . rand(1, 100000); //文件名字
$config['allowed_types'] = 'gif|jpg|png'; //允许的类型
$config['max_size'] = '1000'; //单位KB
$this->load->library('upload', $config);
$result = $this->upload->do_upload('files');
if($result){
p($this->upload->data());
}
}
uniqid() . rand(1, 100000) 生成唯一ID
-----------------------------------------------------------------------------------------
验证码:
public function vf(){
$this->load->helper('url');
$this->load->helper('captcha');
$vals = array(
'img_path' => './captcha/',
'img_url' => base_url().'captcha/'
);
$cap = create_captcha($vals);
$_SESSION['cap'] = $cap['word']; //提交时直接验证session
$this->load->view('welcome_message',array('img'=>$cap['image']));
}
-----------------------------------------------------------------------------------------
动态访问设置配置类:
$this->config->item('name');
$this->config->set_item('name','value');
-------------------------------------------------------------------------------------------